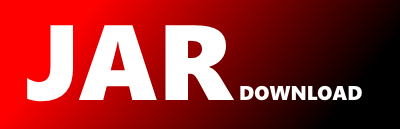
org.droidparts.test.testcase.EventBusTestCase Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2016 Alex Yanchenko
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.droidparts.test.testcase;
import org.droidparts.annotation.bus.ReceiveEvents;
import org.droidparts.bus.EventBus;
import org.droidparts.bus.EventReceiver;
import android.test.AndroidTestCase;
public class EventBusTestCase extends AndroidTestCase {
private final String NAME = "name";
private final String DATA = "data";
private int calledBackTimes = 0;
private final EventReceiver
© 2015 - 2025 Weber Informatics LLC | Privacy Policy