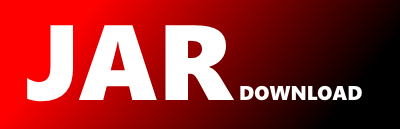
org.drools.grid.remote.command.AsyncBatchExecutionCommandImpl Maven / Gradle / Ivy
/*
* Copyright 2010 JBoss Inc
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.drools.grid.remote.command;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElements;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.drools.command.BatchExecutionCommand;
import org.drools.command.Command;
import org.drools.command.Context;
import org.drools.command.impl.GenericCommand;
import org.drools.command.runtime.process.AbortWorkItemCommand;
import org.drools.command.runtime.process.CompleteWorkItemCommand;
import org.drools.command.runtime.process.SignalEventCommand;
import org.drools.command.runtime.process.StartProcessCommand;
import org.drools.command.runtime.rule.FireAllRulesCommand;
import org.drools.command.runtime.rule.GetObjectCommand;
import org.drools.command.runtime.rule.GetObjectsCommand;
import org.drools.command.runtime.rule.InsertElementsCommand;
import org.drools.command.runtime.rule.InsertObjectCommand;
import org.drools.command.runtime.rule.ModifyCommand;
import org.drools.command.runtime.rule.RetractCommand;
import org.drools.command.runtime.rule.QueryCommand;
import org.drools.command.runtime.GetGlobalCommand;
import org.drools.command.runtime.SetGlobalCommand;
import org.drools.runtime.ExecutionResults;
/**
* Java class for BatchExecutionCommand complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="BatchExecutionCommand">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice maxOccurs="unbounded">
* <element name="abort-work-item" type="{http://drools.org/drools-5.0/knowledge-session}AbortWorkItemCommand"/>
* <element name="complete-work-item" type="{http://drools.org/drools-5.0/knowledge-session}CompleteWorkItemCommand"/>
* <element name="fire-all-rules" type="{http://drools.org/drools-5.0/knowledge-session}FireAllRulesCommand"/>
* <element name="get-global" type="{http://drools.org/drools-5.0/knowledge-session}GetGlobalCommand"/>
* <element name="insert" type="{http://drools.org/drools-5.0/knowledge-session}InsertObjectCommand"/>
* <element name="insert-elements" type="{http://drools.org/drools-5.0/knowledge-session}InsertElementsCommand"/>
* <element name="query" type="{http://drools.org/drools-5.0/knowledge-session}QueryCommand"/>
* <element name="set-global" type="{http://drools.org/drools-5.0/knowledge-session}SetGlobalCommand"/>
* <element name="signal-event" type="{http://drools.org/drools-5.0/knowledge-session}SignalEventCommand"/>
* <element name="start-process" type="{http://drools.org/drools-5.0/knowledge-session}StartProcessCommand"/>
* </choice>
* </restriction>
* </complexContent>
* </complexType>
*
*/
@XmlRootElement(name="batch-execution")
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "batch-execution", propOrder = {"lookup", "commands"})
public class AsyncBatchExecutionCommandImpl implements BatchExecutionCommand, GenericCommand {
private static final long serialVersionUID = 510l;
@XmlAttribute
private String lookup;
public AsyncBatchExecutionCommandImpl(){
}
public AsyncBatchExecutionCommandImpl( List commands ) {
List> genericCmds = new ArrayList>();
for(Command cmd : commands){
genericCmds.add((GenericCommand>)cmd);
}
this.commands = genericCmds;
}
public AsyncBatchExecutionCommandImpl( List> commands, String lookup ) {
this.commands = commands;
this.lookup = lookup;
}
@XmlElements({
@XmlElement(name = "abort-work-item", type = AbortWorkItemCommand.class),
@XmlElement(name = "signal-event", type = SignalEventCommand.class),
@XmlElement(name = "start-process", type = StartProcessCommand.class),
@XmlElement(name = "retract", type = RetractCommand.class),
@XmlElement(name = "get-global", type = GetGlobalCommand.class),
@XmlElement(name = "set-global", type = SetGlobalCommand.class),
@XmlElement(name = "insert-elements", type = InsertElementsCommand.class),
@XmlElement(name = "query", type = QueryCommand.class),
@XmlElement(name = "insert", type = InsertObjectCommand.class),
@XmlElement(name = "modify", type = ModifyCommand.class),
@XmlElement(name = "get-object", type = GetObjectCommand.class),
@XmlElement(name = "fire-all-rules", type = FireAllRulesCommand.class),
@XmlElement(name = "complete-work-item", type = CompleteWorkItemCommand.class),
@XmlElement(name = "get-objects", type = GetObjectsCommand.class)
})
protected List> commands;
/**
* Gets the value of the abortWorkItemOrCompleteWorkItemOrFireAllRules property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the abortWorkItemOrCompleteWorkItemOrFireAllRules property.
*
*
* For example, to add a new item, do as follows:
*
* getCommand().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SetGlobalCommand }
* {@link CompleteWorkItemCommand }
* {@link AbortWorkItemCommand }
* {@link SignalEventCommand }
* {@link FireAllRulesCommand }
* {@link StartProcessCommand }
* {@link GetGlobalCommand }
* {@link InsertElementsCommand }
* {@link QueryCommand }
* {@link InsertObjectCommand }
*/
public List> getCommands() {
if (commands == null) {
commands = new ArrayList>();
}
return this.commands;
}
public ExecutionResults execute(Context context) {
for ( GenericCommand> command : commands ) {
((GenericCommand>)command).execute( context );
}
return null;
}
public void setLookup(String lookup) {
this.lookup = lookup;
}
public String getLookup() {
return lookup;
}
public String toString() {
return "BatchExecutionCommandImpl{" +
"lookup='" + lookup + '\'' +
", commands=" + commands +
'}';
}
}