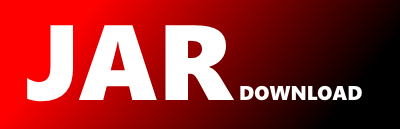
org.dspace.app.util.DCInputSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dspace-api Show documentation
Show all versions of dspace-api Show documentation
DSpace core data model and service APIs.
/**
* The contents of this file are subject to the license and copyright
* detailed in the LICENSE and NOTICE files at the root of the source
* tree and available online at
*
* http://www.dspace.org/license/
*/
package org.dspace.app.util;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
import org.dspace.core.Utils;
/**
* Class representing all DC inputs required for a submission, organized into pages
*
* @author Brian S. Hughes, based on work by Jenny Toves, OCLC
* @version $Revision$
*/
public class DCInputSet {
/**
* name of the input set
*/
private String formName = null;
/**
* the inputs ordered by row position
*/
private DCInput[][] inputs = null;
/**
* constructor
*
* @param formName form name
* @param mandatoryFlags
* @param rows the rows
* @param listMap map
*/
public DCInputSet(String formName, List>> rows, Map> listMap) {
this.formName = formName;
this.inputs = new DCInput[rows.size()][];
for (int i = 0; i < inputs.length; i++) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy