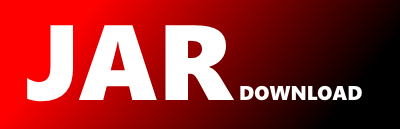
org.drmcrop.swecrop.ObjectFactory Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.02.07 at 09:47:09 AM CET
//
package org.drmcrop.swecrop;
import java.util.List;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the org.drmcrop.swecrop package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _AllowedValues_QNAME = new QName("http://www.opengis.net/swe/2.0", "AllowedValues");
private final static QName _AbstractSimpleComponent_QNAME = new QName("http://www.opengis.net/swe/2.0", "AbstractSimpleComponent");
private final static QName _AbstractEncoding_QNAME = new QName("http://www.opengis.net/swe/2.0", "AbstractEncoding");
private final static QName _AbstractDataComponent_QNAME = new QName("http://www.opengis.net/swe/2.0", "AbstractDataComponent");
private final static QName _AbstractSWE_QNAME = new QName("http://www.opengis.net/swe/2.0", "AbstractSWE");
private final static QName _DataRecord_QNAME = new QName("http://www.opengis.net/swe/2.0", "DataRecord");
private final static QName _QuantityRange_QNAME = new QName("http://www.opengis.net/swe/2.0", "QuantityRange");
private final static QName _AllowedTokens_QNAME = new QName("http://www.opengis.net/swe/2.0", "AllowedTokens");
private final static QName _NilValues_QNAME = new QName("http://www.opengis.net/swe/2.0", "NilValues");
private final static QName _Text_QNAME = new QName("http://www.opengis.net/swe/2.0", "Text");
private final static QName _Category_QNAME = new QName("http://www.opengis.net/swe/2.0", "Category");
private final static QName _AbstractSWEIdentifiable_QNAME = new QName("http://www.opengis.net/swe/2.0", "AbstractSWEIdentifiable");
private final static QName _DataArray_QNAME = new QName("http://www.opengis.net/swe/2.0", "DataArray");
private final static QName _Quantity_QNAME = new QName("http://www.opengis.net/swe/2.0", "Quantity");
private final static QName _Count_QNAME = new QName("http://www.opengis.net/swe/2.0", "Count");
private final static QName _AllowedValuesTypeInterval_QNAME = new QName("http://www.opengis.net/swe/2.0", "interval");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: org.drmcrop.swecrop
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link DataRecordType }
*
*/
public DataRecordType createDataRecordType() {
return new DataRecordType();
}
/**
* Create an instance of {@link DataArrayType }
*
*/
public DataArrayType createDataArrayType() {
return new DataArrayType();
}
/**
* Create an instance of {@link AbstractSWEType }
*
*/
public AbstractSWEType createAbstractSWEType() {
return new AbstractSWEType();
}
/**
* Create an instance of {@link AllowedValuesType }
*
*/
public AllowedValuesType createAllowedValuesType() {
return new AllowedValuesType();
}
/**
* Create an instance of {@link AbstractSWEIdentifiableType }
*
*/
public AbstractSWEIdentifiableType createAbstractSWEIdentifiableType() {
return new AbstractSWEIdentifiableType();
}
/**
* Create an instance of {@link CountType }
*
*/
public CountType createCountType() {
return new CountType();
}
/**
* Create an instance of {@link QuantityType }
*
*/
public QuantityType createQuantityType() {
return new QuantityType();
}
/**
* Create an instance of {@link TextType }
*
*/
public TextType createTextType() {
return new TextType();
}
/**
* Create an instance of {@link CategoryType }
*
*/
public CategoryType createCategoryType() {
return new CategoryType();
}
/**
* Create an instance of {@link NilValuesType }
*
*/
public NilValuesType createNilValuesType() {
return new NilValuesType();
}
/**
* Create an instance of {@link AllowedTokensType }
*
*/
public AllowedTokensType createAllowedTokensType() {
return new AllowedTokensType();
}
/**
* Create an instance of {@link QuantityRangeType }
*
*/
public QuantityRangeType createQuantityRangeType() {
return new QuantityRangeType();
}
/**
* Create an instance of {@link NilValuesPropertyType }
*
*/
public NilValuesPropertyType createNilValuesPropertyType() {
return new NilValuesPropertyType();
}
/**
* Create an instance of {@link CountPropertyType }
*
*/
public CountPropertyType createCountPropertyType() {
return new CountPropertyType();
}
/**
* Create an instance of {@link QualityPropertyType }
*
*/
public QualityPropertyType createQualityPropertyType() {
return new QualityPropertyType();
}
/**
* Create an instance of {@link UnitReference }
*
*/
public UnitReference createUnitReference() {
return new UnitReference();
}
/**
* Create an instance of {@link QuantityRangePropertyType }
*
*/
public QuantityRangePropertyType createQuantityRangePropertyType() {
return new QuantityRangePropertyType();
}
/**
* Create an instance of {@link AbstractDataComponentPropertyType }
*
*/
public AbstractDataComponentPropertyType createAbstractDataComponentPropertyType() {
return new AbstractDataComponentPropertyType();
}
/**
* Create an instance of {@link QuantityPropertyType }
*
*/
public QuantityPropertyType createQuantityPropertyType() {
return new QuantityPropertyType();
}
/**
* Create an instance of {@link TextEncodingType }
*
*/
public TextEncodingType createTextEncodingType() {
return new TextEncodingType();
}
/**
* Create an instance of {@link AllowedValuesPropertyType }
*
*/
public AllowedValuesPropertyType createAllowedValuesPropertyType() {
return new AllowedValuesPropertyType();
}
/**
* Create an instance of {@link AllowedTokensPropertyType }
*
*/
public AllowedTokensPropertyType createAllowedTokensPropertyType() {
return new AllowedTokensPropertyType();
}
/**
* Create an instance of {@link Reference }
*
*/
public Reference createReference() {
return new Reference();
}
/**
* Create an instance of {@link CategoryPropertyType }
*
*/
public CategoryPropertyType createCategoryPropertyType() {
return new CategoryPropertyType();
}
/**
* Create an instance of {@link TextPropertyType }
*
*/
public TextPropertyType createTextPropertyType() {
return new TextPropertyType();
}
/**
* Create an instance of {@link NilValue }
*
*/
public NilValue createNilValue() {
return new NilValue();
}
/**
* Create an instance of {@link EncodedValuesPropertyType }
*
*/
public EncodedValuesPropertyType createEncodedValuesPropertyType() {
return new EncodedValuesPropertyType();
}
/**
* Create an instance of {@link DataRecordType.Field }
*
*/
public DataRecordType.Field createDataRecordTypeField() {
return new DataRecordType.Field();
}
/**
* Create an instance of {@link DataArrayType.ElementType }
*
*/
public DataArrayType.ElementType createDataArrayTypeElementType() {
return new DataArrayType.ElementType();
}
/**
* Create an instance of {@link DataArrayType.Encoding }
*
*/
public DataArrayType.Encoding createDataArrayTypeEncoding() {
return new DataArrayType.Encoding();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AllowedValuesType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "AllowedValues", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSWE")
public JAXBElement createAllowedValues(AllowedValuesType value) {
return new JAXBElement(_AllowedValues_QNAME, AllowedValuesType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AbstractSimpleComponentType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "AbstractSimpleComponent", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractDataComponent")
public JAXBElement createAbstractSimpleComponent(AbstractSimpleComponentType value) {
return new JAXBElement(_AbstractSimpleComponent_QNAME, AbstractSimpleComponentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AbstractEncodingType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "AbstractEncoding", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSWE")
public JAXBElement createAbstractEncoding(AbstractEncodingType value) {
return new JAXBElement(_AbstractEncoding_QNAME, AbstractEncodingType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AbstractDataComponentType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "AbstractDataComponent", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSWEIdentifiable")
public JAXBElement createAbstractDataComponent(AbstractDataComponentType value) {
return new JAXBElement(_AbstractDataComponent_QNAME, AbstractDataComponentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AbstractSWEType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "AbstractSWE")
public JAXBElement createAbstractSWE(AbstractSWEType value) {
return new JAXBElement(_AbstractSWE_QNAME, AbstractSWEType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DataRecordType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "DataRecord", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractDataComponent")
public JAXBElement createDataRecord(DataRecordType value) {
return new JAXBElement(_DataRecord_QNAME, DataRecordType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link QuantityRangeType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "QuantityRange", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSimpleComponent")
public JAXBElement createQuantityRange(QuantityRangeType value) {
return new JAXBElement(_QuantityRange_QNAME, QuantityRangeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AllowedTokensType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "AllowedTokens", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSWE")
public JAXBElement createAllowedTokens(AllowedTokensType value) {
return new JAXBElement(_AllowedTokens_QNAME, AllowedTokensType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link NilValuesType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "NilValues", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSWE")
public JAXBElement createNilValues(NilValuesType value) {
return new JAXBElement(_NilValues_QNAME, NilValuesType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TextType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "Text", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSimpleComponent")
public JAXBElement createText(TextType value) {
return new JAXBElement(_Text_QNAME, TextType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link CategoryType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "Category", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSimpleComponent")
public JAXBElement createCategory(CategoryType value) {
return new JAXBElement(_Category_QNAME, CategoryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AbstractSWEIdentifiableType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "AbstractSWEIdentifiable", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSWE")
public JAXBElement createAbstractSWEIdentifiable(AbstractSWEIdentifiableType value) {
return new JAXBElement(_AbstractSWEIdentifiable_QNAME, AbstractSWEIdentifiableType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DataArrayType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "DataArray", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractDataComponent")
public JAXBElement createDataArray(DataArrayType value) {
return new JAXBElement(_DataArray_QNAME, DataArrayType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link QuantityType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "Quantity", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSimpleComponent")
public JAXBElement createQuantity(QuantityType value) {
return new JAXBElement(_Quantity_QNAME, QuantityType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link CountType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "Count", substitutionHeadNamespace = "http://www.opengis.net/swe/2.0", substitutionHeadName = "AbstractSimpleComponent")
public JAXBElement createCount(CountType value) {
return new JAXBElement(_Count_QNAME, CountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link List }{@code <}{@link Double }{@code >}{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opengis.net/swe/2.0", name = "interval", scope = AllowedValuesType.class)
public JAXBElement> createAllowedValuesTypeInterval(List value) {
return new JAXBElement>(_AllowedValuesTypeInterval_QNAME, ((Class) List.class), AllowedValuesType.class, ((List ) value));
}
}