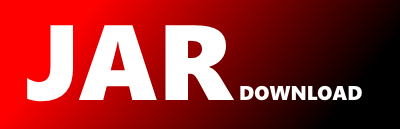
org.dromara.jpom.controller.node.NodeProjectInfoController Maven / Gradle / Ivy
/*
* The MIT License (MIT)
*
* Copyright (c) 2019 Code Technology Studio
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of
* this software and associated documentation files (the "Software"), to deal in
* the Software without restriction, including without limitation the rights to
* use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
* the Software, and to permit persons to whom the Software is furnished to do so,
* subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
* FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
* COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
* IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package org.dromara.jpom.controller.node;
import cn.hutool.core.io.FileUtil;
import cn.hutool.core.util.StrUtil;
import cn.hutool.db.Entity;
import cn.keepbx.jpom.IJsonMessage;
import cn.keepbx.jpom.model.JsonMessage;
import lombok.extern.slf4j.Slf4j;
import org.dromara.jpom.common.BaseServerController;
import org.dromara.jpom.common.ServerConst;
import org.dromara.jpom.common.ServerOpenApi;
import org.dromara.jpom.common.UrlRedirectUtil;
import org.dromara.jpom.common.validator.ValidatorItem;
import org.dromara.jpom.model.PageResultDto;
import org.dromara.jpom.model.data.NodeModel;
import org.dromara.jpom.model.node.ProjectInfoCacheModel;
import org.dromara.jpom.model.user.UserModel;
import org.dromara.jpom.permission.ClassFeature;
import org.dromara.jpom.permission.Feature;
import org.dromara.jpom.permission.MethodFeature;
import org.dromara.jpom.permission.SystemPermission;
import org.dromara.jpom.service.node.ProjectInfoCacheService;
import org.dromara.jpom.service.user.TriggerTokenLogServer;
import org.springframework.http.MediaType;
import org.springframework.util.Assert;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* 节点管理
*
* @author bwcx_jzy
* @since 2019/4/16
*/
@RestController
@RequestMapping(value = "/node")
@Feature(cls = ClassFeature.NODE)
@Slf4j
public class NodeProjectInfoController extends BaseServerController {
private final ProjectInfoCacheService projectInfoCacheService;
private final TriggerTokenLogServer triggerTokenLogServer;
public NodeProjectInfoController(ProjectInfoCacheService projectInfoCacheService,
TriggerTokenLogServer triggerTokenLogServer) {
this.projectInfoCacheService = projectInfoCacheService;
this.triggerTokenLogServer = triggerTokenLogServer;
}
/**
* @return json
* @author Hotstrip
* load node project list
* 加载节点项目列表
*/
@PostMapping(value = "node_project_list", produces = MediaType.APPLICATION_JSON_VALUE)
public IJsonMessage> nodeProjectList() {
PageResultDto resultDto = projectInfoCacheService.listPageNode(getRequest());
return JsonMessage.success("success", resultDto);
}
/**
* load node project list
* 加载节点项目列表
*
* @return json
* @author Hotstrip
*/
@PostMapping(value = "project_list", produces = MediaType.APPLICATION_JSON_VALUE)
public IJsonMessage> projectList() {
PageResultDto resultDto = projectInfoCacheService.listPage(getRequest());
return JsonMessage.success("success", resultDto);
}
/**
* load node project list
* 加载节点项目列表
*
* @return json
* @author Hotstrip
*/
@GetMapping(value = "project_list_all", produces = MediaType.APPLICATION_JSON_VALUE)
public IJsonMessage> projectListAll() {
List projectInfoCacheModels = projectInfoCacheService.listByWorkspace(getRequest());
return JsonMessage.success("", projectInfoCacheModels);
}
/**
* 查询所有的分组
*
* @return list
*/
@GetMapping(value = "list-project-group-all", produces = MediaType.APPLICATION_JSON_VALUE)
@Feature(method = MethodFeature.LIST)
public IJsonMessage> listGroupAll(HttpServletRequest request) {
List listGroup = projectInfoCacheService.listGroup(request);
return JsonMessage.success("", listGroup);
}
/**
* 同步节点项目
*
* @return json
*/
@GetMapping(value = "sync_project", produces = MediaType.APPLICATION_JSON_VALUE)
@Feature(cls = ClassFeature.PROJECT, method = MethodFeature.DEL)
public IJsonMessage
© 2015 - 2024 Weber Informatics LLC | Privacy Policy