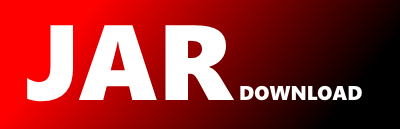
org.dspace.checker.DailyReportEmailer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dspace-api Show documentation
Show all versions of dspace-api Show documentation
DSpace core data model and service APIs.
/**
* The contents of this file are subject to the license and copyright
* detailed in the LICENSE and NOTICE files at the root of the source
* tree and available online at
*
* http://www.dspace.org/license/
*/
package org.dspace.checker;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.sql.SQLException;
import java.util.Date;
import java.util.GregorianCalendar;
import javax.mail.MessagingException;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.HelpFormatter;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.dspace.checker.factory.CheckerServiceFactory;
import org.dspace.checker.service.SimpleReporterService;
import org.dspace.core.Context;
import org.dspace.core.Email;
import org.dspace.core.Utils;
import org.dspace.services.ConfigurationService;
import org.dspace.services.factory.DSpaceServicesFactory;
/**
*
* The email reporter creates and sends emails to an administrator. This only
* reports information for today's date. It is expected this will be used just
* after the checksum checker has been run.
*
*
* @author Jim Downing
* @author Grace Carpenter
* @author Nathan Sarr
*/
public class DailyReportEmailer {
/**
* log4j logger.
*/
private static final Logger log = LogManager.getLogger(DailyReportEmailer.class);
/**
* Default constructor.
*/
public DailyReportEmailer() {
}
/**
* Send the report through email.
*
* @param attachment the file containing the report
* @param numberOfBitstreams the number of bitstreams reported
* @throws IOException if IO exception occurs
* @throws MessagingException if message cannot be sent.
*/
public void sendReport(File attachment, int numberOfBitstreams)
throws IOException, javax.mail.MessagingException {
if (numberOfBitstreams > 0) {
ConfigurationService configurationService
= DSpaceServicesFactory.getInstance().getConfigurationService();
String hostname = Utils.getHostName(configurationService.getProperty("dspace.ui.url"));
Email email = new Email();
email.setSubject(String.format(
"Checksum checker Report - %d Bitstreams found with POSSIBLE issues on %s",
numberOfBitstreams, hostname));
email.setContent("Checker Report", "report is attached ...");
email.addAttachment(attachment, "checksum_checker_report.txt");
email.addRecipient(configurationService.getProperty("mail.admin"));
email.send();
}
}
/**
* Allows users to have email sent to them. The default is to send all
* reports in one email
*
*
* - -h
* - help
* - -d
* - Select deleted bitstreams
* - -m
* - Bitstreams missing from assetstore
* - -c
* - Bitstreams whose checksums were changed
* - -n
* - Bitstreams whose checksums were changed
* - -a
* - Send all reports in one email
*
*
* @param args the command line arguments given
*/
public static void main(String[] args) {
// set up command line parser
CommandLineParser parser = new DefaultParser();
CommandLine line = null;
// create an options object and populate it
Options options = new Options();
options.addOption("h", "help", false, "Help");
options.addOption("d", "Deleted", false,
"Send E-mail report for all bitstreams set as deleted for today");
options.addOption("m", "Missing", false,
"Send E-mail report for all bitstreams not found in assetstore for today");
options.addOption("c", "Changed", false,
"Send E-mail report for all bitstreams where checksum has been changed for today");
options.addOption("a", "All", false,
"Send all E-mail reports");
options.addOption("u", "Unchecked", false,
"Send the Unchecked bitstream report");
options.addOption("n", "Not Processed", false,
"Send E-mail report for all bitstreams set to longer be processed for today");
try {
line = parser.parse(options, args);
} catch (ParseException e) {
log.fatal(e);
System.exit(1);
}
// user asks for help
if (line.hasOption('h')) {
HelpFormatter myhelp = new HelpFormatter();
myhelp.printHelp("Checksum Reporter\n", options);
System.out.println("\nSend Deleted bitstream email report: DailyReportEmailer -d");
System.out.println("\nSend Missing bitstreams email report: DailyReportEmailer -m");
System.out.println("\nSend Checksum Changed email report: DailyReportEmailer -c");
System.out.println("\nSend bitstream not to be processed email report: DailyReportEmailer -n");
System.out.println("\nSend Un-checked bitstream report: DailyReportEmailer -u");
System.out.println("\nSend All email reports: DailyReportEmailer");
System.exit(0);
}
// create a new simple reporter
SimpleReporterService reporter = CheckerServiceFactory.getInstance().getSimpleReporterService();
DailyReportEmailer emailer = new DailyReportEmailer();
// get dates for yesterday and tomorrow
GregorianCalendar calendar = new GregorianCalendar();
calendar.add(GregorianCalendar.DAY_OF_YEAR, -1);
Date yesterday = calendar.getTime();
calendar.add(GregorianCalendar.DAY_OF_YEAR, 2);
Date tomorrow = calendar.getTime();
File report = null;
FileWriter writer = null;
Context context = null;
try {
context = new Context(Context.Mode.READ_ONLY);
// the number of bitstreams in report
int numBitstreams = 0;
// create a temporary file in the log directory
ConfigurationService configurationService
= DSpaceServicesFactory.getInstance().getConfigurationService();
String dirLocation = configurationService.getProperty("log.report.dir");
File directory = new File(dirLocation);
if (directory.exists() && directory.isDirectory()) {
report = File.createTempFile("checker_report", ".txt",
directory);
} else {
throw new IllegalStateException("directory :" + dirLocation
+ " does not exist");
}
writer = new FileWriter(report);
if ((line.hasOption("a")) || (line.getOptions().length == 0)) {
writer.write("\n--------------------------------- Begin Reporting ------------------------\n\n");
numBitstreams += reporter.getDeletedBitstreamReport(context, yesterday, tomorrow, writer);
writer.write("\n--------------------------------- Report Spacer ---------------------------\n\n");
numBitstreams += reporter.getChangedChecksumReport(context, yesterday, tomorrow, writer);
writer.write("\n--------------------------------- Report Spacer ---------------------------\n\n");
numBitstreams += reporter.getBitstreamNotFoundReport(context, yesterday, tomorrow, writer);
writer.write("\n--------------------------------- Report Spacer ---------------------------\n\n");
numBitstreams += reporter.getNotToBeProcessedReport(context, yesterday, tomorrow, writer);
writer.write("\n--------------------------------- Report Spacer ---------------------------\n\n");
numBitstreams += reporter.getUncheckedBitstreamsReport(context, writer);
writer.write("\n--------------------------------- End Report ---------------------------\n\n");
writer.flush();
writer.close();
emailer.sendReport(report, numBitstreams);
} else {
if (line.hasOption("d")) {
writer.write("\n--------------------------------- Begin Reporting ------------------------\n\n");
numBitstreams += reporter.getDeletedBitstreamReport(context,
yesterday, tomorrow, writer);
writer.flush();
writer.close();
emailer.sendReport(report, numBitstreams);
}
if (line.hasOption("m")) {
writer.write("\n--------------------------------- Begin Reporting ------------------------\n\n");
numBitstreams += reporter.getBitstreamNotFoundReport(context,
yesterday, tomorrow, writer);
writer.flush();
writer.close();
emailer.sendReport(report, numBitstreams);
}
if (line.hasOption("c")) {
writer.write("\n--------------------------------- Begin Reporting ------------------------\n\n");
numBitstreams += reporter.getChangedChecksumReport(context,
yesterday, tomorrow, writer);
writer.flush();
writer.close();
emailer.sendReport(report, numBitstreams);
}
if (line.hasOption("n")) {
writer.write("\n--------------------------------- Begin Reporting ------------------------\n\n");
numBitstreams += reporter.getNotToBeProcessedReport(context,
yesterday, tomorrow, writer);
writer.flush();
writer.close();
emailer.sendReport(report, numBitstreams);
}
if (line.hasOption("u")) {
writer.write("\n--------------------------------- Begin Reporting ------------------------\n\n");
numBitstreams += reporter
.getUncheckedBitstreamsReport(context, writer);
writer.flush();
writer.close();
emailer.sendReport(report, numBitstreams);
}
}
} catch (MessagingException | SQLException | IOException e) {
log.fatal(e);
} finally {
if (context != null && context.isValid()) {
context.abort();
}
if (writer != null) {
try {
writer.close();
} catch (IOException e) {
log.fatal("Could not close writer", e);
}
}
if (report != null && report.exists()) {
if (!report.delete()) {
log.error("Unable to delete report file");
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy