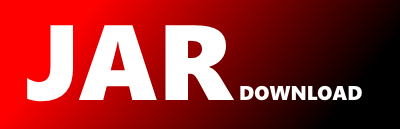
org.jbpm.simulation.impl.SimulationPath Maven / Gradle / Ivy
/*
* Copyright 2015 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jbpm.simulation.impl;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.jbpm.simulation.PathContext;
public class SimulationPath {
private String pathId;
private List sequenceFlowsIds = new ArrayList();
private List activityIds = new ArrayList();
private List boundaryEventIds = new ArrayList();
private List origPaths = new ArrayList();
private Map catchEvents = new HashMap();
private Map throwEvents = new HashMap();
private Map seqenceFlowsSources = new HashMap();
private String signalName;
private boolean startable = false;
private double probability;
public double getProbability() {
return probability;
}
public void setProbability(double probability) {
this.probability = probability;
}
public void addSequenceFlow(String id) {
this.sequenceFlowsIds.add(id);
}
public void addActivity(String id) {
this.activityIds.add(id);
}
public List getSequenceFlowsIds() {
return sequenceFlowsIds;
}
public void setSequenceFlowsIds(List sequenceFlowsIds) {
this.sequenceFlowsIds = sequenceFlowsIds;
}
public List getActivityIds() {
return activityIds;
}
public void setActivityIds(List activityIds) {
this.activityIds = activityIds;
}
public List getOrigPaths() {
return origPaths;
}
public void setOrigPaths(List origPaths) {
this.origPaths = origPaths;
}
public String getPathId() {
return pathId;
}
public void setPathId(String pathId) {
this.pathId = pathId;
}
public List getBoundaryEventIds() {
return boundaryEventIds;
}
public void setBoundaryEventIds(List boundaryEventIds) {
this.boundaryEventIds = boundaryEventIds;
}
public void addBoundaryEventId(String id) {
this.boundaryEventIds.add(id);
}
public Map getCatchEvents() {
return catchEvents;
}
public void setCatchEvents(Map catchEvents) {
this.catchEvents = catchEvents;
}
public void addCatchEvent(String ref, String activityId) {
this.catchEvents.put(ref, activityId);
}
public Map getThrowEvents() {
return throwEvents;
}
public void setThrowEvents(Map throwEvents) {
this.throwEvents = throwEvents;
}
public void addThrowEvent(String activityId, String ref) {
this.throwEvents.put(activityId, ref);
}
public String getSignalName() {
return signalName;
}
public void setSignalName(String signalName) {
this.signalName = signalName;
}
public boolean isStartable() {
return startable;
}
public void setStartable(boolean startable) {
this.startable = startable;
}
public Map getSeqenceFlowsSources() {
return seqenceFlowsSources;
}
public void setSeqenceFlowsSources(Map seqenceFlowsSources) {
this.seqenceFlowsSources = seqenceFlowsSources;
}
public void addSequenceFlowSource(String seqId, String sourceElemId) {
this.seqenceFlowsSources.put(seqId, sourceElemId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy