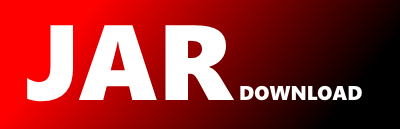
org.eclipse.collections.impl.bimap.mutable.SynchronizedBiMap Maven / Gradle / Ivy
/*
* Copyright (c) 2017 Bhavana Hindupur and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.bimap.mutable;
import java.io.Serializable;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.bimap.ImmutableBiMap;
import org.eclipse.collections.api.bimap.MutableBiMap;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.block.procedure.Procedure;
import org.eclipse.collections.api.multimap.set.MutableSetMultimap;
import org.eclipse.collections.api.ordered.OrderedIterable;
import org.eclipse.collections.api.partition.set.PartitionMutableSet;
import org.eclipse.collections.api.set.MutableSet;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.impl.collection.mutable.SynchronizedMutableCollection;
import org.eclipse.collections.impl.list.fixed.ArrayAdapter;
import org.eclipse.collections.impl.map.AbstractSynchronizedMapIterable;
import org.eclipse.collections.impl.map.mutable.SynchronizedBiMapSerializationProxy;
import org.eclipse.collections.impl.set.mutable.SynchronizedMutableSet;
import org.eclipse.collections.impl.utility.LazyIterate;
public class SynchronizedBiMap extends AbstractSynchronizedMapIterable implements MutableBiMap, Serializable
{
private static final long serialVersionUID = 1L;
protected SynchronizedBiMap(MutableBiMap delegate)
{
super(delegate);
}
protected SynchronizedBiMap(MutableBiMap delegate, Object lock)
{
super(delegate, lock);
}
/**
* This method will take a MutableBiMap and wrap it directly in a SynchronizedBiMap.
*/
public static SynchronizedBiMap of(MutableBiMap map)
{
if (map == null)
{
throw new IllegalArgumentException("cannot create a SynchronizedBiMap for null");
}
return new SynchronizedBiMap<>(map);
}
@Override
protected MutableBiMap getDelegate()
{
return (MutableBiMap) super.getDelegate();
}
@Override
public V forcePut(K key, V value)
{
synchronized (this.lock)
{
return this.getDelegate().forcePut(key, value);
}
}
@Override
public MutableBiMap asSynchronized()
{
return this;
}
@Override
public MutableBiMap asUnmodifiable()
{
throw new UnsupportedOperationException(this.getClass().getSimpleName() + ".asUnmodifiable() not implemented yet");
}
@Override
public MutableBiMap clone()
{
synchronized (this.lock)
{
return SynchronizedBiMap.of(this.getDelegate().clone());
}
}
@Override
public MutableBiMap tap(Procedure super V> procedure)
{
return (MutableBiMap) super.tap(procedure);
}
@Override
public MutableBiMap collect(Function2 super K, ? super V, Pair> function)
{
synchronized (this.lock)
{
return this.getDelegate().collect(function);
}
}
@Override
public MutableBiMap collectValues(Function2 super K, ? super V, ? extends R> function)
{
synchronized (this.lock)
{
return this.getDelegate().collectValues(function);
}
}
@Override
public MutableSet select(Predicate super V> predicate)
{
return (MutableSet) super.select(predicate);
}
@Override
public MutableBiMap select(Predicate2 super K, ? super V> predicate)
{
synchronized (this.lock)
{
return this.getDelegate().select(predicate);
}
}
@Override
public MutableSet selectWith(Predicate2 super V, ? super P> predicate, P parameter)
{
return (MutableSet) super.selectWith(predicate, parameter);
}
@Override
public MutableSet selectInstancesOf(Class clazz)
{
return (MutableSet) super.selectInstancesOf(clazz);
}
@Override
public MutableSet reject(Predicate super V> predicate)
{
return (MutableSet) super.reject(predicate);
}
@Override
public MutableBiMap reject(Predicate2 super K, ? super V> predicate)
{
synchronized (this.lock)
{
return this.getDelegate().reject(predicate);
}
}
@Override
public MutableSet rejectWith(Predicate2 super V, ? super P> predicate, P parameter)
{
return (MutableSet) super.rejectWith(predicate, parameter);
}
@Override
public PartitionMutableSet partition(Predicate super V> predicate)
{
return (PartitionMutableSet) super.partition(predicate);
}
@Override
public PartitionMutableSet partitionWith(Predicate2 super V, ? super P> predicate, P parameter)
{
return (PartitionMutableSet) super.partitionWith(predicate, parameter);
}
@Override
public MutableSetMultimap groupBy(Function super V, ? extends V1> function)
{
synchronized (this.lock)
{
return this.getDelegate().groupBy(function);
}
}
@Override
public MutableSetMultimap groupByEach(Function super V, ? extends Iterable> function)
{
synchronized (this.lock)
{
return this.getDelegate().groupByEach(function);
}
}
@Override
public MutableSetMultimap flip()
{
synchronized (this.lock)
{
return this.getDelegate().flip();
}
}
@Override
public MutableBiMap newEmpty()
{
synchronized (this.lock)
{
return this.getDelegate().newEmpty();
}
}
@Override
public MutableBiMap inverse()
{
synchronized (this.lock)
{
return new SynchronizedBiMap<>(this.getDelegate().inverse(), this.lock);
}
}
@Override
public MutableBiMap flipUniqueValues()
{
synchronized (this.lock)
{
return this.getDelegate().flipUniqueValues();
}
}
@Override
public RichIterable keysView()
{
return LazyIterate.adapt(this.keySet());
}
@Override
public RichIterable valuesView()
{
return LazyIterate.adapt(this.values());
}
@Override
public ImmutableBiMap toImmutable()
{
synchronized (this.lock)
{
return this.getDelegate().toImmutable();
}
}
/**
* @deprecated in 8.0. Use {@link OrderedIterable#zipWithIndex()} instead.
*/
@Override
@Deprecated
public MutableSet> zipWithIndex()
{
return (MutableSet>) super.zipWithIndex();
}
@Override
public MutableBiMap groupByUniqueKey(Function super V, ? extends VV> function)
{
return (MutableBiMap) super.groupByUniqueKey(function);
}
/**
* @deprecated in 8.0. Use {@link OrderedIterable#zip(Iterable)} instead.
*/
@Override
@Deprecated
public MutableSet> zip(Iterable that)
{
return (MutableSet>) super.zip(that);
}
@Override
public MutableBiMap withKeyValue(K key, V value)
{
synchronized (this.lock)
{
this.getDelegate().put(key, value);
return this;
}
}
@Override
public MutableBiMap withMap(Map extends K, ? extends V> map)
{
synchronized (this.lock)
{
this.putAll(map);
return this;
}
}
@Override
public MutableBiMap withAllKeyValues(Iterable extends Pair extends K, ? extends V>> keyValues)
{
synchronized (this.lock)
{
for (Pair extends K, ? extends V> keyValue : keyValues)
{
this.getDelegate().put(keyValue.getOne(), keyValue.getTwo());
}
return this;
}
}
@Override
public MutableBiMap withAllKeyValueArguments(Pair extends K, ? extends V>... keyValuePairs)
{
return this.withAllKeyValues(ArrayAdapter.adapt(keyValuePairs));
}
@Override
public MutableBiMap withoutKey(K key)
{
synchronized (this.lock)
{
this.getDelegate().remove(key);
return this;
}
}
@Override
public MutableBiMap withoutAllKeys(Iterable extends K> keys)
{
synchronized (this.lock)
{
for (K key : keys)
{
this.getDelegate().removeKey(key);
}
return this;
}
}
@Override
public Set keySet()
{
synchronized (this.lock)
{
return SynchronizedMutableSet.of(this.getDelegate().keySet(), this.lock);
}
}
@Override
public Collection values()
{
synchronized (this.lock)
{
return SynchronizedMutableCollection.of(this.getDelegate().values(), this.lock);
}
}
@Override
public Set> entrySet()
{
synchronized (this.lock)
{
return SynchronizedMutableSet.of(this.getDelegate().entrySet(), this.lock);
}
}
protected Object writeReplace()
{
return new SynchronizedBiMapSerializationProxy<>(this.getDelegate());
}
}