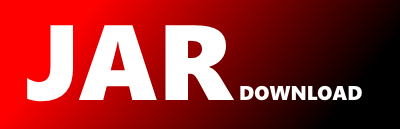
org.drools.jsr94.rules.admin.LocalRuleExecutionSetProviderImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of drools-jsr94 Show documentation
Show all versions of drools-jsr94 Show documentation
Implements the JSR-94 API with Drools.
The newest version!
/*
* Copyright 2010 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.drools.jsr94.rules.admin;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.io.StringReader;
import java.util.Map;
import javax.rules.admin.LocalRuleExecutionSetProvider;
import javax.rules.admin.RuleExecutionSet;
import javax.rules.admin.RuleExecutionSetCreateException;
import org.drools.compiler.builder.impl.KnowledgeBuilderConfigurationImpl;
import org.drools.compiler.builder.impl.KnowledgeBuilderImpl;
import org.drools.compiler.compiler.DroolsParserException;
import org.drools.core.definitions.InternalKnowledgePackage;
import org.drools.core.util.IoUtils;
import org.drools.decisiontable.InputType;
import org.drools.decisiontable.SpreadsheetCompiler;
import org.drools.jsr94.rules.Constants;
/**
* The Drools implementation of the LocalRuleExecutionSetProvider
* interface which defines RuleExecutionSet
creation methods for
* defining RuleExecutionSet
s from local (non-serializable)
* resources.
*
* @see LocalRuleExecutionSetProvider
*/
public class LocalRuleExecutionSetProviderImpl
implements
LocalRuleExecutionSetProvider {
/** Default constructor. */
public LocalRuleExecutionSetProviderImpl() {
super();
}
/**
* Creates a RuleExecutionSet
implementation using a supplied
* input stream and additional Drools-specific properties. A Drools-specific
* rule execution set is read from the supplied InputStream. The method
* createRuleExecutionSet
taking a Reader instance should be
* used if the source is a character stream and encoding conversion should
* be performed.
*
* @param ruleExecutionSetStream
* an input stream used to read the rule execution set.
* @param properties
* additional properties used to create the
* RuleExecutionSet
implementation. May be
* null
.
*
* @throws RuleExecutionSetCreateException
* on rule execution set creation error.
*
* @return The created RuleExecutionSet
.
*/
public RuleExecutionSet createRuleExecutionSet(final InputStream ruleExecutionSetStream,
final Map properties) throws RuleExecutionSetCreateException {
if ( properties != null ) {
String source = ( String ) properties.get( Constants.RES_SOURCE );
if ( source == null ) {
// support legacy name
source = ( String ) properties.get( "source" );
}
if ( source != null && source.equals( Constants.RES_SOURCE_TYPE_DECISION_TABLE ) ) {
final SpreadsheetCompiler converter = new SpreadsheetCompiler();
final String drl = converter.compile( ruleExecutionSetStream,
InputType.XLS );
return createRuleExecutionSet( new StringReader( drl ), properties );
} else {
return createRuleExecutionSet( new InputStreamReader( ruleExecutionSetStream, IoUtils.UTF8_CHARSET ), properties);
}
} else
return createRuleExecutionSet( new InputStreamReader( ruleExecutionSetStream, IoUtils.UTF8_CHARSET ), properties);
}
/**
* Creates a RuleExecutionSet
implementation using a supplied
* character stream Reader and additional Drools-specific properties. A
* Drools-specific rule execution set is read from the supplied Reader.
*
* @param ruleExecutionSetReader
* a Reader used to read the rule execution set.
* @param properties
* additional properties used to create the
* RuleExecutionSet
implementation. May be
* null
.
*
* @throws RuleExecutionSetCreateException
* on rule execution set creation error.
*
* @return The created RuleExecutionSet
.
*/
public RuleExecutionSet createRuleExecutionSet(final Reader ruleExecutionSetReader,
final Map properties) throws RuleExecutionSetCreateException {
try {
KnowledgeBuilderConfigurationImpl config= null;
if ( properties != null ) {
config = (KnowledgeBuilderConfigurationImpl) properties.get( Constants.RES_PACKAGEBUILDER_CONFIG );
}
KnowledgeBuilderImpl builder = null;
if ( config != null ) {
builder = new KnowledgeBuilderImpl(config);
} else {
builder = new KnowledgeBuilderImpl();
}
Object dsrl = null;
String source = null;
if ( properties != null ) {
dsrl = properties.get( Constants.RES_DSL );
if ( dsrl == null ) {
// check for old legacy name ending
dsrl = properties.get( "dsl" );
}
source = ( String ) properties.get( Constants.RES_SOURCE );
if ( source == null ) {
// check for old legacy name ending
source = ( String ) properties.get( "source" );
}
}
if ( source == null ) {
source = "drl";
}
if ( dsrl == null ) {
if ( source.equals( Constants.RES_SOURCE_TYPE_XML ) || source.equals( "xml" ) ) {
builder.addPackageFromXml( ruleExecutionSetReader );
} else {
builder.addPackageFromDrl( ruleExecutionSetReader );
}
} else {
if ( source.equals( Constants.RES_SOURCE_TYPE_XML ) || source.equals( "xml" ) ) {
// xml cannot specify a dsl
builder.addPackageFromXml( ruleExecutionSetReader );
} else {
if ( dsrl instanceof Reader ) {
builder.addPackageFromDrl( ruleExecutionSetReader,
(Reader) dsrl );
} else {
builder.addPackageFromDrl( ruleExecutionSetReader,
new StringReader( (String) dsrl ) );
}
}
}
InternalKnowledgePackage pkg = builder.getPackageNames().stream()
.map( builder::getPackage )
.filter( p -> !p.getRules().isEmpty() )
.findFirst().get();
return createRuleExecutionSet( pkg,
properties );
} catch ( final IOException e ) {
throw new RuleExecutionSetCreateException( "cannot create rule execution set",
e );
} catch ( final DroolsParserException e ) {
throw new RuleExecutionSetCreateException( "cannot create rule execution set",
e );
}
}
/**
* Creates a RuleExecutionSet
implementation from a
* Drools-specific AST representation and Drools-specific properties.
*
* @param ruleExecutionSetAst
* the vendor representation of a rule execution set
* @param properties
* additional properties used to create the
* RuleExecutionSet
implementation. May be
* null
.
*
* @throws RuleExecutionSetCreateException
* on rule execution set creation error.
*
* @return The created RuleExecutionSet
.
*/
public RuleExecutionSet createRuleExecutionSet(final Object ruleExecutionSetAst,
final Map properties) throws RuleExecutionSetCreateException {
if ( ruleExecutionSetAst instanceof InternalKnowledgePackage ) {
InternalKnowledgePackage pkg = (InternalKnowledgePackage) ruleExecutionSetAst;
return this.createRuleExecutionSet( pkg,
properties );
}
throw new RuleExecutionSetCreateException( " Incoming AST object must be an org.kie.rule.Package. Was " + ruleExecutionSetAst.getClass() );
}
/**
* Creates a RuleExecutionSet
implementation from a
* RuleSet
and Drools-specific properties.
*
* @param pkg
* a Drools org.kie.rule.Package
representation
* of a rule execution set.
* @param properties
* additional properties used to create the RuleExecutionSet
* implementation. May be null
.
*
* @throws RuleExecutionSetCreateException
* on rule execution set creation error.
*
* @return The created RuleExecutionSet
.
*/
private RuleExecutionSet createRuleExecutionSet(final InternalKnowledgePackage pkg,
final Map properties) throws RuleExecutionSetCreateException {
try {
return new RuleExecutionSetImpl( pkg,
properties );
} catch ( Exception e ) {
throw new RuleExecutionSetCreateException( "Failed to create RuleExecutionSet",
e );
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy