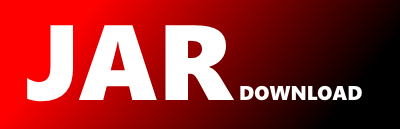
org.drools.verifier.components.VerifierRule Maven / Gradle / Ivy
/*
* Copyright 2010 JBoss Inc
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.drools.verifier.components;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.drools.compiler.lang.descr.BaseDescr;
import org.drools.verifier.components.Consequence.ConsequenceType;
import org.drools.verifier.report.components.Cause;
public class VerifierRule extends PackageComponent
implements
Cause {
private Map attributes = new HashMap();
private String consequencePath;
private ConsequenceType consequenceType;
private int lineNumber;
private int packageId;
private String name;
private Collection header = new ArrayList();
private Collection lhsRows = new ArrayList();
private Collection rhsRows = new ArrayList();
private String description;
private Map> metadata = new HashMap>();
private Collection commentMetadata = new ArrayList();
private Map> otherInfo = new HashMap>();
private int offset = 0;
public VerifierRule(BaseDescr descr,RulePackage rulePackage) {
super(descr, rulePackage );
}
@Override
public String getPath() {
return String.format( "%s/rule[@name='%s']",
getPackagePath(),
getName() );
}
public int getOffset() {
offset++;
return offset % 2;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setAttributes(Map attributes) {
this.attributes = attributes;
}
public Map getAttributes() {
return attributes;
}
public String getConsequencePath() {
return consequencePath;
}
public void setConsequencePath(String consequencePath) {
this.consequencePath = consequencePath;
}
public ConsequenceType getConsequenceType() {
return consequenceType;
}
public void setConsequenceType(ConsequenceType consequenceType) {
this.consequenceType = consequenceType;
}
public int getLineNumber() {
return lineNumber;
}
public void setLineNumber(int lineNumber) {
this.lineNumber = lineNumber;
}
@Override
public String toString() {
return "Rule '" + getName() + "'";
}
public int getPackageId() {
return packageId;
}
public void setPackageId(int packageId) {
this.packageId = packageId;
}
public VerifierComponentType getVerifierComponentType() {
return VerifierComponentType.RULE;
}
public Collection getHeader() {
return header;
}
public Collection getLhsRows() {
return lhsRows;
}
public Collection getRhsRows() {
return rhsRows;
}
public Map> getMetadata() {
return metadata;
}
public String getMetaAttribute(String key) {
Map elementValues = metadata.get(key);
return elementValues != null ? elementValues.keySet().iterator().next() : null;
}
public Collection getCommentMetadata() {
return commentMetadata;
}
public void setDescription(String description) {
this.description = description;
}
public String getDescription() {
return description;
}
public Map> getOtherInfo() {
return otherInfo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy