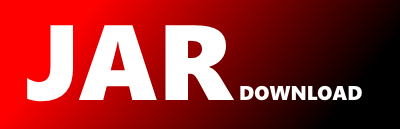
org.duckdb.DuckDBArray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of duckdb_jdbc Show documentation
Show all versions of duckdb_jdbc Show documentation
A JDBC-Compliant driver for the DuckDB data management system
The newest version!
package org.duckdb;
import static org.duckdb.DuckDBResultSetMetaData.type_to_int;
import java.sql.Array;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Arrays;
import java.util.Map;
public class DuckDBArray implements Array {
private final Object[] array;
private final DuckDBVector vector;
final int offset, length;
DuckDBArray(DuckDBVector vector, int offset, int length) throws SQLException {
this.vector = vector;
this.length = length;
this.offset = offset;
array = new Object[length];
for (int i = 0; i < length; i++) {
array[i] = vector.getObject(offset + i);
}
}
@Override
public void free() throws SQLException {
// we don't own the vector, so cannot free it
}
@Override
public Object getArray() throws SQLException {
return array;
}
@Override
public Object getArray(Map> map) throws SQLException {
return getArray();
}
@Override
public Object getArray(long index, int count) throws SQLException {
// TODO Auto-generated method stub
throw new UnsupportedOperationException("Unimplemented method 'getArray'");
}
@Override
public Object getArray(long index, int count, Map> map) throws SQLException {
// TODO Auto-generated method stub
throw new UnsupportedOperationException("Unimplemented method 'getArray'");
}
@Override
public int getBaseType() throws SQLException {
return type_to_int(vector.duckdb_type);
}
@Override
public String getBaseTypeName() throws SQLException {
return vector.duckdb_type.name();
}
@Override
public ResultSet getResultSet() throws SQLException {
return new DuckDBArrayResultSet(vector, offset, length);
}
@Override
public ResultSet getResultSet(Map> map) throws SQLException {
// TODO Auto-generated method stub
throw new UnsupportedOperationException("Unimplemented method 'getResultSet'");
}
@Override
public ResultSet getResultSet(long index, int count) throws SQLException {
// TODO Auto-generated method stub
throw new UnsupportedOperationException("Unimplemented method 'getResultSet'");
}
@Override
public ResultSet getResultSet(long index, int count, Map> map) throws SQLException {
// TODO Auto-generated method stub
throw new UnsupportedOperationException("Unimplemented method 'getResultSet'");
}
@Override
public String toString() {
return Arrays.toString(array);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy