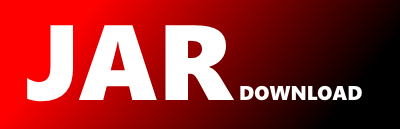
org.eclipse.collections.impl.test.Verify Maven / Gradle / Ivy
/*
* Copyright (c) 2016 Goldman Sachs.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.test;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.NotSerializableException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.ObjectStreamClass;
import java.io.Serializable;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.SortedSet;
import java.util.concurrent.Callable;
import org.apache.commons.codec.binary.Base64;
import org.eclipse.collections.api.InternalIterable;
import org.eclipse.collections.api.PrimitiveIterable;
import org.eclipse.collections.api.bag.Bag;
import org.eclipse.collections.api.bag.sorted.SortedBag;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.procedure.primitive.ObjectIntProcedure;
import org.eclipse.collections.api.collection.ImmutableCollection;
import org.eclipse.collections.api.list.ImmutableList;
import org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.api.map.ImmutableMapIterable;
import org.eclipse.collections.api.map.MapIterable;
import org.eclipse.collections.api.map.MutableMap;
import org.eclipse.collections.api.map.MutableMapIterable;
import org.eclipse.collections.api.map.sorted.SortedMapIterable;
import org.eclipse.collections.api.multimap.Multimap;
import org.eclipse.collections.api.multimap.bag.BagMultimap;
import org.eclipse.collections.api.multimap.list.ListMultimap;
import org.eclipse.collections.api.multimap.set.SetMultimap;
import org.eclipse.collections.api.multimap.sortedbag.SortedBagMultimap;
import org.eclipse.collections.api.multimap.sortedset.SortedSetMultimap;
import org.eclipse.collections.api.set.ImmutableSet;
import org.eclipse.collections.api.set.MutableSet;
import org.eclipse.collections.impl.block.factory.Comparators;
import org.eclipse.collections.impl.block.factory.Predicates;
import org.eclipse.collections.impl.block.procedure.CollectionAddProcedure;
import org.eclipse.collections.impl.factory.Lists;
import org.eclipse.collections.impl.factory.Sets;
import org.eclipse.collections.impl.list.mutable.FastList;
import org.eclipse.collections.impl.map.mutable.UnifiedMap;
import org.eclipse.collections.impl.set.mutable.UnifiedSet;
import org.eclipse.collections.impl.tuple.ImmutableEntry;
import org.eclipse.collections.impl.utility.ArrayIterate;
import org.eclipse.collections.impl.utility.Iterate;
import org.junit.Assert;
/**
* An extension of the {@link Assert} class, which adds useful additional "assert" methods.
* You can import this class instead of Assert, and use it thus, e.g.:
*
* Verify.assertEquals("fred", name); // from original Assert class
* Verify.assertContains("fred", nameList); // from new extensions
* Verify.assertBefore("fred", "jim", orderedNamesList); // from new extensions
*
*/
public final class Verify extends Assert
{
private static final int MAX_DIFFERENCES = 5;
private static final byte[] LINE_SEPARATOR = {'\n'};
private Verify()
{
throw new AssertionError("Suppress default constructor for noninstantiability");
}
/**
* Mangles the stack trace of {@link AssertionError} so that it looks like its been thrown from the line that
* called to a custom assertion.
*
* This method behaves identically to {@link #throwMangledException(AssertionError, int)} and is provided
* for convenience for assert methods that only want to pop two stack frames. The only time that you would want to
* call the other {@link #throwMangledException(AssertionError, int)} method is if you have a custom assert
* that calls another custom assert i.e. the source line calling the custom asserts is more than two stack frames
* away
*
* @param e The exception to mangle.
* @see #throwMangledException(AssertionError, int)
*/
public static void throwMangledException(AssertionError e)
{
/*
* Note that we actually remove 3 frames from the stack trace because
* we wrap the real method doing the work: e.fillInStackTrace() will
* include us in the exceptions stack frame.
*/
Verify.throwMangledException(e, 3);
}
/**
* Mangles the stack trace of {@link AssertionError} so that it looks like
* its been thrown from the line that called to a custom assertion.
*
* This is useful for when you are in a debugging session and you want to go to the source
* of the problem in the test case quickly. The regular use case for this would be something
* along the lines of:
*
* public class TestFoo extends junit.framework.TestCase
* {
* public void testFoo() throws Exception
* {
* Foo foo = new Foo();
* ...
* assertFoo(foo);
* }
*
* // Custom assert
* private static void assertFoo(Foo foo)
* {
* try
* {
* assertEquals(...);
* ...
* assertSame(...);
* }
* catch (AssertionFailedException e)
* {
* AssertUtils.throwMangledException(e, 2);
* }
* }
* }
*
*
* Without the {@code try ... catch} block around lines 11-13 the stack trace following a test failure
* would look a little like:
*
*
* java.lang.AssertionError: ...
* at TestFoo.assertFoo(TestFoo.java:11)
* at TestFoo.testFoo(TestFoo.java:5)
* at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
* at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
* at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
* at java.lang.reflect.Method.invoke(Method.java:324)
* ...
*
*
* Note that the source of the error isn't readily apparent as the first line in the stack trace
* is the code within the custom assert. If we were debugging the failure we would be more interested
* in the second line of the stack trace which shows us where in our tests the assert failed.
*
* With the {@code try ... catch} block around lines 11-13 the stack trace would look like the
* following:
*
*
* java.lang.AssertionError: ...
* at TestFoo.testFoo(TestFoo.java:5)
* at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
* at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
* at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
* at java.lang.reflect.Method.invoke(Method.java:324)
* ...
*
*
* Here the source of the error is more visible as we can instantly see that the testFoo test is
* failing at line 5.
*
* @param e The exception to mangle.
* @param framesToPop The number of frames to remove from the stack trace.
* @throws AssertionError that was given as an argument with its stack trace mangled.
*/
public static void throwMangledException(AssertionError e, int framesToPop)
{
e.fillInStackTrace();
StackTraceElement[] stackTrace = e.getStackTrace();
StackTraceElement[] newStackTrace = new StackTraceElement[stackTrace.length - framesToPop];
System.arraycopy(stackTrace, framesToPop, newStackTrace, 0, newStackTrace.length);
e.setStackTrace(newStackTrace);
throw e;
}
public static void fail(String message, Throwable cause)
{
AssertionError failedException = new AssertionError(message, cause);
Verify.throwMangledException(failedException);
}
/**
* Assert that two items are not the same. If one item is null, the the other must be non-null.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(String, Object, Object)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(String itemsName, Object item1, Object item2)
{
try
{
if (Comparators.nullSafeEquals(item1, item2) || Comparators.nullSafeEquals(item2, item1))
{
Assert.fail(itemsName + " should not be equal, item1:<" + item1 + ">, item2:<" + item2 + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that two items are not the same. If one item is null, the the other must be non-null.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(Object, Object)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(Object item1, Object item2)
{
try
{
Verify.assertNotEquals("items", item1, item2);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two Strings are not equal.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(String, Object, Object)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(String itemName, String notExpected, String actual)
{
try
{
if (Comparators.nullSafeEquals(notExpected, actual))
{
Assert.fail(itemName + " should not equal:<" + notExpected + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two Strings are not equal.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(Object, Object)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(String notExpected, String actual)
{
try
{
Verify.assertNotEquals("string", notExpected, actual);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two doubles are not equal concerning a delta. If the expected value is infinity then the delta value
* is ignored.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(String, double, double, double)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(String itemName, double notExpected, double actual, double delta)
{
// handle infinity specially since subtracting to infinite values gives NaN and the
// the following test fails
try
{
//noinspection FloatingPointEquality
if (Double.isInfinite(notExpected) && notExpected == actual || Math.abs(notExpected - actual) <= delta)
{
Assert.fail(itemName + " should not be equal:<" + notExpected + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two doubles are not equal concerning a delta. If the expected value is infinity then the delta value
* is ignored.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(double, double, double)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(double notExpected, double actual, double delta)
{
try
{
Verify.assertNotEquals("double", notExpected, actual, delta);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two floats are not equal concerning a delta. If the expected value is infinity then the delta value
* is ignored.
*/
public static void assertNotEquals(String itemName, float notExpected, float actual, float delta)
{
try
{
// handle infinity specially since subtracting to infinite values gives NaN and the
// the following test fails
//noinspection FloatingPointEquality
if (Float.isInfinite(notExpected) && notExpected == actual || Math.abs(notExpected - actual) <= delta)
{
Assert.fail(itemName + " should not be equal:<" + notExpected + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two floats are not equal concerning a delta. If the expected value is infinity then the delta value
* is ignored.
*/
public static void assertNotEquals(float expected, float actual, float delta)
{
try
{
Verify.assertNotEquals("float", expected, actual, delta);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two longs are not equal.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(String, long, long)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(String itemName, long notExpected, long actual)
{
try
{
if (notExpected == actual)
{
Assert.fail(itemName + " should not be equal:<" + notExpected + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two longs are not equal.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(long, long)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(long notExpected, long actual)
{
try
{
Verify.assertNotEquals("long", notExpected, actual);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two booleans are not equal.
*/
public static void assertNotEquals(String itemName, boolean notExpected, boolean actual)
{
try
{
if (notExpected == actual)
{
Assert.fail(itemName + " should not be equal:<" + notExpected + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two booleans are not equal.
*/
public static void assertNotEquals(boolean notExpected, boolean actual)
{
try
{
Verify.assertNotEquals("boolean", notExpected, actual);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two bytes are not equal.
*/
public static void assertNotEquals(String itemName, byte notExpected, byte actual)
{
try
{
if (notExpected == actual)
{
Assert.fail(itemName + " should not be equal:<" + notExpected + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two bytes are not equal.
*/
public static void assertNotEquals(byte notExpected, byte actual)
{
try
{
Verify.assertNotEquals("byte", notExpected, actual);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two chars are not equal.
*/
public static void assertNotEquals(String itemName, char notExpected, char actual)
{
try
{
if (notExpected == actual)
{
Assert.fail(itemName + " should not be equal:<" + notExpected + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two chars are not equal.
*/
public static void assertNotEquals(char notExpected, char actual)
{
try
{
Verify.assertNotEquals("char", notExpected, actual);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two shorts are not equal.
*/
public static void assertNotEquals(String itemName, short notExpected, short actual)
{
try
{
if (notExpected == actual)
{
Assert.fail(itemName + " should not be equal:<" + notExpected + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two shorts are not equal.
*/
public static void assertNotEquals(short notExpected, short actual)
{
try
{
Verify.assertNotEquals("short", notExpected, actual);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two ints are not equal.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(String, long, long)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(String itemName, int notExpected, int actual)
{
try
{
if (notExpected == actual)
{
Assert.fail(itemName + " should not be equal:<" + notExpected + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Asserts that two ints are not equal.
*
* @deprecated in 3.0. Use {@link Assert#assertNotEquals(long, long)} in JUnit 4.11 instead.
*/
@Deprecated
public static void assertNotEquals(int notExpected, int actual)
{
try
{
Verify.assertNotEquals("int", notExpected, actual);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Iterable} is empty.
*/
public static void assertEmpty(Iterable> actualIterable)
{
try
{
Verify.assertEmpty("iterable", actualIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Collection} is empty.
*/
public static void assertEmpty(String iterableName, Iterable> actualIterable)
{
try
{
Verify.assertObjectNotNull(iterableName, actualIterable);
if (Iterate.notEmpty(actualIterable))
{
Assert.fail(iterableName + " should be empty; actual size:<" + Iterate.sizeOf(actualIterable) + '>');
}
if (!Iterate.isEmpty(actualIterable))
{
Assert.fail(iterableName + " should be empty; actual size:<" + Iterate.sizeOf(actualIterable) + '>');
}
if (Iterate.sizeOf(actualIterable) != 0)
{
Assert.fail(iterableName + " should be empty; actual size:<" + Iterate.sizeOf(actualIterable) + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link MutableMapIterable} is empty.
*/
public static void assertEmpty(MutableMapIterable, ?> actualMutableMapIterable)
{
try
{
Verify.assertEmpty("mutableMapIterable", actualMutableMapIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Collection} is empty.
*/
public static void assertEmpty(String mutableMapIterableName, MutableMapIterable, ?> actualMutableMapIterable)
{
try
{
Verify.assertObjectNotNull(mutableMapIterableName, actualMutableMapIterable);
if (Iterate.notEmpty(actualMutableMapIterable))
{
Assert.fail(mutableMapIterableName + " should be empty; actual size:<" + Iterate.sizeOf(actualMutableMapIterable) + '>');
}
if (!Iterate.isEmpty(actualMutableMapIterable))
{
Assert.fail(mutableMapIterableName + " should be empty; actual size:<" + Iterate.sizeOf(actualMutableMapIterable) + '>');
}
if (!actualMutableMapIterable.isEmpty())
{
Assert.fail(mutableMapIterableName + " should be empty; actual size:<" + Iterate.sizeOf(actualMutableMapIterable) + '>');
}
if (actualMutableMapIterable.notEmpty())
{
Assert.fail(mutableMapIterableName + " should be empty; actual size:<" + Iterate.sizeOf(actualMutableMapIterable) + '>');
}
if (actualMutableMapIterable.size() != 0)
{
Assert.fail(mutableMapIterableName + " should be empty; actual size:<" + actualMutableMapIterable.size() + '>');
}
if (actualMutableMapIterable.keySet().size() != 0)
{
Assert.fail(mutableMapIterableName + " should be empty; actual size:<" + actualMutableMapIterable.keySet().size() + '>');
}
if (actualMutableMapIterable.values().size() != 0)
{
Assert.fail(mutableMapIterableName + " should be empty; actual size:<" + actualMutableMapIterable.values().size() + '>');
}
if (actualMutableMapIterable.entrySet().size() != 0)
{
Assert.fail(mutableMapIterableName + " should be empty; actual size:<" + actualMutableMapIterable.entrySet().size() + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link PrimitiveIterable} is empty.
*/
public static void assertEmpty(PrimitiveIterable primitiveIterable)
{
try
{
Verify.assertEmpty("primitiveIterable", primitiveIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link PrimitiveIterable} is empty.
*/
public static void assertEmpty(String iterableName, PrimitiveIterable primitiveIterable)
{
try
{
Verify.assertObjectNotNull(iterableName, primitiveIterable);
if (primitiveIterable.notEmpty())
{
Assert.fail(iterableName + " should be empty; actual size:<" + primitiveIterable.size() + '>');
}
if (!primitiveIterable.isEmpty())
{
Assert.fail(iterableName + " should be empty; actual size:<" + primitiveIterable.size() + '>');
}
if (primitiveIterable.size() != 0)
{
Assert.fail(iterableName + " should be empty; actual size:<" + primitiveIterable.size() + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Iterable} is empty.
*/
public static void assertIterableEmpty(Iterable> iterable)
{
try
{
Verify.assertIterableEmpty("iterable", iterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Iterable} is empty.
*/
public static void assertIterableEmpty(String iterableName, Iterable> iterable)
{
try
{
Verify.assertObjectNotNull(iterableName, iterable);
if (Iterate.notEmpty(iterable))
{
Assert.fail(iterableName + " should be empty; actual size:<" + Iterate.sizeOf(iterable) + '>');
}
if (!Iterate.isEmpty(iterable))
{
Assert.fail(iterableName + " should be empty; actual size:<" + Iterate.sizeOf(iterable) + '>');
}
if (Iterate.sizeOf(iterable) != 0)
{
Assert.fail(iterableName + " should be empty; actual size:<" + Iterate.sizeOf(iterable) + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given object is an instanceof expectedClassType.
*/
public static void assertInstanceOf(Class> expectedClassType, Object actualObject)
{
try
{
Verify.assertInstanceOf(actualObject.getClass().getName(), expectedClassType, actualObject);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given object is an instanceof expectedClassType.
*/
public static void assertInstanceOf(String objectName, Class> expectedClassType, Object actualObject)
{
try
{
if (!expectedClassType.isInstance(actualObject))
{
Assert.fail(objectName + " is not an instance of " + expectedClassType.getName());
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given object is not an instanceof expectedClassType.
*/
public static void assertNotInstanceOf(Class> expectedClassType, Object actualObject)
{
try
{
Verify.assertNotInstanceOf(actualObject.getClass().getName(), expectedClassType, actualObject);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given object is not an instanceof expectedClassType.
*/
public static void assertNotInstanceOf(String objectName, Class> expectedClassType, Object actualObject)
{
try
{
if (expectedClassType.isInstance(actualObject))
{
Assert.fail(objectName + " is an instance of " + expectedClassType.getName());
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Map} is empty.
*/
public static void assertEmpty(Map, ?> actualMap)
{
try
{
Verify.assertEmpty("map", actualMap);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Multimap} is empty.
*/
public static void assertEmpty(Multimap, ?> actualMultimap)
{
try
{
Verify.assertEmpty("multimap", actualMultimap);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Multimap} is empty.
*/
public static void assertEmpty(String multimapName, Multimap, ?> actualMultimap)
{
try
{
Verify.assertObjectNotNull(multimapName, actualMultimap);
if (actualMultimap.notEmpty())
{
Assert.fail(multimapName + " should be empty; actual size:<" + actualMultimap.size() + '>');
}
if (!actualMultimap.isEmpty())
{
Assert.fail(multimapName + " should be empty; actual size:<" + actualMultimap.size() + '>');
}
if (actualMultimap.size() != 0)
{
Assert.fail(multimapName + " should be empty; actual size:<" + actualMultimap.size() + '>');
}
if (actualMultimap.sizeDistinct() != 0)
{
Assert.fail(multimapName + " should be empty; actual size:<" + actualMultimap.size() + '>');
}
if (actualMultimap.keyBag().size() != 0)
{
Assert.fail(multimapName + " should be empty; actual size:<" + actualMultimap.keyBag().size() + '>');
}
if (actualMultimap.keysView().size() != 0)
{
Assert.fail(multimapName + " should be empty; actual size:<" + actualMultimap.keysView().size() + '>');
}
if (actualMultimap.valuesView().size() != 0)
{
Assert.fail(multimapName + " should be empty; actual size:<" + actualMultimap.valuesView().size() + '>');
}
if (actualMultimap.keyValuePairsView().size() != 0)
{
Assert.fail(multimapName + " should be empty; actual size:<" + actualMultimap.keyValuePairsView().size() + '>');
}
if (actualMultimap.keyMultiValuePairsView().size() != 0)
{
Assert.fail(multimapName + " should be empty; actual size:<" + actualMultimap.keyMultiValuePairsView().size() + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Map} is empty.
*/
public static void assertEmpty(String mapName, Map, ?> actualMap)
{
try
{
Verify.assertObjectNotNull(mapName, actualMap);
if (!actualMap.isEmpty())
{
Assert.fail(mapName + " should be empty; actual size:<" + actualMap.size() + '>');
}
if (actualMap.size() != 0)
{
Assert.fail(mapName + " should be empty; actual size:<" + actualMap.size() + '>');
}
if (actualMap.keySet().size() != 0)
{
Assert.fail(mapName + " should be empty; actual size:<" + actualMap.keySet().size() + '>');
}
if (actualMap.values().size() != 0)
{
Assert.fail(mapName + " should be empty; actual size:<" + actualMap.values().size() + '>');
}
if (actualMap.entrySet().size() != 0)
{
Assert.fail(mapName + " should be empty; actual size:<" + actualMap.entrySet().size() + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Iterable} is not empty.
*/
public static void assertNotEmpty(Iterable> actualIterable)
{
try
{
Verify.assertNotEmpty("iterable", actualIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Iterable} is not empty.
*/
public static void assertNotEmpty(String iterableName, Iterable> actualIterable)
{
try
{
Verify.assertObjectNotNull(iterableName, actualIterable);
Assert.assertFalse(iterableName + " should be non-empty, but was empty", Iterate.isEmpty(actualIterable));
Assert.assertTrue(iterableName + " should be non-empty, but was empty", Iterate.notEmpty(actualIterable));
Assert.assertNotEquals(iterableName + " should be non-empty, but was empty", 0, Iterate.sizeOf(actualIterable));
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link MutableMapIterable} is not empty.
*/
public static void assertNotEmpty(MutableMapIterable, ?> actualMutableMapIterable)
{
try
{
Verify.assertNotEmpty("mutableMapIterable", actualMutableMapIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link MutableMapIterable} is not empty.
*/
public static void assertNotEmpty(String mutableMapIterableName, MutableMapIterable, ?> actualMutableMapIterable)
{
try
{
Verify.assertObjectNotNull(mutableMapIterableName, actualMutableMapIterable);
Assert.assertFalse(mutableMapIterableName + " should be non-empty, but was empty", Iterate.isEmpty(actualMutableMapIterable));
Assert.assertTrue(mutableMapIterableName + " should be non-empty, but was empty", Iterate.notEmpty(actualMutableMapIterable));
Assert.assertTrue(mutableMapIterableName + " should be non-empty, but was empty", actualMutableMapIterable.notEmpty());
Assert.assertNotEquals(mutableMapIterableName + " should be non-empty, but was empty", 0, actualMutableMapIterable.size());
Assert.assertNotEquals(mutableMapIterableName + " should be non-empty, but was empty", 0, actualMutableMapIterable.keySet().size());
Assert.assertNotEquals(mutableMapIterableName + " should be non-empty, but was empty", 0, actualMutableMapIterable.values().size());
Assert.assertNotEquals(mutableMapIterableName + " should be non-empty, but was empty", 0, actualMutableMapIterable.entrySet().size());
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link PrimitiveIterable} is not empty.
*/
public static void assertNotEmpty(PrimitiveIterable primitiveIterable)
{
try
{
Verify.assertNotEmpty("primitiveIterable", primitiveIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link PrimitiveIterable} is not empty.
*/
public static void assertNotEmpty(String iterableName, PrimitiveIterable primitiveIterable)
{
try
{
Verify.assertObjectNotNull(iterableName, primitiveIterable);
Assert.assertFalse(iterableName + " should be non-empty, but was empty", primitiveIterable.isEmpty());
Assert.assertTrue(iterableName + " should be non-empty, but was empty", primitiveIterable.notEmpty());
Assert.assertNotEquals(iterableName + " should be non-empty, but was empty", 0, primitiveIterable.size());
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Iterable} is not empty.
*/
public static void assertIterableNotEmpty(Iterable> iterable)
{
try
{
Verify.assertIterableNotEmpty("iterable", iterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Iterable} is not empty.
*/
public static void assertIterableNotEmpty(String iterableName, Iterable> iterable)
{
try
{
Verify.assertObjectNotNull(iterableName, iterable);
Assert.assertFalse(iterableName + " should be non-empty, but was empty", Iterate.isEmpty(iterable));
Assert.assertTrue(iterableName + " should be non-empty, but was empty", Iterate.notEmpty(iterable));
Assert.assertNotEquals(iterableName + " should be non-empty, but was empty", 0, Iterate.sizeOf(iterable));
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Map} is not empty.
*/
public static void assertNotEmpty(Map, ?> actualMap)
{
try
{
Verify.assertNotEmpty("map", actualMap);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Map} is not empty.
*/
public static void assertNotEmpty(String mapName, Map, ?> actualMap)
{
try
{
Verify.assertObjectNotNull(mapName, actualMap);
Assert.assertFalse(mapName + " should be non-empty, but was empty", actualMap.isEmpty());
Assert.assertNotEquals(mapName + " should be non-empty, but was empty", 0, actualMap.size());
Assert.assertNotEquals(mapName + " should be non-empty, but was empty", 0, actualMap.keySet().size());
Assert.assertNotEquals(mapName + " should be non-empty, but was empty", 0, actualMap.values().size());
Assert.assertNotEquals(mapName + " should be non-empty, but was empty", 0, actualMap.entrySet().size());
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Multimap} is not empty.
*/
public static void assertNotEmpty(Multimap, ?> actualMultimap)
{
try
{
Verify.assertNotEmpty("multimap", actualMultimap);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Multimap} is not empty.
*/
public static void assertNotEmpty(String multimapName, Multimap, ?> actualMultimap)
{
try
{
Verify.assertObjectNotNull(multimapName, actualMultimap);
Assert.assertTrue(multimapName + " should be non-empty, but was empty", actualMultimap.notEmpty());
Assert.assertFalse(multimapName + " should be non-empty, but was empty", actualMultimap.isEmpty());
Assert.assertNotEquals(multimapName + " should be non-empty, but was empty", 0, actualMultimap.size());
Assert.assertNotEquals(multimapName + " should be non-empty, but was empty", 0, actualMultimap.sizeDistinct());
Assert.assertNotEquals(multimapName + " should be non-empty, but was empty", 0, actualMultimap.keyBag().size());
Assert.assertNotEquals(multimapName + " should be non-empty, but was empty", 0, actualMultimap.keysView().size());
Assert.assertNotEquals(multimapName + " should be non-empty, but was empty", 0, actualMultimap.valuesView().size());
Assert.assertNotEquals(multimapName + " should be non-empty, but was empty", 0, actualMultimap.keyValuePairsView().size());
Assert.assertNotEquals(multimapName + " should be non-empty, but was empty", 0, actualMultimap.keyMultiValuePairsView().size());
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertNotEmpty(String itemsName, T[] items)
{
try
{
Verify.assertObjectNotNull(itemsName, items);
Verify.assertNotEquals(itemsName, 0, items.length);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertNotEmpty(T[] items)
{
try
{
Verify.assertNotEmpty("items", items);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given array.
*/
public static void assertSize(int expectedSize, Object[] actualArray)
{
try
{
Verify.assertSize("array", expectedSize, actualArray);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given array.
*/
public static void assertSize(String arrayName, int expectedSize, Object[] actualArray)
{
try
{
Assert.assertNotNull(arrayName + " should not be null", actualArray);
int actualSize = actualArray.length;
if (actualSize != expectedSize)
{
Assert.fail("Incorrect size for "
+ arrayName
+ "; expected:<"
+ expectedSize
+ "> but was:<"
+ actualSize
+ '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link Iterable}.
*/
public static void assertSize(int expectedSize, Iterable> actualIterable)
{
try
{
Verify.assertSize("iterable", expectedSize, actualIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link Iterable}.
*/
public static void assertSize(
String iterableName,
int expectedSize,
Iterable> actualIterable)
{
try
{
Verify.assertObjectNotNull(iterableName, actualIterable);
int actualSize = Iterate.sizeOf(actualIterable);
if (actualSize != expectedSize)
{
Assert.fail("Incorrect size for "
+ iterableName
+ "; expected:<"
+ expectedSize
+ "> but was:<"
+ actualSize
+ '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link PrimitiveIterable}.
*/
public static void assertSize(int expectedSize, PrimitiveIterable primitiveIterable)
{
try
{
Verify.assertSize("primitiveIterable", expectedSize, primitiveIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link PrimitiveIterable}.
*/
public static void assertSize(
String primitiveIterableName,
int expectedSize,
PrimitiveIterable actualPrimitiveIterable)
{
try
{
Verify.assertObjectNotNull(primitiveIterableName, actualPrimitiveIterable);
int actualSize = actualPrimitiveIterable.size();
if (actualSize != expectedSize)
{
Assert.fail("Incorrect size for "
+ primitiveIterableName
+ "; expected:<"
+ expectedSize
+ "> but was:<"
+ actualSize
+ '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link Iterable}.
*/
public static void assertIterableSize(int expectedSize, Iterable> actualIterable)
{
try
{
Verify.assertIterableSize("iterable", expectedSize, actualIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link Iterable}.
*/
public static void assertIterableSize(
String iterableName,
int expectedSize,
Iterable> actualIterable)
{
try
{
Verify.assertObjectNotNull(iterableName, actualIterable);
int actualSize = Iterate.sizeOf(actualIterable);
if (actualSize != expectedSize)
{
Assert.fail("Incorrect size for "
+ iterableName
+ "; expected:<"
+ expectedSize
+ "> but was:<"
+ actualSize
+ '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link Map}.
*/
public static void assertSize(String mapName, int expectedSize, Map, ?> actualMap)
{
try
{
Verify.assertSize(mapName, expectedSize, actualMap.keySet());
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link Map}.
*/
public static void assertSize(int expectedSize, Map, ?> actualMap)
{
try
{
Verify.assertSize("map", expectedSize, actualMap);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link Multimap}.
*/
public static void assertSize(int expectedSize, Multimap, ?> actualMultimap)
{
try
{
Verify.assertSize("multimap", expectedSize, actualMultimap);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link Multimap}.
*/
public static void assertSize(String multimapName, int expectedSize, Multimap, ?> actualMultimap)
{
try
{
int actualSize = actualMultimap.size();
if (actualSize != expectedSize)
{
Assert.fail("Incorrect size for "
+ multimapName
+ "; expected:<"
+ expectedSize
+ "> but was:<"
+ actualSize
+ '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link MutableMapIterable}.
*/
public static void assertSize(int expectedSize, MutableMapIterable, ?> mutableMapIterable)
{
try
{
Verify.assertSize("map", expectedSize, mutableMapIterable);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link MutableMapIterable}.
*/
public static void assertSize(String mapName, int expectedSize, MutableMapIterable, ?> mutableMapIterable)
{
try
{
int actualSize = mutableMapIterable.size();
if (actualSize != expectedSize)
{
Assert.fail("Incorrect size for " + mapName + "; expected:<" + expectedSize + "> but was:<" + actualSize + '>');
}
int keySetSize = mutableMapIterable.keySet().size();
if (keySetSize != expectedSize)
{
Assert.fail("Incorrect size for " + mapName + ".keySet(); expected:<" + expectedSize + "> but was:<" + actualSize + '>');
}
int valuesSize = mutableMapIterable.values().size();
if (valuesSize != expectedSize)
{
Assert.fail("Incorrect size for " + mapName + ".values(); expected:<" + expectedSize + "> but was:<" + actualSize + '>');
}
int entrySetSize = mutableMapIterable.entrySet().size();
if (entrySetSize != expectedSize)
{
Assert.fail("Incorrect size for " + mapName + ".entrySet(); expected:<" + expectedSize + "> but was:<" + actualSize + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link ImmutableSet}.
*/
public static void assertSize(int expectedSize, ImmutableSet> actualImmutableSet)
{
try
{
Verify.assertSize("immutable set", expectedSize, actualImmutableSet);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert the size of the given {@link ImmutableSet}.
*/
public static void assertSize(String immutableSetName, int expectedSize, ImmutableSet> actualImmutableSet)
{
try
{
int actualSize = actualImmutableSet.size();
if (actualSize != expectedSize)
{
Assert.fail("Incorrect size for "
+ immutableSetName
+ "; expected:<"
+ expectedSize
+ "> but was:<"
+ actualSize
+ '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@code stringToFind} is contained within the {@code stringToSearch}.
*/
public static void assertContains(String stringToFind, String stringToSearch)
{
try
{
Verify.assertContains("string", stringToFind, stringToSearch);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@code unexpectedString} is not contained within the {@code stringToSearch}.
*/
public static void assertNotContains(String unexpectedString, String stringToSearch)
{
try
{
Verify.assertNotContains("string", unexpectedString, stringToSearch);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@code stringToFind} is contained within the {@code stringToSearch}.
*/
public static void assertContains(String stringName, String stringToFind, String stringToSearch)
{
try
{
Assert.assertNotNull("stringToFind should not be null", stringToFind);
Assert.assertNotNull("stringToSearch should not be null", stringToSearch);
if (!stringToSearch.contains(stringToFind))
{
Assert.fail(stringName
+ " did not contain stringToFind:<"
+ stringToFind
+ "> in stringToSearch:<"
+ stringToSearch
+ '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@code unexpectedString} is not contained within the {@code stringToSearch}.
*/
public static void assertNotContains(String stringName, String unexpectedString, String stringToSearch)
{
try
{
Assert.assertNotNull("unexpectedString should not be null", unexpectedString);
Assert.assertNotNull("stringToSearch should not be null", stringToSearch);
if (stringToSearch.contains(unexpectedString))
{
Assert.fail(stringName
+ " contains unexpectedString:<"
+ unexpectedString
+ "> in stringToSearch:<"
+ stringToSearch
+ '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertCount(
int expectedCount,
Iterable iterable,
Predicate super T> predicate)
{
Assert.assertEquals(expectedCount, Iterate.count(iterable, predicate));
}
public static void assertAllSatisfy(Iterable iterable, Predicate super T> predicate)
{
try
{
Verify.assertAllSatisfy("The following items failed to satisfy the condition", iterable, predicate);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertAllSatisfy(Map map, Predicate super V> predicate)
{
try
{
Verify.assertAllSatisfy(map.values(), predicate);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertAllSatisfy(String message, Iterable iterable, Predicate super T> predicate)
{
try
{
MutableList unnacceptable = Iterate.reject(iterable, predicate, Lists.mutable.of());
if (unnacceptable.notEmpty())
{
Assert.fail(message + " <" + unnacceptable + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertAnySatisfy(Iterable iterable, Predicate super T> predicate)
{
try
{
Verify.assertAnySatisfy("No items satisfied the condition", iterable, predicate);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertAnySatisfy(Map map, Predicate super V> predicate)
{
try
{
Verify.assertAnySatisfy(map.values(), predicate);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertAnySatisfy(String message, Iterable iterable, Predicate super T> predicate)
{
try
{
Assert.assertTrue(message, Predicates.anySatisfy(predicate).accept(iterable));
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertNoneSatisfy(Iterable iterable, Predicate super T> predicate)
{
try
{
Verify.assertNoneSatisfy("The following items satisfied the condition", iterable, predicate);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertNoneSatisfy(Map map, Predicate super V> predicate)
{
try
{
Verify.assertNoneSatisfy(map.values(), predicate);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertNoneSatisfy(String message, Iterable iterable, Predicate super T> predicate)
{
try
{
MutableList unnacceptable = Iterate.select(iterable, predicate, Lists.mutable.empty());
if (unnacceptable.notEmpty())
{
Assert.fail(message + " <" + unnacceptable + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Map} contains all of the given keys and values.
*/
public static void assertContainsAllKeyValues(Map, ?> actualMap, Object... keyValues)
{
try
{
Verify.assertContainsAllKeyValues("map", actualMap, keyValues);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Map} contains all of the given keys and values.
*/
public static void assertContainsAllKeyValues(
String mapName,
Map, ?> actualMap,
Object... expectedKeyValues)
{
try
{
Verify.assertNotEmpty("Expected keys/values in assertion", expectedKeyValues);
if (expectedKeyValues.length % 2 != 0)
{
Assert.fail("Odd number of keys and values (every key must have a value)");
}
Verify.assertObjectNotNull(mapName, actualMap);
Verify.assertMapContainsKeys(mapName, actualMap, expectedKeyValues);
Verify.assertMapContainsValues(mapName, actualMap, expectedKeyValues);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link MapIterable} contains all of the given keys and values.
*/
public static void assertContainsAllKeyValues(MapIterable, ?> mapIterable, Object... keyValues)
{
try
{
Verify.assertContainsAllKeyValues("map", mapIterable, keyValues);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link MapIterable} contains all of the given keys and values.
*/
public static void assertContainsAllKeyValues(
String mapIterableName,
MapIterable, ?> mapIterable,
Object... expectedKeyValues)
{
try
{
Verify.assertNotEmpty("Expected keys/values in assertion", expectedKeyValues);
if (expectedKeyValues.length % 2 != 0)
{
Assert.fail("Odd number of keys and values (every key must have a value)");
}
Verify.assertObjectNotNull(mapIterableName, mapIterable);
Verify.assertMapContainsKeys(mapIterableName, mapIterable, expectedKeyValues);
Verify.assertMapContainsValues(mapIterableName, mapIterable, expectedKeyValues);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link MutableMapIterable} contains all of the given keys and values.
*/
public static void assertContainsAllKeyValues(MutableMapIterable, ?> mutableMapIterable, Object... keyValues)
{
try
{
Verify.assertContainsAllKeyValues("map", mutableMapIterable, keyValues);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link MutableMapIterable} contains all of the given keys and values.
*/
public static void assertContainsAllKeyValues(
String mutableMapIterableName,
MutableMapIterable, ?> mutableMapIterable,
Object... expectedKeyValues)
{
try
{
Verify.assertNotEmpty("Expected keys/values in assertion", expectedKeyValues);
if (expectedKeyValues.length % 2 != 0)
{
Assert.fail("Odd number of keys and values (every key must have a value)");
}
Verify.assertObjectNotNull(mutableMapIterableName, mutableMapIterable);
Verify.assertMapContainsKeys(mutableMapIterableName, mutableMapIterable, expectedKeyValues);
Verify.assertMapContainsValues(mutableMapIterableName, mutableMapIterable, expectedKeyValues);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link ImmutableMapIterable} contains all of the given keys and values.
*/
public static void assertContainsAllKeyValues(ImmutableMapIterable, ?> immutableMapIterable, Object... keyValues)
{
try
{
Verify.assertContainsAllKeyValues("map", immutableMapIterable, keyValues);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link ImmutableMapIterable} contains all of the given keys and values.
*/
public static void assertContainsAllKeyValues(
String immutableMapIterableName,
ImmutableMapIterable, ?> immutableMapIterable,
Object... expectedKeyValues)
{
try
{
Verify.assertNotEmpty("Expected keys/values in assertion", expectedKeyValues);
if (expectedKeyValues.length % 2 != 0)
{
Assert.fail("Odd number of keys and values (every key must have a value)");
}
Verify.assertObjectNotNull(immutableMapIterableName, immutableMapIterable);
Verify.assertMapContainsKeys(immutableMapIterableName, immutableMapIterable, expectedKeyValues);
Verify.assertMapContainsValues(immutableMapIterableName, immutableMapIterable, expectedKeyValues);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void denyContainsAny(Collection> actualCollection, Object... items)
{
try
{
Verify.denyContainsAny("collection", actualCollection, items);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertContainsNone(Collection> actualCollection, Object... items)
{
try
{
Verify.denyContainsAny("collection", actualCollection, items);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Collection} contains the given item.
*/
public static void assertContains(Object expectedItem, Collection> actualCollection)
{
try
{
Verify.assertContains("collection", expectedItem, actualCollection);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link Collection} contains the given item.
*/
public static void assertContains(
String collectionName,
Object expectedItem,
Collection> actualCollection)
{
try
{
Verify.assertObjectNotNull(collectionName, actualCollection);
if (!actualCollection.contains(expectedItem))
{
Assert.fail(collectionName + " did not contain expectedItem:<" + expectedItem + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link ImmutableCollection} contains the given item.
*/
public static void assertContains(Object expectedItem, ImmutableCollection> actualImmutableCollection)
{
try
{
Verify.assertContains("ImmutableCollection", expectedItem, actualImmutableCollection);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
/**
* Assert that the given {@link ImmutableCollection} contains the given item.
*/
public static void assertContains(
String immutableCollectionName,
Object expectedItem,
ImmutableCollection> actualImmutableCollection)
{
try
{
Verify.assertObjectNotNull(immutableCollectionName, actualImmutableCollection);
if (!actualImmutableCollection.contains(expectedItem))
{
Assert.fail(immutableCollectionName + " did not contain expectedItem:<" + expectedItem + '>');
}
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertContainsAll(
Iterable> iterable,
Object... items)
{
try
{
Verify.assertContainsAll("iterable", iterable, items);
}
catch (AssertionError e)
{
Verify.throwMangledException(e);
}
}
public static void assertContainsAll(
String collectionName,
Iterable> iterable,
Object... items)
{
try
{
Verify.assertObjectNotNull(collectionName, iterable);
Verify.assertNotEmpty("Expected items in assertion", items);
Predicate