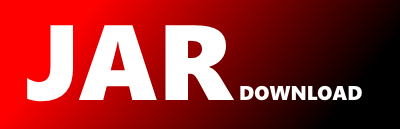
org.dspace.authority.PersonAuthorityValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dspace-api Show documentation
Show all versions of dspace-api Show documentation
DSpace core data model and service APIs.
/**
* The contents of this file are subject to the license and copyright
* detailed in the LICENSE and NOTICE files at the root of the source
* tree and available online at
*
* http://www.dspace.org/license/
*/
package org.dspace.authority;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import org.apache.commons.lang3.StringUtils;
import org.apache.solr.common.SolrDocument;
import org.apache.solr.common.SolrInputDocument;
/**
* @author Antoine Snyers (antoine at atmire.com)
* @author Kevin Van de Velde (kevin at atmire dot com)
* @author Ben Bosman (ben at atmire dot com)
* @author Mark Diggory (markd at atmire dot com)
*/
public class PersonAuthorityValue extends AuthorityValue {
private String firstName;
private String lastName;
private List nameVariants = new ArrayList();
private String institution;
private List emails = new ArrayList();
public PersonAuthorityValue() {
}
public PersonAuthorityValue(SolrDocument document) {
super(document);
}
public String getName() {
String name = "";
if (StringUtils.isNotBlank(lastName)) {
name = lastName;
if (StringUtils.isNotBlank(firstName)) {
name += ", ";
}
}
if (StringUtils.isNotBlank(firstName)) {
name += firstName;
}
return name;
}
public void setName(String name) {
if (StringUtils.isNotBlank(name)) {
String[] split = name.split(",");
if (split.length > 0) {
setLastName(split[0].trim());
if (split.length > 1) {
setFirstName(split[1].trim());
}
}
}
if (!StringUtils.equals(getValue(), name)) {
setValue(name);
}
}
@Override
public void setValue(String value) {
super.setValue(value);
setName(value);
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public List getNameVariants() {
return nameVariants;
}
public void addNameVariant(String name) {
if (StringUtils.isNotBlank(name)) {
nameVariants.add(name);
}
}
public String getInstitution() {
return institution;
}
public void setInstitution(String institution) {
this.institution = institution;
}
public List getEmails() {
return emails;
}
public void addEmail(String email) {
if (StringUtils.isNotBlank(email)) {
emails.add(email);
}
}
@Override
public SolrInputDocument getSolrInputDocument() {
SolrInputDocument doc = super.getSolrInputDocument();
if (StringUtils.isNotBlank(getFirstName())) {
doc.addField("first_name", getFirstName());
}
if (StringUtils.isNotBlank(getLastName())) {
doc.addField("last_name", getLastName());
}
for (String nameVariant : getNameVariants()) {
doc.addField("name_variant", nameVariant);
}
for (String email : emails) {
doc.addField("email", email);
}
doc.addField("institution", getInstitution());
return doc;
}
@Override
public void setValues(SolrDocument document) {
super.setValues(document);
this.firstName = Objects.toString(document.getFieldValue("first_name"), "");
this.lastName = Objects.toString(document.getFieldValue("last_name"), "");
nameVariants = new ArrayList();
Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy