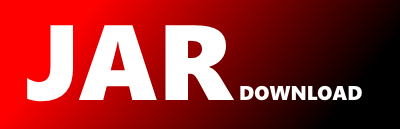
org.dstadler.commons.collections.ConcurrentMappedCounter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons-dost Show documentation
Show all versions of commons-dost Show documentation
Common utilities I find useful in many of my projects.
package org.dstadler.commons.collections;
import java.util.Map;
import java.util.Set;
/**
* Thread-Safe variant of {@link MappedCounter},
* currently internally delegates to a {@link MappedCounterImpl}
*
* @param
* @author dominik.stadler
*/
public class ConcurrentMappedCounter implements MappedCounter {
// simply delegate to a normal MappedCounter in synchronized blocks for now
// a better implementation, e.g. by using ConcurrentHashMap can be added later if necessary
private MappedCounter counter = new MappedCounterImpl<>();
@Override
public void addInt(T k, int v) {
synchronized (counter) {
counter.addInt(k, v);
}
}
@Override
public int get(T k) {
synchronized (counter) {
return counter.get(k);
}
}
@Override
public int remove(T key) {
synchronized (counter) {
return counter.remove(key);
}
}
@Override
public Set keys() {
synchronized (counter) {
return counter.keys();
}
}
@Override
public Set> entries() {
synchronized (counter) {
return counter.entries();
}
}
@Override
public Map sortedMap() {
synchronized (counter) {
return counter.sortedMap();
}
}
@Override
public int sum() {
synchronized (counter) {
return counter.sum();
}
}
@Override
public String toString() {
return "Concurrent: " + counter.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy