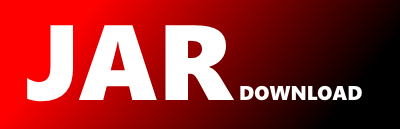
org.dspace.content.dao.impl.BitstreamDAOImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dspace-api Show documentation
Show all versions of dspace-api Show documentation
DSpace core data model and service APIs.
/**
* The contents of this file are subject to the license and copyright
* detailed in the LICENSE and NOTICE files at the root of the source
* tree and available online at
*
* http://www.dspace.org/license/
*/
package org.dspace.content.dao.impl;
import java.sql.SQLException;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.persistence.Query;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Root;
import org.dspace.content.Bitstream;
import org.dspace.content.Bitstream_;
import org.dspace.content.Collection;
import org.dspace.content.Community;
import org.dspace.content.Item;
import org.dspace.content.dao.BitstreamDAO;
import org.dspace.core.AbstractHibernateDSODAO;
import org.dspace.core.Constants;
import org.dspace.core.Context;
/**
* Hibernate implementation of the Database Access Object interface class for the Bitstream object.
* This class is responsible for all database calls for the Bitstream object and is autowired by spring
* This class should never be accessed directly.
*
* @author kevinvandevelde at atmire.com
*/
public class BitstreamDAOImpl extends AbstractHibernateDSODAO implements BitstreamDAO {
protected BitstreamDAOImpl() {
super();
}
@Override
public List findDeletedBitstreams(Context context) throws SQLException {
CriteriaBuilder criteriaBuilder = getCriteriaBuilder(context);
CriteriaQuery criteriaQuery = getCriteriaQuery(criteriaBuilder, Bitstream.class);
Root bitstreamRoot = criteriaQuery.from(Bitstream.class);
criteriaQuery.select(bitstreamRoot);
criteriaQuery.where(criteriaBuilder.equal(bitstreamRoot.get(Bitstream_.deleted), true));
return list(context, criteriaQuery, false, Bitstream.class, -1, -1);
}
@Override
public List findDuplicateInternalIdentifier(Context context, Bitstream bitstream) throws SQLException {
CriteriaBuilder criteriaBuilder = getCriteriaBuilder(context);
CriteriaQuery criteriaQuery = getCriteriaQuery(criteriaBuilder, Bitstream.class);
Root bitstreamRoot = criteriaQuery.from(Bitstream.class);
criteriaQuery.select(bitstreamRoot);
criteriaQuery.where(criteriaBuilder.and(
criteriaBuilder.equal(bitstreamRoot.get(Bitstream_.internalId), bitstream.getInternalId()),
criteriaBuilder.notEqual(bitstreamRoot.get(Bitstream_.id), bitstream.getID())
)
);
return list(context, criteriaQuery, false, Bitstream.class, -1, -1);
}
@Override
public List findBitstreamsWithNoRecentChecksum(Context context) throws SQLException {
Query query = createQuery(context,
"select b from Bitstream b where b not in (select c.bitstream from " +
"MostRecentChecksum c)");
return query.getResultList();
}
@Override
public Iterator findByCommunity(Context context, Community community) throws SQLException {
Query query = createQuery(context, "select b from Bitstream b " +
"join b.bundles bitBundles " +
"join bitBundles.items item " +
"join item.collections itemColl " +
"join itemColl.communities community " +
"WHERE :community IN community");
query.setParameter("community", community);
return iterate(query);
}
@Override
public Iterator findByCollection(Context context, Collection collection) throws SQLException {
Query query = createQuery(context, "select b from Bitstream b " +
"join b.bundles bitBundles " +
"join bitBundles.items item " +
"join item.collections c " +
"WHERE :collection IN c");
query.setParameter("collection", collection);
return iterate(query);
}
@Override
public Iterator findByItem(Context context, Item item) throws SQLException {
Query query = createQuery(context, "select b from Bitstream b " +
"join b.bundles bitBundles " +
"join bitBundles.items item " +
"WHERE :item IN item");
query.setParameter("item", item);
return iterate(query);
}
@Override
public Iterator findByStoreNumber(Context context, Integer storeNumber) throws SQLException {
Query query = createQuery(context, "select b from Bitstream b where b.storeNumber = :storeNumber");
query.setParameter("storeNumber", storeNumber);
return iterate(query);
}
@Override
public Long countByStoreNumber(Context context, Integer storeNumber) throws SQLException {
CriteriaBuilder criteriaBuilder = getCriteriaBuilder(context);
CriteriaQuery criteriaQuery = criteriaBuilder.createQuery(Long.class);
Root bitstreamRoot = criteriaQuery.from(Bitstream.class);
criteriaQuery.where(criteriaBuilder.equal(bitstreamRoot.get(Bitstream_.storeNumber), storeNumber));
return countLong(context, criteriaQuery, criteriaBuilder, bitstreamRoot);
}
@Override
public int countRows(Context context) throws SQLException {
return count(createQuery(context, "SELECT count(*) from Bitstream"));
}
@Override
public int countDeleted(Context context) throws SQLException {
return count(createQuery(context, "SELECT count(*) FROM Bitstream b WHERE b.deleted=true"));
}
@Override
public int countWithNoPolicy(Context context) throws SQLException {
Query query = createQuery(context,
"SELECT count(bit.id) from Bitstream bit where bit.deleted<>true and bit.id not in" +
" (select res.dSpaceObject from ResourcePolicy res where res.resourceTypeId = " +
":typeId )");
query.setParameter("typeId", Constants.BITSTREAM);
return count(query);
}
@Override
public List getNotReferencedBitstreams(Context context) throws SQLException {
return list(createQuery(context, "select bit from Bitstream bit where bit.deleted != true" +
" and bit.id not in (select bit2.id from Bundle bun join bun.bitstreams bit2)" +
" and bit.id not in (select com.logo.id from Community com)" +
" and bit.id not in (select col.logo.id from Collection col)" +
" and bit.id not in (select bun.primaryBitstream.id from Bundle bun)"));
}
@Override
public Iterator findAll(Context context, int limit, int offset) throws SQLException {
Map map = new HashMap<>();
return findByX(context, Bitstream.class, map, true, limit, offset).iterator();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy