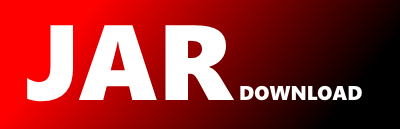
org.drools.verifier.incoherence.Patterns.drl Maven / Gradle / Ivy
#created on: 14.11.2007
package org.drools.verifier.incoherence
#list any import classes here.
import org.drools.verifier.components.VerifierRule;
import org.drools.verifier.components.LiteralRestriction;
import org.drools.verifier.components.PatternPossibility;
import org.drools.verifier.components.RulePossibility;
import org.drools.verifier.components.Pattern;
import org.drools.verifier.components.VariableRestriction;
import org.drools.verifier.report.components.Cause;
import org.drools.verifier.report.components.MissingRange;
import org.drools.verifier.report.components.VerifierMessage;
import org.drools.verifier.report.components.Severity;
import org.drools.verifier.report.components.MessageType;
import org.drools.verifier.dao.VerifierResult;
import java.util.Collection;
import java.util.ArrayList;
import org.drools.base.evaluators.Operator;
#declare any global variables here
global VerifierResult result;
#
# If two Patterns are in conflict.
#
# Type: Warning
# Dependencies: None
# Example:
# IncorencePattern( a == 1 )
# not IncorencePattern( a == 1 )
#
rule "Incoherent Patterns in rule possibility"
when
$r1 :LiteralRestriction(
patternIsNot == true
)
$r2 :LiteralRestriction(
ruleId == $r1.ruleId,
patternIsNot == false,
restrictionType == $r1.restrictionType,
fieldId == $r1.fieldId,
valueType == $r1.valueType,
operator == $r1.operator
)
eval( $r1.compareValues( $r2 ) == 0 )
$pp1 :PatternPossibility(
patternId == $r1.patternId
)
$pp2 :PatternPossibility(
patternId == $r2.patternId
)
# There is a problem if both of these are in the same RulePossibility.
$rp :RulePossibility(
items contains $pp1,
items contains $pp2
)
$p1 :Pattern(
id == $r1.patternId
)
$p2 :Pattern(
id == $r2.patternId
)
$r :VerifierRule(
id == $rp.ruleId
)
then
Collection list = new ArrayList();
list.add( $p1 );
list.add( $p2 );
result.add( new VerifierMessage(
Severity.WARNING,
MessageType.INCOHERENCE,
$r,
"Pattern " + $p1 + " and " + $p2 +
" are in conflict. Because of this, it is possible that the rule that contains them can never be satisfied.",
list
) );
end
#
# If two Patterns are in conflict.
#
# Type: Warning
# Dependencies: None
# Example:
# $var :Object()
# IncorencePattern( a == $var )
# not IncorencePattern( a == $var )
#
rule "Incoherent Patterns in rule possibility, variables"
when
$r1 :VariableRestriction(
patternIsNot == true
)
$r2 :VariableRestriction(
ruleId == $r1.ruleId,
patternIsNot == false,
fieldId == $r1.fieldId,
variable.objectId == $r1.variable.objectId,
variable.objectType == $r1.variable.objectType,
operator == $r1.operator
)
$pp1 :PatternPossibility(
patternId == $r1.patternId
)
$pp2 :PatternPossibility(
patternId == $r2.patternId
)
# There is a problem if both of these are in the same RulePossibility.
$rp :RulePossibility(
items contains $pp1,
items contains $pp2
)
$p1 :Pattern(
id == $r1.patternId
)
$p2 :Pattern(
id == $r2.patternId
)
$r :VerifierRule(
id == $rp.ruleId
)
then
Collection list = new ArrayList();
list.add( $p1 );
list.add( $p2 );
result.add( new VerifierMessage(
Severity.WARNING,
MessageType.INCOHERENCE,
$r,
"Pattern " + $p1 + " and " + $p2 +
" are in conflict. Because of this, it is possible that the rule that contains them can never be satisfied.",
list
) );
end
#
# If two Patterns are in conflict.
#
# Type: Warning
# Dependencies: None
# Example:
# IncorencePattern8( a > 11 )
# not IncorencePattern8( a > 1 )
#
rule "Incoherent Patterns in rule possibility, ranges when not conflicts with lesser value"
when
$r1 :LiteralRestriction(
patternIsNot == true,
( operator == Operator.GREATER || == Operator.GREATER_OR_EQUAL )
)
$r2 :LiteralRestriction(
ruleId == $r1.ruleId,
patternIsNot == false,
( operator == Operator.GREATER || == Operator.GREATER_OR_EQUAL || == Operator.EQUAL ),
fieldId == $r1.fieldId
)
eval( $r1.compareValues( $r2 ) == -1 )
$pp1 :PatternPossibility(
patternId == $r1.patternId
)
$pp2 :PatternPossibility(
patternId == $r2.patternId
)
# There is a problem if both of these are in the same RulePossibility.
$rp :RulePossibility(
items contains $pp1,
items contains $pp2
)
$p1 :Pattern(
id == $r1.patternId
)
$p2 :Pattern(
id == $r2.patternId
)
$r :VerifierRule(
id == $rp.ruleId
)
then
Collection list = new ArrayList();
list.add( $p1 );
list.add( $p2 );
result.add( new VerifierMessage(
Severity.WARNING,
MessageType.INCOHERENCE,
$r,
"Pattern " + $p1 + " and " + $p2 +
" are in conflict. Because of this, it is possible that the rule that contains them can never be satisfied.",
list
) );
end
#
# If two Patterns are in conflict.
#
# Type: Warning
# Dependencies: None
# Example:
# IncorencePattern( a < 1 )
# not IncorencePattern( a < 11 )
#
rule "Incoherent Patterns in rule possibility, ranges when not conflicts with greater value"
when
$r1 :LiteralRestriction(
patternIsNot == true,
( operator == Operator.LESS || == Operator.LESS_OR_EQUAL )
)
$r2 :LiteralRestriction(
ruleId == $r1.ruleId,
patternIsNot == false,
( operator == Operator.LESS || == Operator.LESS_OR_EQUAL || == Operator.EQUAL ),
fieldId == $r1.fieldId
)
eval( $r1.compareValues( $r2 ) == 1 )
$pp1 :PatternPossibility(
patternId == $r1.patternId
)
$pp2 :PatternPossibility(
patternId == $r2.patternId
)
# There is a problem if both of these are in the same RulePossibility.
$rp :RulePossibility(
items contains $pp1,
items contains $pp2
)
$p1 :Pattern(
id == $r1.patternId
)
$p2 :Pattern(
id == $r2.patternId
)
$r :VerifierRule(
id == $rp.ruleId
)
then
Collection list = new ArrayList();
list.add( $p1 );
list.add( $p2 );
result.add( new VerifierMessage(
Severity.WARNING,
MessageType.INCOHERENCE,
$r,
"Pattern " + $p1 + " and " + $p2 +
" are in conflict. Because of this, it is possible that the rule that contains them can never be satisfied.",
list
) );
end
#
# If two Patterns are in conflict.
#
# Type: Warning
# Dependencies: None
# Example:
# IncoherencePattern( a >= 1 )
# not IncoherencePattern( a != 1 )
#
rule "Incoherent Patterns in rule possibility, ranges when not conflicts with equal or unequal value"
when
$r1 :LiteralRestriction(
patternIsNot == true,
operator == Operator.NOT_EQUAL
)
$r2 :LiteralRestriction(
ruleId == $r1.ruleId,
patternIsNot == false,
# It is also a problem if the value is NOT_EQUAL, but there is already a rule for that.
( operator != Operator.EQUAL && != Operator.NOT_EQUAL ),
fieldId == $r1.fieldId
)
eval( $r1.compareValues( $r2 ) == 0 )
$pp1 :PatternPossibility(
patternId == $r1.patternId
)
$pp2 :PatternPossibility(
patternId == $r2.patternId
)
# There is a problem if both of these are in the same RulePossibility.
$rp :RulePossibility(
items contains $pp1,
items contains $pp2
)
$p1 :Pattern(
id == $r1.patternId
)
$p2 :Pattern(
id == $r2.patternId
)
$r :VerifierRule(
id == $rp.ruleId
)
then
Collection list = new ArrayList();
list.add( $p1 );
list.add( $p2 );
result.add( new VerifierMessage(
Severity.WARNING,
MessageType.INCOHERENCE,
$r,
"Pattern " + $p1 + " and " + $p2 +
" are in conflict. Because of this, it is possible that the rule that contains them can never be satisfied.",
list
) );
end
#
# If two Patterns are in conflict.
#
# Type: Warning
# Dependencies: None
# Example:
# IncoherencePattern15( a >= $var )
# not IncoherencePattern15( a != $var )
#
rule "Incoherent Patterns in rule possibility, ranges when not conflicts with equal or unequal variables"
when
$r1 :VariableRestriction(
patternIsNot == true,
operator == Operator.NOT_EQUAL
)
$r2 :VariableRestriction(
ruleId == $r1.ruleId,
patternIsNot == false,
fieldId == $r1.fieldId,
variable.objectId == $r1.variable.objectId,
variable.objectType == $r1.variable.objectType,
# It is also a problem if the value is NOT_EQUAL, but there is already a rule for that.
( operator != Operator.EQUAL && != Operator.NOT_EQUAL )
)
$pp1 :PatternPossibility(
patternId == $r1.patternId
)
$pp2 :PatternPossibility(
patternId == $r2.patternId
)
# There is a problem if both of these are in the same RulePossibility.
$rp :RulePossibility(
items contains $pp1,
items contains $pp2
)
$p1 :Pattern(
id == $r1.patternId
)
$p2 :Pattern(
id == $r2.patternId
)
$r :VerifierRule(
id == $rp.ruleId
)
then
Collection list = new ArrayList();
list.add( $p1 );
list.add( $p2 );
result.add( new VerifierMessage(
Severity.WARNING,
MessageType.INCOHERENCE,
$r,
"Pattern " + $p1 + " and " + $p2 +
" are in conflict. Because of this, it is possible that the rule that contains them can never be satisfied.",
list
) );
end
#
# If two Patterns are in conflict.
#
# Type: Warning
# Dependencies: None
# Example:
# IncoherencePattern13( a == $var )
# not IncoherencePattern13( a >= $var )
#
rule "Incoherent Patterns in rule possibility, ranges when not conflicts with equal value"
when
$r1 :LiteralRestriction(
patternIsNot == true,
( operator == Operator.LESS_OR_EQUAL || == Operator.GREATER_OR_EQUAL )
)
$r2 :LiteralRestriction(
ruleId == $r1.ruleId,
patternIsNot == false,
operator == Operator.EQUAL,
fieldId == $r1.fieldId
)
eval( $r1.compareValues( $r2 ) == 0 )
$pp1 :PatternPossibility(
patternId == $r1.patternId
)
$pp2 :PatternPossibility(
patternId == $r2.patternId
)
# There is a problem if both of these are in the same RulePossibility.
$rp :RulePossibility(
items contains $pp1,
items contains $pp2
)
$p1 :Pattern(
id == $r1.patternId
)
$p2 :Pattern(
id == $r2.patternId
)
$r :VerifierRule(
id == $rp.ruleId
)
then
Collection list = new ArrayList();
list.add( $p1 );
list.add( $p2 );
result.add( new VerifierMessage(
Severity.WARNING,
MessageType.INCOHERENCE,
$r,
"Pattern " + $p1 + " and " + $p2 +
" are in conflict. Because of this, it is possible that the rule that contains them can never be satisfied.",
list
) );
end
#
# If two Patterns are in conflict.
#
# Type: Warning
# Dependencies: None
# Example:
# IncoherencePattern13( a == $var )
# not IncoherencePattern13( a >= $var )
#
rule "Incoherent Patterns in rule possibility, ranges when not conflicts with equal variable"
when
$r1 :VariableRestriction(
patternIsNot == true,
# Equal is also a problem, but there is already a rule for that.
( operator == Operator.LESS_OR_EQUAL || == Operator.GREATER_OR_EQUAL )
)
$r2 :VariableRestriction(
ruleId == $r1.ruleId,
patternIsNot == false,
fieldId == $r1.fieldId,
variable.objectId == $r1.variable.objectId,
variable.objectType == $r1.variable.objectType,
operator == Operator.EQUAL
)
$pp1 :PatternPossibility(
patternId == $r1.patternId
)
$pp2 :PatternPossibility(
patternId == $r2.patternId
)
# There is a problem if both of these are in the same RulePossibility.
$rp :RulePossibility(
items contains $pp1,
items contains $pp2
)
$p1 :Pattern(
id == $r1.patternId
)
$p2 :Pattern(
id == $r2.patternId
)
$r :VerifierRule(
id == $rp.ruleId
)
then
Collection list = new ArrayList();
list.add( $p1 );
list.add( $p2 );
result.add( new VerifierMessage(
Severity.WARNING,
MessageType.INCOHERENCE,
$r,
"Pattern " + $p1 + " and " + $p2 +
" are in conflict. Because of this, it is possible that the rule that contains them can never be satisfied.",
list
) );
end
© 2015 - 2025 Weber Informatics LLC | Privacy Policy