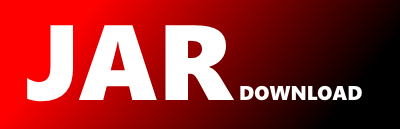
org.dspace.xoai.model.oaipmh.Request Maven / Gradle / Ivy
The newest version!
/*
* The contents of this file are subject to the license and copyright
* detailed in the LICENSE and NOTICE files at the root of the source
* tree and available online at
*
* http://www.dspace.org/license/
*/
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.2-147
// See client://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.01.13 at 08:24:23 PM WET
//
package org.dspace.xoai.model.oaipmh;
import com.lyncode.xml.exceptions.XmlWriteException;
import org.dspace.xoai.xml.XmlWritable;
import org.dspace.xoai.xml.XmlWriter;
import javax.xml.stream.XMLStreamException;
import java.util.Date;
public class Request implements XmlWritable {
private final String baseUrl;
private String verbType;
private String identifier;
private String metadataPrefix;
private Date from;
private Date until;
private String set;
private String resumptionToken;
private String fromString;
private String untilString;
public Request(String baseUrl) {
this.baseUrl = baseUrl;
}
public String getBaseUrl() {
return baseUrl;
}
public Verb.Type getVerbType() {
return Verb.Type.fromValue(verbType);
}
public String getVerb () {
return verbType;
}
public Request withVerbType(Verb.Type value) {
this.verbType = value.displayName();
return this;
}
public Request withVerbType (String verb) {
this.verbType = verb;
return this;
}
public String getIdentifier() {
return identifier;
}
public Request withIdentifier(String value) {
this.identifier = value;
return this;
}
public String getMetadataPrefix() {
return metadataPrefix;
}
public Request withMetadataPrefix(String value) {
this.metadataPrefix = value;
return this;
}
public Date getFrom() {
return from;
}
public Request withFrom(Date value) {
this.from = value;
return this;
}
public Date getUntil() {
return until;
}
public Request withUntil(Date value) {
this.until = value;
return this;
}
public String getSet() {
return set;
}
public Request withSet(String value) {
this.set = value;
return this;
}
public String getResumptionToken() {
return resumptionToken;
}
public Request withResumptionToken(String value) {
this.resumptionToken = value;
return this;
}
public Request withFrom(String value) {
this.fromString = value;
return this;
}
public Request withUntil(String value) {
this.untilString = value;
return this;
}
@Override
public void write(XmlWriter writer) throws XmlWriteException {
try {
writer.writeAttribute("verb", verbType);
writer.writeAttribute("identifier", identifier);
writer.writeAttribute("metadataPrefix", metadataPrefix);
if (from != null)
writer.writeAttribute("from", from);
else
writer.writeAttribute("from", fromString);
if (until != null)
writer.writeAttribute("until", until);
else
writer.writeAttribute("until", untilString);
writer.writeAttribute("set", set);
writer.writeAttribute("resumptionToken", resumptionToken);
writer.writeCharacters(baseUrl);
} catch (XMLStreamException e) {
throw new XmlWriteException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy