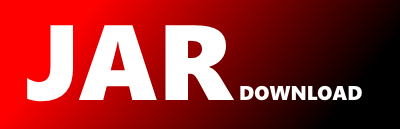
org.eclipse.collections.impl.collection.mutable.AbstractMultiReaderMutableCollection Maven / Gradle / Ivy
/*
* Copyright (c) 2020 Goldman Sachs and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.collection.mutable;
import java.util.Collection;
import java.util.Comparator;
import java.util.Iterator;
import java.util.Map;
import java.util.Optional;
import java.util.Spliterator;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.stream.Stream;
import org.eclipse.collections.api.BooleanIterable;
import org.eclipse.collections.api.ByteIterable;
import org.eclipse.collections.api.CharIterable;
import org.eclipse.collections.api.DoubleIterable;
import org.eclipse.collections.api.FloatIterable;
import org.eclipse.collections.api.IntIterable;
import org.eclipse.collections.api.LazyIterable;
import org.eclipse.collections.api.LongIterable;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.ShortIterable;
import org.eclipse.collections.api.bag.MutableBag;
import org.eclipse.collections.api.bag.sorted.MutableSortedBag;
import org.eclipse.collections.api.bimap.MutableBiMap;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function0;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.function.Function3;
import org.eclipse.collections.api.block.function.primitive.BooleanFunction;
import org.eclipse.collections.api.block.function.primitive.ByteFunction;
import org.eclipse.collections.api.block.function.primitive.CharFunction;
import org.eclipse.collections.api.block.function.primitive.DoubleFunction;
import org.eclipse.collections.api.block.function.primitive.DoubleObjectToDoubleFunction;
import org.eclipse.collections.api.block.function.primitive.FloatFunction;
import org.eclipse.collections.api.block.function.primitive.FloatObjectToFloatFunction;
import org.eclipse.collections.api.block.function.primitive.IntFunction;
import org.eclipse.collections.api.block.function.primitive.IntObjectToIntFunction;
import org.eclipse.collections.api.block.function.primitive.LongFunction;
import org.eclipse.collections.api.block.function.primitive.LongObjectToLongFunction;
import org.eclipse.collections.api.block.function.primitive.ShortFunction;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.block.procedure.Procedure;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.block.procedure.primitive.ObjectIntProcedure;
import org.eclipse.collections.api.collection.MutableCollection;
import org.eclipse.collections.api.collection.primitive.MutableBooleanCollection;
import org.eclipse.collections.api.collection.primitive.MutableByteCollection;
import org.eclipse.collections.api.collection.primitive.MutableCharCollection;
import org.eclipse.collections.api.collection.primitive.MutableDoubleCollection;
import org.eclipse.collections.api.collection.primitive.MutableFloatCollection;
import org.eclipse.collections.api.collection.primitive.MutableIntCollection;
import org.eclipse.collections.api.collection.primitive.MutableLongCollection;
import org.eclipse.collections.api.collection.primitive.MutableShortCollection;
import org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.api.map.MutableMap;
import org.eclipse.collections.api.map.MutableMapIterable;
import org.eclipse.collections.api.map.primitive.MutableObjectDoubleMap;
import org.eclipse.collections.api.map.primitive.MutableObjectLongMap;
import org.eclipse.collections.api.map.sorted.MutableSortedMap;
import org.eclipse.collections.api.multimap.MutableMultimap;
import org.eclipse.collections.api.set.MutableSet;
import org.eclipse.collections.api.set.sorted.MutableSortedSet;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.api.tuple.Twin;
import org.eclipse.collections.impl.block.factory.PrimitiveFunctions;
import org.eclipse.collections.impl.block.procedure.MutatingAggregationProcedure;
import org.eclipse.collections.impl.factory.primitive.ObjectDoubleMaps;
import org.eclipse.collections.impl.factory.primitive.ObjectLongMaps;
import org.eclipse.collections.impl.map.mutable.UnifiedMap;
/**
* AbstractMultiReaderMutableCollection is a common abstraction that provides thread-safe collection behaviors.
* Subclasses of this class must provide implementations of getDelegate() and getLock().
*/
@SuppressWarnings({"unused", "TransientFieldInNonSerializableClass"})
public abstract class AbstractMultiReaderMutableCollection implements MutableCollection
{
protected transient ReadWriteLock lock;
protected transient ReadWriteLockWrapper lockWrapper;
protected abstract MutableCollection getDelegate();
@Override
public boolean contains(Object item)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().contains(item);
}
}
@Override
public boolean containsAll(Collection> collection)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().containsAll(collection);
}
}
@Override
public boolean containsAllIterable(Iterable> source)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().containsAllIterable(source);
}
}
@Override
public boolean containsAllArguments(Object... elements)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().containsAllArguments(elements);
}
}
@Override
public boolean noneSatisfy(Predicate super T> predicate)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().noneSatisfy(predicate);
}
}
@Override
public boolean noneSatisfyWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().noneSatisfyWith(predicate, parameter);
}
}
@Override
public boolean allSatisfy(Predicate super T> predicate)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().allSatisfy(predicate);
}
}
@Override
public
boolean allSatisfyWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().allSatisfyWith(predicate, parameter);
}
}
@Override
public boolean anySatisfy(Predicate super T> predicate)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().anySatisfy(predicate);
}
}
@Override
public
boolean anySatisfyWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().anySatisfyWith(predicate, parameter);
}
}
@Override
public > R into(R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().into(target);
}
}
@Override
public MutableList toList()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toList();
}
}
@Override
public MutableMap toMap(
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toMap(keyFunction, valueFunction);
}
}
@Override
public > R toMap(
Function super T, ? extends K> keyFunction,
Function super T, ? extends V> valueFunction,
R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toMap(keyFunction, valueFunction, target);
}
}
@Override
public MutableSortedMap toSortedMap(
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedMap(keyFunction, valueFunction);
}
}
@Override
public MutableSortedMap toSortedMap(
Comparator super NK> comparator,
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedMap(comparator, keyFunction, valueFunction);
}
}
@Override
public , NK, NV> MutableSortedMap toSortedMapBy(
Function super NK, KK> sortBy,
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedMapBy(sortBy, keyFunction, valueFunction);
}
}
@Override
public MutableBiMap toBiMap(
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toBiMap(keyFunction, valueFunction);
}
}
@Override
public LazyIterable asLazy()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().asLazy();
}
}
@Override
public MutableSet toSet()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSet();
}
}
@Override
public MutableBag toBag()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toBag();
}
}
@Override
public MutableSortedBag toSortedBag()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedBag();
}
}
@Override
public MutableSortedBag toSortedBag(Comparator super T> comparator)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedBag(comparator);
}
}
@Override
public > MutableSortedBag toSortedBagBy(
Function super T, ? extends V> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedBagBy(function);
}
}
@Override
public MutableList toSortedList()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedList();
}
}
@Override
public MutableList toSortedList(Comparator super T> comparator)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedList(comparator);
}
}
@Override
public > MutableList toSortedListBy(
Function super T, ? extends V> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedListBy(function);
}
}
@Override
public MutableSortedSet toSortedSet()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedSet();
}
}
@Override
public MutableSortedSet toSortedSet(Comparator super T> comparator)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedSet(comparator);
}
}
@Override
public > MutableSortedSet toSortedSetBy(
Function super T, ? extends V> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toSortedSetBy(function);
}
}
@Override
public int count(Predicate super T> predicate)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().count(predicate);
}
}
@Override
public int countWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().countWith(predicate, parameter);
}
}
@Override
public T detect(Predicate super T> predicate)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().detect(predicate);
}
}
@Override
public
T detectWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().detectWith(predicate, parameter);
}
}
@Override
public Optional detectOptional(Predicate super T> predicate)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().detectOptional(predicate);
}
}
@Override
public Optional detectWithOptional(
Predicate2 super T, ? super P> predicate,
P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().detectWithOptional(predicate, parameter);
}
}
@Override
public T detectIfNone(
Predicate super T> predicate,
Function0 extends T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().detectIfNone(predicate, function);
}
}
@Override
public T detectWithIfNone(
Predicate2 super T, ? super P> predicate,
P parameter,
Function0 extends T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().detectWithIfNone(predicate, parameter, function);
}
}
@Override
public T min(Comparator super T> comparator)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().min(comparator);
}
}
@Override
public T max(Comparator super T> comparator)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().max(comparator);
}
}
@Override
public Optional minOptional(Comparator super T> comparator)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().minOptional(comparator);
}
}
@Override
public Optional maxOptional(Comparator super T> comparator)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().maxOptional(comparator);
}
}
@Override
public T min()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().min();
}
}
@Override
public T max()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().max();
}
}
@Override
public Optional minOptional()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().minOptional();
}
}
@Override
public Optional maxOptional()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().maxOptional();
}
}
@Override
public > T minBy(Function super T, ? extends V> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().minBy(function);
}
}
@Override
public > T maxBy(Function super T, ? extends V> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().maxBy(function);
}
}
@Override
public > Optional minByOptional(Function super T, ? extends V> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().minByOptional(function);
}
}
@Override
public > Optional maxByOptional(Function super T, ? extends V> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().maxByOptional(function);
}
}
@Override
public T getFirst()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().getFirst();
}
}
@Override
public T getLast()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().getLast();
}
}
@Override
public T getOnly()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().getOnly();
}
}
@Override
public boolean notEmpty()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().notEmpty();
}
}
@Override
public Twin> selectAndRejectWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().selectAndRejectWith(predicate, parameter);
}
}
@Override
public > R collect(
Function super T, ? extends V> function,
R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collect(function, target);
}
}
@Override
public R collectBoolean(BooleanFunction super T> booleanFunction, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectBoolean(booleanFunction, target);
}
}
@Override
public R flatCollectBoolean(
Function super T, ? extends BooleanIterable> function, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().flatCollectBoolean(function, target);
}
}
@Override
public R collectByte(ByteFunction super T> byteFunction, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectByte(byteFunction, target);
}
}
@Override
public R flatCollectByte(
Function super T, ? extends ByteIterable> function, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().flatCollectByte(function, target);
}
}
@Override
public R collectChar(CharFunction super T> charFunction, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectChar(charFunction, target);
}
}
@Override
public R flatCollectChar(
Function super T, ? extends CharIterable> function, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().flatCollectChar(function, target);
}
}
@Override
public R collectDouble(DoubleFunction super T> doubleFunction, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectDouble(doubleFunction, target);
}
}
@Override
public R flatCollectDouble(
Function super T, ? extends DoubleIterable> function, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().flatCollectDouble(function, target);
}
}
@Override
public R collectFloat(FloatFunction super T> floatFunction, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectFloat(floatFunction, target);
}
}
@Override
public R flatCollectFloat(
Function super T, ? extends FloatIterable> function, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().flatCollectFloat(function, target);
}
}
@Override
public R collectInt(IntFunction super T> intFunction, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectInt(intFunction, target);
}
}
@Override
public R flatCollectInt(
Function super T, ? extends IntIterable> function, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().flatCollectInt(function, target);
}
}
@Override
public R collectLong(LongFunction super T> longFunction, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectLong(longFunction, target);
}
}
@Override
public R flatCollectLong(
Function super T, ? extends LongIterable> function, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().flatCollectLong(function, target);
}
}
@Override
public R collectShort(ShortFunction super T> shortFunction, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectShort(shortFunction, target);
}
}
@Override
public R flatCollectShort(
Function super T, ? extends ShortIterable> function, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().flatCollectShort(function, target);
}
}
@Override
public > R flatCollect(
Function super T, ? extends Iterable> function,
R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().flatCollect(function, target);
}
}
@Override
public > R collectIf(
Predicate super T> predicate,
Function super T, ? extends V> function,
R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectIf(predicate, function, target);
}
}
@Override
public > R collectWith(
Function2 super T, ? super P, ? extends V> function,
P parameter,
R targetCollection)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().collectWith(function, parameter, targetCollection);
}
}
@Override
public
> R selectWith(
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().selectWith(predicate, parameter, targetCollection);
}
}
@Override
public > R reject(
Predicate super T> predicate,
R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().reject(predicate, target);
}
}
@Override
public > R rejectWith(
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().rejectWith(predicate, parameter, targetCollection);
}
}
@Override
public > R select(Predicate super T> predicate, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().select(predicate, target);
}
}
@Override
public IV injectInto(
IV injectedValue,
Function2 super IV, ? super T, ? extends IV> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().injectInto(injectedValue, function);
}
}
@Override
public int injectInto(int injectedValue, IntObjectToIntFunction super T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().injectInto(injectedValue, function);
}
}
@Override
public long injectInto(long injectedValue, LongObjectToLongFunction super T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().injectInto(injectedValue, function);
}
}
@Override
public double injectInto(double injectedValue, DoubleObjectToDoubleFunction super T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().injectInto(injectedValue, function);
}
}
@Override
public float injectInto(float injectedValue, FloatObjectToFloatFunction super T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().injectInto(injectedValue, function);
}
}
@Override
public long sumOfInt(IntFunction super T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().sumOfInt(function);
}
}
@Override
public double sumOfFloat(FloatFunction super T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().sumOfFloat(function);
}
}
@Override
public long sumOfLong(LongFunction super T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().sumOfLong(function);
}
}
@Override
public double sumOfDouble(DoubleFunction super T> function)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().sumOfDouble(function);
}
}
@Override
public MutableObjectLongMap sumByInt(Function super T, ? extends V> groupBy, IntFunction super T> function)
{
MutableObjectLongMap result = ObjectLongMaps.mutable.empty();
return this.injectInto(result, PrimitiveFunctions.sumByIntFunction(groupBy, function));
}
@Override
public MutableObjectDoubleMap sumByFloat(Function super T, ? extends V> groupBy, FloatFunction super T> function)
{
MutableObjectDoubleMap result = ObjectDoubleMaps.mutable.empty();
return this.injectInto(result, PrimitiveFunctions.sumByFloatFunction(groupBy, function));
}
@Override
public MutableObjectLongMap sumByLong(Function super T, ? extends V> groupBy, LongFunction super T> function)
{
MutableObjectLongMap result = ObjectLongMaps.mutable.empty();
return this.injectInto(result, PrimitiveFunctions.sumByLongFunction(groupBy, function));
}
@Override
public MutableObjectDoubleMap sumByDouble(Function super T, ? extends V> groupBy, DoubleFunction super T> function)
{
MutableObjectDoubleMap result = ObjectDoubleMaps.mutable.empty();
return this.injectInto(result, PrimitiveFunctions.sumByDoubleFunction(groupBy, function));
}
@Override
public IV injectIntoWith(
IV injectValue,
Function3 super IV, ? super T, ? super P, ? extends IV> function,
P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().injectIntoWith(injectValue, function, parameter);
}
}
@Override
public boolean removeIf(Predicate super T> predicate)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().removeIf(predicate);
}
}
@Override
public boolean removeIfWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().removeIfWith(predicate, parameter);
}
}
@Override
public boolean add(T item)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().add(item);
}
}
@Override
public boolean addAll(Collection extends T> collection)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().addAll(collection);
}
}
@Override
public boolean addAllIterable(Iterable extends T> iterable)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().addAllIterable(iterable);
}
}
@Override
public void clear()
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
this.getDelegate().clear();
}
}
@Override
public boolean isEmpty()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().isEmpty();
}
}
/**
* This method is not supported directly on MultiReader collections because it is not thread-safe. If you would like
* to use an iterator with a MultiReader collection, then you must do the following:
*
*
* multiReaderList.withReadLockAndDelegate(MutableList<Person> ->
* {
* Iterator it = people.iterator();
* ....
* });
*
*
*
* final Collection jdkSet = new HashSet();
* final boolean containsAll = new boolean[1];
* multiReaderList.withReadLockAndDelegate(MutableList<Person> people ->
* {
* set.addAll(people); // addAll uses iterator() in AbstractCollection
* containsAll[0] = set.containsAll(people); // containsAll uses iterator() in AbstractCollection
* });
*
*/
@Override
public Iterator iterator()
{
throw new UnsupportedOperationException(
"Iterator is not supported directly on MultiReader collections. "
+ "If you would like to use an iterator, you must either use withReadLockAndDelegate() or withWriteLockAndDelegate().");
}
/**
* This method is not supported directly on MultiReader collections because it is not thread-safe. If you would like
* to use a spliterator with a MultiReader collection, then you must protect the calls by calling either
* withReadLockAndDelegate or withWriteLockAndDelegate.
*/
@Override
public Spliterator spliterator()
{
throw new UnsupportedOperationException(
"Spliterator is not supported directly on MultiReader collections. "
+ "If you would like to use an spliterator, you must either use withReadLockAndDelegate() or withWriteLockAndDelegate().");
}
/**
* This method is not supported directly on MultiReader collections because it is not thread-safe. If you would like
* to use stream with a MultiReader collection, then you must protect the calls by calling either
* withReadLockAndDelegate or withWriteLockAndDelegate.
*/
@Override
public Stream stream()
{
throw new UnsupportedOperationException(
"Stream is not supported directly on MultiReader collections. "
+ "If you would like to use stream, you must either use withReadLockAndDelegate() or withWriteLockAndDelegate().");
}
/**
* This method is not supported directly on MultiReader collections because it is not thread-safe. If you would like
* to use parallelStream with a MultiReader collection, then you must protect the calls by calling either
* withReadLockAndDelegate or withWriteLockAndDelegate.
*/
@Override
public Stream parallelStream()
{
throw new UnsupportedOperationException(
"parallelStream is not supported directly on MultiReader collections. "
+ "If you would like to use parallelStream, you must either use withReadLockAndDelegate() or withWriteLockAndDelegate().");
}
@Override
public boolean remove(Object item)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().remove(item);
}
}
@Override
public boolean removeAll(Collection> collection)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().removeAll(collection);
}
}
@Override
public boolean removeAllIterable(Iterable> iterable)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().removeAllIterable(iterable);
}
}
@Override
public boolean retainAll(Collection> collection)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().retainAll(collection);
}
}
@Override
public boolean retainAllIterable(Iterable> iterable)
{
try (LockWrapper wrapper = this.lockWrapper.acquireWriteLock())
{
return this.getDelegate().retainAllIterable(iterable);
}
}
@Override
public int size()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().size();
}
}
@Override
public Object[] toArray()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toArray();
}
}
@Override
public E[] toArray(E[] a)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toArray(a);
}
}
@Override
public void each(Procedure super T> procedure)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
this.getDelegate().forEach(procedure);
}
}
@Override
public void forEachWith(Procedure2 super T, ? super P> procedure, P parameter)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
this.getDelegate().forEachWith(procedure, parameter);
}
}
@Override
public void forEachWithIndex(ObjectIntProcedure super T> objectIntProcedure)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
this.getDelegate().forEachWithIndex(objectIntProcedure);
}
}
@Override
public String toString()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().toString();
}
}
@Override
public String makeString()
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().makeString();
}
}
@Override
public String makeString(String separator)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().makeString(separator);
}
}
@Override
public String makeString(String start, String separator, String end)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().makeString(start, separator, end);
}
}
@Override
public void appendString(Appendable appendable)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
this.getDelegate().appendString(appendable);
}
}
@Override
public void appendString(Appendable appendable, String separator)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
this.getDelegate().appendString(appendable, separator);
}
}
@Override
public void appendString(Appendable appendable, String start, String separator, String end)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
this.getDelegate().appendString(appendable, start, separator, end);
}
}
@Override
public > R groupBy(
Function super T, ? extends V> function,
R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().groupBy(function, target);
}
}
@Override
public > R groupByEach(
Function super T, ? extends Iterable> function,
R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().groupByEach(function, target);
}
}
@Override
public > R groupByUniqueKey(
Function super T, ? extends V> function,
R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().groupByUniqueKey(function, target);
}
}
@Override
public >> R zip(Iterable that, R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().zip(that, target);
}
}
@Override
public >> R zipWithIndex(R target)
{
try (LockWrapper wrapper = this.lockWrapper.acquireReadLock())
{
return this.getDelegate().zipWithIndex(target);
}
}
@Override
public MutableMap aggregateInPlaceBy(
Function super T, ? extends K> groupBy,
Function0 extends V> zeroValueFactory,
Procedure2 super V, ? super T> mutatingAggregator)
{
MutableMap map = UnifiedMap.newMap();
this.forEach(new MutatingAggregationProcedure<>(map, groupBy, zeroValueFactory, mutatingAggregator));
return map;
}
protected abstract static class UntouchableMutableCollection
implements MutableCollection
{
protected MutableCollection delegate;
@Override
public boolean allSatisfy(Predicate super T> predicate)
{
return this.delegate.allSatisfy(predicate);
}
@Override
public boolean allSatisfyWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
return this.delegate.allSatisfyWith(predicate, parameter);
}
@Override
public boolean noneSatisfy(Predicate super T> predicate)
{
return this.delegate.noneSatisfy(predicate);
}
@Override
public
boolean noneSatisfyWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
return this.delegate.noneSatisfyWith(predicate, parameter);
}
@Override
public boolean anySatisfy(Predicate super T> predicate)
{
return this.delegate.anySatisfy(predicate);
}
@Override
public
boolean anySatisfyWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
return this.delegate.anySatisfyWith(predicate, parameter);
}
@Override
public > R into(R target)
{
return this.delegate.into(target);
}
@Override
public MutableList toList()
{
return this.delegate.toList();
}
@Override
public MutableMap toMap(
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
return this.delegate.toMap(keyFunction, valueFunction);
}
@Override
public > R toMap(
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction,
R target)
{
return this.delegate.toMap(keyFunction, valueFunction, target);
}
@Override
public MutableSortedMap toSortedMap(
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
return this.delegate.toSortedMap(keyFunction, valueFunction);
}
@Override
public MutableSortedMap toSortedMap(
Comparator super NK> comparator,
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
return this.delegate.toSortedMap(comparator, keyFunction, valueFunction);
}
@Override
public , NK, NV> MutableSortedMap toSortedMapBy(
Function super NK, KK> sortBy,
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
return this.delegate.toSortedMapBy(sortBy, keyFunction, valueFunction);
}
@Override
public MutableBiMap toBiMap(
Function super T, ? extends NK> keyFunction,
Function super T, ? extends NV> valueFunction)
{
return this.delegate.toBiMap(keyFunction, valueFunction);
}
@Override
public MutableSet toSet()
{
return this.delegate.toSet();
}
@Override
public MutableBag toBag()
{
return this.delegate.toBag();
}
@Override
public MutableSortedBag toSortedBag()
{
return this.delegate.toSortedBag();
}
@Override
public MutableSortedBag toSortedBag(Comparator super T> comparator)
{
return this.delegate.toSortedBag(comparator);
}
@Override
public > MutableSortedBag toSortedBagBy(Function super T, ? extends V> function)
{
return this.delegate.toSortedBagBy(function);
}
@Override
public MutableList toSortedList()
{
return this.delegate.toSortedList();
}
@Override
public MutableList toSortedList(Comparator super T> comparator)
{
return this.delegate.toSortedList(comparator);
}
@Override
public > MutableList toSortedListBy(Function super T, ? extends V> function)
{
return this.delegate.toSortedListBy(function);
}
@Override
public MutableSortedSet toSortedSet()
{
return this.delegate.toSortedSet();
}
@Override
public MutableSortedSet toSortedSet(Comparator super T> comparator)
{
return this.delegate.toSortedSet(comparator);
}
@Override
public > MutableSortedSet toSortedSetBy(Function super T, ? extends V> function)
{
return this.delegate.toSortedSetBy(function);
}
@Override
public > R collect(
Function super T, ? extends V> function,
R target)
{
return this.delegate.collect(function, target);
}
@Override
public > R flatCollect(
Function super T, ? extends Iterable> function,
R target)
{
return this.delegate.flatCollect(function, target);
}
@Override
public > R collectIf(
Predicate super T> predicate,
Function super T, ? extends V> function,
R target)
{
return this.delegate.collectIf(predicate, function, target);
}
@Override
public > R collectWith(
Function2 super T, ? super P, ? extends V> function,
P parameter,
R targetCollection)
{
return this.delegate.collectWith(function, parameter, targetCollection);
}
@Override
public > R groupBy(
Function super T, ? extends V> function,
R target)
{
return this.delegate.groupBy(function, target);
}
@Override
public > R groupByEach(
Function super T, ? extends Iterable> function,
R target)
{
return this.delegate.groupByEach(function, target);
}
@Override
public > R groupByUniqueKey(
Function super T, ? extends V> function,
R target)
{
return this.delegate.groupByUniqueKey(function, target);
}
@Override
public int count(Predicate super T> predicate)
{
return this.delegate.count(predicate);
}
@Override
public int countWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.delegate.countWith(predicate, parameter);
}
@Override
public T detect(Predicate super T> predicate)
{
return this.delegate.detect(predicate);
}
@Override
public
T detectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.delegate.detectWith(predicate, parameter);
}
@Override
public Optional detectOptional(Predicate super T> predicate)
{
return this.delegate.detectOptional(predicate);
}
@Override
public Optional detectWithOptional(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.delegate.detectWithOptional(predicate, parameter);
}
@Override
public T detectIfNone(Predicate super T> predicate, Function0 extends T> function)
{
return this.delegate.detectIfNone(predicate, function);
}
@Override
public T detectWithIfNone(
Predicate2 super T, ? super P> predicate,
P parameter,
Function0 extends T> function)
{
return this.delegate.detectWithIfNone(predicate, parameter, function);
}
@Override
public T min(Comparator super T> comparator)
{
return this.delegate.min(comparator);
}
@Override
public T max(Comparator super T> comparator)
{
return this.delegate.max(comparator);
}
@Override
public T min()
{
return this.delegate.min();
}
@Override
public T max()
{
return this.delegate.max();
}
@Override
public > T minBy(Function super T, ? extends V> function)
{
return this.delegate.minBy(function);
}
@Override
public > T maxBy(Function super T, ? extends V> function)
{
return this.delegate.maxBy(function);
}
@Override
public T getFirst()
{
return this.delegate.getFirst();
}
@Override
public T getLast()
{
return this.delegate.getLast();
}
@Override
public T getOnly()
{
return this.delegate.getOnly();
}
@Override
public IV injectInto(
IV injectedValue,
Function2 super IV, ? super T, ? extends IV> function)
{
return this.delegate.injectInto(injectedValue, function);
}
@Override
public int injectInto(int injectedValue, IntObjectToIntFunction super T> function)
{
return this.delegate.injectInto(injectedValue, function);
}
@Override
public long injectInto(long injectedValue, LongObjectToLongFunction super T> function)
{
return this.delegate.injectInto(injectedValue, function);
}
@Override
public double injectInto(double injectedValue, DoubleObjectToDoubleFunction super T> function)
{
return this.delegate.injectInto(injectedValue, function);
}
@Override
public float injectInto(float injectedValue, FloatObjectToFloatFunction super T> function)
{
return this.delegate.injectInto(injectedValue, function);
}
@Override
public long sumOfInt(IntFunction super T> function)
{
return this.delegate.sumOfInt(function);
}
@Override
public double sumOfFloat(FloatFunction super T> function)
{
return this.delegate.sumOfFloat(function);
}
@Override
public long sumOfLong(LongFunction super T> function)
{
return this.delegate.sumOfLong(function);
}
@Override
public double sumOfDouble(DoubleFunction super T> function)
{
return this.delegate.sumOfDouble(function);
}
@Override
public MutableObjectLongMap sumByInt(Function super T, ? extends V> groupBy, IntFunction super T> function)
{
return this.delegate.sumByInt(groupBy, function);
}
@Override
public MutableObjectDoubleMap sumByFloat(Function super T, ? extends V> groupBy, FloatFunction super T> function)
{
return this.delegate.sumByFloat(groupBy, function);
}
@Override
public MutableObjectLongMap sumByLong(Function super T, ? extends V> groupBy, LongFunction super T> function)
{
return this.delegate.sumByLong(groupBy, function);
}
@Override
public MutableObjectDoubleMap sumByDouble(Function super T, ? extends V> groupBy, DoubleFunction super T> function)
{
return this.delegate.sumByDouble(groupBy, function);
}
@Override
public IV injectIntoWith(
IV injectValue,
Function3 super IV, ? super T, ? super P, ? extends IV> function,
P parameter)
{
return this.delegate.injectIntoWith(injectValue, function, parameter);
}
@Override
public boolean notEmpty()
{
return this.delegate.notEmpty();
}
@Override
public > R reject(Predicate super T> predicate, R target)
{
return this.delegate.reject(predicate, target);
}
@Override
public > R rejectWith(
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
return this.delegate.rejectWith(predicate, parameter, targetCollection);
}
@Override
public boolean removeIf(Predicate super T> predicate)
{
return this.delegate.removeIf(predicate);
}
@Override
public
boolean removeIfWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
return this.delegate.removeIfWith(predicate, parameter);
}
@Override
public > R select(Predicate super T> predicate, R target)
{
return this.delegate.select(predicate, target);
}
@Override
public Twin> selectAndRejectWith(
Predicate2 super T, ? super P> predicate,
P parameter)
{
return this.delegate.selectAndRejectWith(predicate, parameter);
}
@Override
public > R selectWith(
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
return this.delegate.selectWith(predicate, parameter, targetCollection);
}
@Override
public boolean add(T o)
{
return this.delegate.add(o);
}
@Override
public boolean addAll(Collection extends T> collection)
{
return this.delegate.addAll(collection);
}
@Override
public boolean addAllIterable(Iterable extends T> iterable)
{
return this.delegate.addAllIterable(iterable);
}
@Override
public void clear()
{
this.delegate.clear();
}
@Override
public boolean contains(Object o)
{
return this.delegate.contains(o);
}
@Override
public boolean containsAll(Collection> collection)
{
return this.delegate.containsAll(collection);
}
@Override
public boolean containsAllIterable(Iterable> source)
{
return this.delegate.containsAllIterable(source);
}
@Override
public boolean containsAllArguments(Object... elements)
{
return this.delegate.containsAllArguments(elements);
}
@Override
public boolean equals(Object o)
{
return this.delegate.equals(o);
}
@Override
public int hashCode()
{
return this.delegate.hashCode();
}
@Override
public boolean isEmpty()
{
return this.delegate.isEmpty();
}
@Override
public boolean remove(Object o)
{
return this.delegate.remove(o);
}
@Override
public boolean removeAll(Collection> collection)
{
return this.delegate.removeAll(collection);
}
@Override
public boolean removeAllIterable(Iterable> iterable)
{
return this.delegate.removeAllIterable(iterable);
}
@Override
public boolean retainAll(Collection> collection)
{
return this.delegate.retainAll(collection);
}
@Override
public boolean retainAllIterable(Iterable> iterable)
{
return this.delegate.retainAllIterable(iterable);
}
@Override
public int size()
{
return this.delegate.size();
}
@Override
public Object[] toArray()
{
return this.delegate.toArray();
}
@Override
public E[] toArray(E[] a)
{
return this.delegate.toArray(a);
}
@Override
public void each(Procedure super T> procedure)
{
this.delegate.forEach(procedure);
}
@Override
public void forEachWith(Procedure2 super T, ? super P> procedure, P parameter)
{
this.delegate.forEachWith(procedure, parameter);
}
@Override
public void forEachWithIndex(ObjectIntProcedure super T> objectIntProcedure)
{
this.delegate.forEachWithIndex(objectIntProcedure);
}
@Override
public String toString()
{
return this.delegate.toString();
}
@Override
public String makeString()
{
return this.delegate.makeString();
}
@Override
public String makeString(String separator)
{
return this.delegate.makeString(separator);
}
@Override
public String makeString(String start, String separator, String end)
{
return this.delegate.makeString(start, separator, end);
}
@Override
public void appendString(Appendable appendable)
{
this.delegate.appendString(appendable);
}
@Override
public void appendString(Appendable appendable, String separator)
{
this.delegate.appendString(appendable, separator);
}
@Override
public void appendString(Appendable appendable, String start, String separator, String end)
{
this.delegate.appendString(appendable, start, separator, end);
}
@Override
public >> R zip(Iterable that, R target)
{
return this.delegate.zip(that, target);
}
@Override
public >> R zipWithIndex(R target)
{
return this.delegate.zipWithIndex(target);
}
@Override
public RichIterable> chunk(int size)
{
return this.delegate.chunk(size);
}
@Override
public MutableMap aggregateInPlaceBy(
Function super T, ? extends K> groupBy,
Function0 extends V> zeroValueFactory,
Procedure2 super V, ? super T> mutatingAggregator)
{
MutableMap map = UnifiedMap.newMap();
this.each(each -> {
K key = groupBy.valueOf(each);
V value = map.getIfAbsentPut(key, zeroValueFactory);
mutatingAggregator.value(value, each);
});
return map;
}
}
public static class ReadWriteLockWrapper
{
private final LockWrapper readLock;
private final LockWrapper writeLock;
public ReadWriteLockWrapper(ReadWriteLock readWriteLock)
{
this.readLock = new LockWrapper(readWriteLock.readLock());
this.writeLock = new LockWrapper(readWriteLock.writeLock());
}
/**
* This method must be wrapped in a try block.
*/
public LockWrapper acquireReadLock()
{
this.readLock.lock.lock();
return this.readLock;
}
/**
* This method must be wrapped in a try block.
*/
public LockWrapper acquireWriteLock()
{
this.writeLock.lock.lock();
return this.writeLock;
}
}
public static class LockWrapper implements AutoCloseable
{
private final Lock lock;
public LockWrapper(Lock lock)
{
this.lock = lock;
}
@Override
public void close()
{
this.lock.unlock();
}
}
}