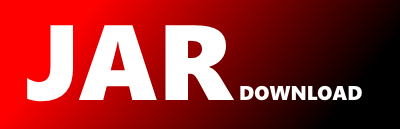
org.drools.verifier.dao.DataTree Maven / Gradle / Ivy
package org.drools.verifier.dao;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.TreeSet;
public class DataTree {
private Map> map = new TreeMap>();
public void put(K key, V value) {
if (map.containsKey(key)) {
Set set = map.get(key);
set.add(value);
} else {
Set set = new TreeSet();
set.add(value);
map.put(key, set);
}
}
public Set keySet() {
return map.keySet();
}
public Set getBranch(K key) {
Set set = map.get(key);
if (set != null) {
return set;
} else {
return Collections.emptySet();
}
}
public Collection values() {
Collection values = new ArrayList();
for (Set set : map.values()) {
for (V value : set) {
values.add(value);
}
}
return values;
}
public boolean remove(K key, V value) {
Set set = map.get(key);
if (set != null) {
return set.remove(value);
} else {
return false;
}
}
@Override
public String toString() {
return values().toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy