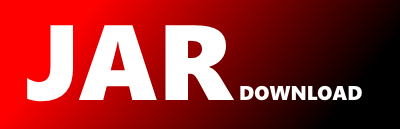
org.echocat.locela.api.java.properties.StandardProperties Maven / Gradle / Ivy
package org.echocat.locela.api.java.properties;
import org.echocat.locela.api.java.annotations.Annotation;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.annotation.concurrent.NotThreadSafe;
import java.util.LinkedHashMap;
import java.util.Map;
@NotThreadSafe
public class StandardProperties extends PropertiesSupport {
@SafeVarargs
@Nonnull
public static StandardProperties properties(@Nullable Property... properties) {
return new StandardProperties().withProperties(properties);
}
@Nonnull
private final Map> _idToProperty = new LinkedHashMap<>();
@Nonnull
protected Map> idToProperty() {
return _idToProperty;
}
@Override
public void add(@Nonnull Property extends V> property) {
// noinspection unchecked
idToProperty().put(property.getId(), (Property) property);
}
@Nullable
@Override
public Property get(@Nonnull String id) {
return idToProperty().get(id);
}
@Override
public boolean contains(@Nonnull String id) {
return idToProperty().containsKey(id);
}
@Override
public void remove(@Nonnull String id) {
idToProperty().remove(id);
}
@Nonnull
@Override
protected Iterable extends Property> getProperties() {
return idToProperty().values();
}
@Nonnull
public StandardProperties withAnnotations(@Nullable Annotation... annotations) {
addAnnotations(annotations);
return this;
}
@Nonnull
public StandardProperties withAnnotations(@Nullable Iterable extends Annotation> annotations) {
addAnnotations(annotations);
return this;
}
@Nonnull
public StandardProperties withProperties(@Nullable Property>... properties) {
if (properties != null) {
for (final Property> property : properties) {
// noinspection unchecked
_idToProperty.put(property.getId(), (Property) property);
}
}
return this;
}
@Nonnull
public StandardProperties withProperties(@Nullable Iterable extends Property extends V>> properties) {
if (properties != null) {
for (final Property> property : properties) {
// noinspection unchecked
_idToProperty.put(property.getId(), (Property) property);
}
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy