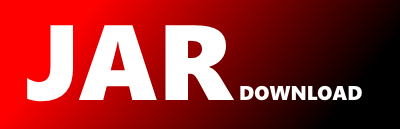
org.dspace.rdf.conversion.DMRM Maven / Gradle / Ivy
Show all versions of dspace-api Show documentation
/**
* The contents of this file are subject to the license and copyright
* detailed in the LICENSE and NOTICE files at the root of the source
* tree and available online at
*
* http://www.dspace.org/license/
*/
package org.dspace.rdf.conversion;
import com.hp.hpl.jena.rdf.model.Model;
import com.hp.hpl.jena.rdf.model.ModelFactory;
import com.hp.hpl.jena.rdf.model.Property;
import com.hp.hpl.jena.rdf.model.Resource;
/**
* Schema for DSpace Metadata RDF Mappings.
*
* @author Pascal-Nicolas Becker (dspace -at- pascal -hyphen- becker -dot- de)
* @see http://digital
* -repositories.org/ontologies/dspace-metadata-mapping/0.2.0
*/
public class DMRM {
/**
* The RDF model that holds the vocabulary terms
*/
private static Model m_model = ModelFactory.createDefaultModel();
/**
* The namespace of the vocabulary as a string
*/
public static final String NS = "http://digital-repositories.org/ontologies/dspace-metadata-mapping/0.2.0#";
/**
* The namespace of the vocabulary as a string
*
* @return Namespace URI
* @see #NS
*/
public static String getURI() {
return NS;
}
/**
* The namespace of the vocabulary as a resource
*/
public static final Resource NAMESPACE = m_model.createResource(NS);
/**
* Represents the mapping of a DSpace metadata value to an RDF equivalent.
*/
public static final Resource DSpaceMetadataRDFMapping = m_model.createResource(NS + "DSpaceMetadataRDFMapping");
/**
* A reified statement that describes the result of the DSpaceMetadataRDFMapping.
*/
public static final Resource Result = m_model.createResource(NS + "Result");
/**
* Processes a metadata value into an RDF value or an IRI.
*/
public static final Resource ValueProcessor = m_model.createResource(NS + "ValueProcessor");
/**
* A regular expression to be used with java, composed of a matching and a replaying expression.
*/
public static final Resource ValueModifier = m_model.createResource(NS + "ValueModifier");
/**
* Generates a literal depending on a DSpace metadata value.
*/
public static final Resource LiteralGenerator = m_model.createResource(NS + "LiteralGenerator");
/**
* Generates an IRI used for a rdfs:Resource depending on the converted DSpace Object and one of its metadata
* values.
*/
public static final Resource ResourceGenerator = m_model.createResource(NS + "ResourceGenerator");
/**
* Placeholder for the IRI of the DSpace Object that gets converted.
*/
public static final Resource DSpaceObjectIRI = m_model.createResource(NS + "DSpaceObjectIRI");
/**
* Shortcut to generate a Literal containing an unchanged metadata value.
*/
public static final Resource DSpaceValue = m_model.createResource(NS + "DSpaceValue");
/**
* Specifies the RDF to generate for a specified matadata.
*/
public static final Property creates = m_model.createProperty(NS + "creates");
/**
* The subject of a DSpace metadata RDF mapping result.
*/
public static final Property subject = m_model.createProperty(NS + "subject");
/**
* The predicate of a DSpace metadata RDF mapping result.
*/
public static final Property predicate = m_model.createProperty(NS + "predicate");
/**
* The object of a DSpace metadata RDF mapping result.
*/
public static final Property object = m_model.createProperty(NS + "object");
/**
* The name of the metadata to convert (e.g. dc.title).
*/
public static final Property metadataName = m_model.createProperty(NS + "metadataName");
/**
* A regex that the metadata value has to fulfill if the mapping should become active.
*/
public static final Property condition = m_model.createProperty(NS + "condition");
/**
* Information how the metadata value should be modified before it is inserted in the pattern.
*/
public static final Property modifier = m_model.createProperty(NS + "modifier");
/**
* A regex that matches those subsequences of a metadata value, that should be replaced.
*/
public static final Property matcher = m_model.createProperty(NS + "matcher");
/**
* A regex that replaces previously matched subsequences of a metadata value.
*/
public static final Property replacement = m_model.createProperty(NS + "replacement");
/**
* A pattern that contains $DSpaceValue as placeholder for the metadata value.
*/
public static final Property pattern = m_model.createProperty(NS + "pattern");
/**
* Defines the datatype a generated literal gets.
*/
public static final Property literalType = m_model.createProperty(NS + "literalType");
/**
* Defines the language a literal uses. Maybe overridden by #dspaceLanguageTag.
*/
public static final Property literalLanguage = m_model.createProperty(NS + "literalLanguage");
/**
* Defines to use the language tag of a DSpace metadata value.
*/
public static final Property dspaceLanguageTag = m_model.createProperty(NS + "dspaceLanguageTag");
/**
* Default constructor
*/
private DMRM() { }
}