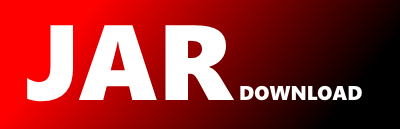
org.echocat.jomon.process.daemon.ProcessDaemonRequirement Maven / Gradle / Ivy
/*****************************************************************************************
* *** BEGIN LICENSE BLOCK *****
*
* Version: MPL 2.0
*
* echocat Jomon, Copyright (c) 2012-2013 echocat
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*
* *** END LICENSE BLOCK *****
****************************************************************************************/
package org.echocat.jomon.process.daemon;
import com.google.common.base.Predicate;
import org.echocat.jomon.process.GeneratedProcess;
import org.echocat.jomon.process.listeners.startup.StartupListenerBasedRequirement;
import org.echocat.jomon.runtime.annotations.Excluding;
import org.echocat.jomon.runtime.annotations.Including;
import org.echocat.jomon.runtime.numbers.IntegerRange;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.Set;
import static org.echocat.jomon.runtime.CollectionUtils.asSet;
public interface ProcessDaemonRequirement, T extends ProcessDaemon> extends StartupListenerBasedRequirement {
public static final Predicate DEFAULT_EXIT_CODE_VALIDATOR = new Predicate() { @Override public boolean apply(@Nullable Integer input) {
return input != null && input == 0;
}};
@Nonnull
public Class getType();
@Nonnull
public Predicate getExitCodeValidator();
public abstract static class Base, T extends ProcessDaemon, B extends Base> extends StartupListenerBasedRequirement.Base implements ProcessDaemonRequirement {
@Nonnull
private final Class _type;
@Nonnull
private Predicate _exitCodeValidator = DEFAULT_EXIT_CODE_VALIDATOR;
public Base(@Nonnull Class type) {
_type = type;
}
@Nonnull
@Override
public Class getType() {
return _type;
}
@Nonnull
public B withExitCodeValidator(@Nonnull Predicate validator) {
_exitCodeValidator = validator;
return thisObject();
}
@Nonnull
public B withExitCodeValidatorThatMatches(@Nullable Integer exitCode) {
return withExitCodeValidatorThatMatches(asSet(exitCode));
}
@Nonnull
public B withExitCodeValidatorThatMatches(@Nullable Integer... exitCodes) {
return withExitCodeValidatorThatMatches(asSet(exitCodes));
}
@Nonnull
public B withExitCodeValidatorThatMatches(@Nullable final Iterable exitCodes) {
final Set potentials = asSet(exitCodes);
return withExitCodeValidator(new Predicate() {
@Override
public boolean apply(@Nullable Integer input) {
return potentials.contains(input);
}
});
}
@Nonnull
public B withExitCodeValidatorThatMatchesBetween(@Nullable @Including Integer from, @Nullable @Excluding Integer to) {
return withExitCodeValidator(new IntegerRange(from, to));
}
@Override
@Nonnull
public Predicate getExitCodeValidator() {
return _exitCodeValidator;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy