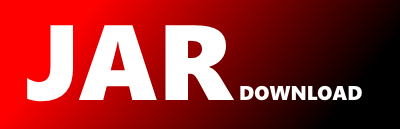
org.eclipse.collections.impl.SynchronizedRichIterable Maven / Gradle / Ivy
/*
* Copyright (c) 2015 Goldman Sachs.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl;
import java.io.Serializable;
import java.util.Collection;
import java.util.Comparator;
import java.util.Iterator;
import net.jcip.annotations.GuardedBy;
import org.eclipse.collections.api.BooleanIterable;
import org.eclipse.collections.api.ByteIterable;
import org.eclipse.collections.api.CharIterable;
import org.eclipse.collections.api.DoubleIterable;
import org.eclipse.collections.api.FloatIterable;
import org.eclipse.collections.api.IntIterable;
import org.eclipse.collections.api.LazyIterable;
import org.eclipse.collections.api.LongIterable;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.ShortIterable;
import org.eclipse.collections.api.bag.MutableBag;
import org.eclipse.collections.api.bag.sorted.MutableSortedBag;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function0;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.function.primitive.BooleanFunction;
import org.eclipse.collections.api.block.function.primitive.ByteFunction;
import org.eclipse.collections.api.block.function.primitive.CharFunction;
import org.eclipse.collections.api.block.function.primitive.DoubleFunction;
import org.eclipse.collections.api.block.function.primitive.DoubleObjectToDoubleFunction;
import org.eclipse.collections.api.block.function.primitive.FloatFunction;
import org.eclipse.collections.api.block.function.primitive.FloatObjectToFloatFunction;
import org.eclipse.collections.api.block.function.primitive.IntFunction;
import org.eclipse.collections.api.block.function.primitive.IntObjectToIntFunction;
import org.eclipse.collections.api.block.function.primitive.LongFunction;
import org.eclipse.collections.api.block.function.primitive.LongObjectToLongFunction;
import org.eclipse.collections.api.block.function.primitive.ShortFunction;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.block.procedure.Procedure;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.block.procedure.primitive.ObjectIntProcedure;
import org.eclipse.collections.api.collection.primitive.MutableBooleanCollection;
import org.eclipse.collections.api.collection.primitive.MutableByteCollection;
import org.eclipse.collections.api.collection.primitive.MutableCharCollection;
import org.eclipse.collections.api.collection.primitive.MutableDoubleCollection;
import org.eclipse.collections.api.collection.primitive.MutableFloatCollection;
import org.eclipse.collections.api.collection.primitive.MutableIntCollection;
import org.eclipse.collections.api.collection.primitive.MutableLongCollection;
import org.eclipse.collections.api.collection.primitive.MutableShortCollection;
import org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.api.map.MapIterable;
import org.eclipse.collections.api.map.MutableMap;
import org.eclipse.collections.api.map.primitive.ObjectDoubleMap;
import org.eclipse.collections.api.map.primitive.ObjectLongMap;
import org.eclipse.collections.api.map.sorted.MutableSortedMap;
import org.eclipse.collections.api.multimap.Multimap;
import org.eclipse.collections.api.multimap.MutableMultimap;
import org.eclipse.collections.api.partition.PartitionIterable;
import org.eclipse.collections.api.set.MutableSet;
import org.eclipse.collections.api.set.sorted.MutableSortedSet;
import org.eclipse.collections.api.tuple.Pair;
/**
* A synchronized view of a RichIterable.
*
* @since 5.0
*/
public class SynchronizedRichIterable
implements RichIterable, Serializable
{
private static final long serialVersionUID = 1L;
private final Object lock;
@GuardedBy("this.lock")
private final RichIterable iterable;
protected SynchronizedRichIterable(RichIterable iterable)
{
this(iterable, null);
}
protected SynchronizedRichIterable(RichIterable iterable, Object newLock)
{
if (iterable == null)
{
throw new IllegalArgumentException("Cannot create a SynchronizedRichIterable on a null collection");
}
this.iterable = iterable;
this.lock = newLock == null ? this : newLock;
}
/**
* This method will take a RichIterable and wrap it directly in a SynchronizedRichIterable.
*/
public static SynchronizedRichIterable of(RichIterable iterable)
{
return new SynchronizedRichIterable(iterable);
}
/**
* This method will take a RichIterable and wrap it directly in a SynchronizedRichIterable. Additionally,
* a developer specifies which lock to use with the collection.
*/
public static SynchronizedRichIterable of(RichIterable iterable, Object lock)
{
return new SynchronizedRichIterable(iterable, lock);
}
public int size()
{
synchronized (this.lock)
{
return this.iterable.size();
}
}
public boolean isEmpty()
{
synchronized (this.lock)
{
return this.iterable.isEmpty();
}
}
public boolean notEmpty()
{
synchronized (this.lock)
{
return this.iterable.notEmpty();
}
}
public T getFirst()
{
synchronized (this.lock)
{
return this.iterable.getFirst();
}
}
public T getLast()
{
synchronized (this.lock)
{
return this.iterable.getLast();
}
}
public boolean contains(Object object)
{
synchronized (this.lock)
{
return this.iterable.contains(object);
}
}
public boolean containsAllIterable(Iterable> source)
{
synchronized (this.lock)
{
return this.iterable.containsAllIterable(source);
}
}
public boolean containsAll(Collection> source)
{
synchronized (this.lock)
{
return this.iterable.containsAll(source);
}
}
public boolean containsAllArguments(Object... elements)
{
synchronized (this.lock)
{
return this.iterable.containsAllArguments(elements);
}
}
public RichIterable select(Predicate super T> predicate)
{
synchronized (this.lock)
{
return this.iterable.select(predicate);
}
}
public > R select(Predicate super T> predicate, R target)
{
synchronized (this.lock)
{
return this.iterable.select(predicate, target);
}
}
public RichIterable selectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.lock)
{
return this.iterable.selectWith(predicate, parameter);
}
}
public > R selectWith(Predicate2 super T, ? super P> predicate, P parameter, R targetCollection)
{
synchronized (this.lock)
{
return this.iterable.selectWith(predicate, parameter, targetCollection);
}
}
public RichIterable reject(Predicate super T> predicate)
{
synchronized (this.lock)
{
return this.iterable.reject(predicate);
}
}
public RichIterable rejectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.lock)
{
return this.iterable.rejectWith(predicate, parameter);
}
}
public > R reject(Predicate super T> predicate, R target)
{
synchronized (this.lock)
{
return this.iterable.reject(predicate, target);
}
}
public > R rejectWith(Predicate2 super T, ? super P> predicate, P parameter, R targetCollection)
{
synchronized (this.lock)
{
return this.iterable.rejectWith(predicate, parameter, targetCollection);
}
}
public PartitionIterable partition(Predicate super T> predicate)
{
synchronized (this.lock)
{
return this.iterable.partition(predicate);
}
}
public PartitionIterable partitionWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.lock)
{
return this.iterable.partitionWith(predicate, parameter);
}
}
public RichIterable selectInstancesOf(Class clazz)
{
synchronized (this.lock)
{
return this.iterable.selectInstancesOf(clazz);
}
}
public RichIterable collect(Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.iterable.collect(function);
}
}
public BooleanIterable collectBoolean(BooleanFunction super T> booleanFunction)
{
synchronized (this.lock)
{
return this.iterable.collectBoolean(booleanFunction);
}
}
public R collectBoolean(BooleanFunction super T> booleanFunction, R target)
{
synchronized (this.lock)
{
return this.iterable.collectBoolean(booleanFunction, target);
}
}
public ByteIterable collectByte(ByteFunction super T> byteFunction)
{
synchronized (this.lock)
{
return this.iterable.collectByte(byteFunction);
}
}
public R collectByte(ByteFunction super T> byteFunction, R target)
{
synchronized (this.lock)
{
return this.iterable.collectByte(byteFunction, target);
}
}
public CharIterable collectChar(CharFunction super T> charFunction)
{
synchronized (this.lock)
{
return this.iterable.collectChar(charFunction);
}
}
public R collectChar(CharFunction super T> charFunction, R target)
{
synchronized (this.lock)
{
return this.iterable.collectChar(charFunction, target);
}
}
public DoubleIterable collectDouble(DoubleFunction super T> doubleFunction)
{
synchronized (this.lock)
{
return this.iterable.collectDouble(doubleFunction);
}
}
public R collectDouble(DoubleFunction super T> doubleFunction, R target)
{
synchronized (this.lock)
{
return this.iterable.collectDouble(doubleFunction, target);
}
}
public FloatIterable collectFloat(FloatFunction super T> floatFunction)
{
synchronized (this.lock)
{
return this.iterable.collectFloat(floatFunction);
}
}
public R collectFloat(FloatFunction super T> floatFunction, R target)
{
synchronized (this.lock)
{
return this.iterable.collectFloat(floatFunction, target);
}
}
public IntIterable collectInt(IntFunction super T> intFunction)
{
synchronized (this.lock)
{
return this.iterable.collectInt(intFunction);
}
}
public R collectInt(IntFunction super T> intFunction, R target)
{
synchronized (this.lock)
{
return this.iterable.collectInt(intFunction, target);
}
}
public LongIterable collectLong(LongFunction super T> longFunction)
{
synchronized (this.lock)
{
return this.iterable.collectLong(longFunction);
}
}
public R collectLong(LongFunction super T> longFunction, R target)
{
synchronized (this.lock)
{
return this.iterable.collectLong(longFunction, target);
}
}
public ShortIterable collectShort(ShortFunction super T> shortFunction)
{
synchronized (this.lock)
{
return this.iterable.collectShort(shortFunction);
}
}
public R collectShort(ShortFunction super T> shortFunction, R target)
{
synchronized (this.lock)
{
return this.iterable.collectShort(shortFunction, target);
}
}
public > R collect(Function super T, ? extends V> function, R target)
{
synchronized (this.lock)
{
return this.iterable.collect(function, target);
}
}
public RichIterable collectWith(Function2 super T, ? super P, ? extends V> function, P parameter)
{
synchronized (this.lock)
{
return this.iterable.collectWith(function, parameter);
}
}
public > R collectWith(Function2 super T, ? super P, ? extends V> function, P parameter, R targetCollection)
{
synchronized (this.lock)
{
return this.iterable.collectWith(function, parameter, targetCollection);
}
}
public RichIterable collectIf(Predicate super T> predicate, Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.iterable.collectIf(predicate, function);
}
}
public > R collectIf(Predicate super T> predicate, Function super T, ? extends V> function, R target)
{
synchronized (this.lock)
{
return this.iterable.collectIf(predicate, function, target);
}
}
public RichIterable flatCollect(Function super T, ? extends Iterable> function)
{
synchronized (this.lock)
{
return this.iterable.flatCollect(function);
}
}
public > R flatCollect(Function super T, ? extends Iterable> function, R target)
{
synchronized (this.lock)
{
return this.iterable.flatCollect(function, target);
}
}
public T detect(Predicate super T> predicate)
{
synchronized (this.lock)
{
return this.iterable.detect(predicate);
}
}
public T detectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.lock)
{
return this.iterable.detectWith(predicate, parameter);
}
}
public T detectIfNone(Predicate super T> predicate, Function0 extends T> function)
{
synchronized (this.lock)
{
return this.iterable.detectIfNone(predicate, function);
}
}
public
T detectWithIfNone(Predicate2 super T, ? super P> predicate, P parameter, Function0 extends T> function)
{
synchronized (this.lock)
{
return this.iterable.detectWithIfNone(predicate, parameter, function);
}
}
public int count(Predicate super T> predicate)
{
synchronized (this.lock)
{
return this.iterable.count(predicate);
}
}
public
int countWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.lock)
{
return this.iterable.countWith(predicate, parameter);
}
}
public boolean anySatisfy(Predicate super T> predicate)
{
synchronized (this.lock)
{
return this.iterable.anySatisfy(predicate);
}
}
public
boolean anySatisfyWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.lock)
{
return this.iterable.anySatisfyWith(predicate, parameter);
}
}
public boolean allSatisfy(Predicate super T> predicate)
{
synchronized (this.lock)
{
return this.iterable.allSatisfy(predicate);
}
}
public
boolean allSatisfyWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.lock)
{
return this.iterable.allSatisfyWith(predicate, parameter);
}
}
public boolean noneSatisfy(Predicate super T> predicate)
{
synchronized (this.lock)
{
return this.iterable.noneSatisfy(predicate);
}
}
public
boolean noneSatisfyWith(Predicate2 super T, ? super P> predicate, P parameter)
{
synchronized (this.lock)
{
return this.iterable.noneSatisfyWith(predicate, parameter);
}
}
public IV injectInto(IV injectedValue, Function2 super IV, ? super T, ? extends IV> function)
{
synchronized (this.lock)
{
return this.iterable.injectInto(injectedValue, function);
}
}
public int injectInto(int injectedValue, IntObjectToIntFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.injectInto(injectedValue, function);
}
}
public long injectInto(long injectedValue, LongObjectToLongFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.injectInto(injectedValue, function);
}
}
public float injectInto(float injectedValue, FloatObjectToFloatFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.injectInto(injectedValue, function);
}
}
public double injectInto(double injectedValue, DoubleObjectToDoubleFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.injectInto(injectedValue, function);
}
}
public MutableList toList()
{
synchronized (this.lock)
{
return this.iterable.toList();
}
}
public MutableList toSortedList()
{
synchronized (this.lock)
{
return this.iterable.toSortedList();
}
}
public MutableList toSortedList(Comparator super T> comparator)
{
synchronized (this.lock)
{
return this.iterable.toSortedList(comparator);
}
}
public > MutableList toSortedListBy(Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.iterable.toSortedListBy(function);
}
}
public MutableSet toSet()
{
synchronized (this.lock)
{
return this.iterable.toSet();
}
}
public MutableSortedSet toSortedSet()
{
synchronized (this.lock)
{
return this.iterable.toSortedSet();
}
}
public MutableSortedSet toSortedSet(Comparator super T> comparator)
{
synchronized (this.lock)
{
return this.iterable.toSortedSet(comparator);
}
}
public > MutableSortedSet toSortedSetBy(Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.iterable.toSortedSetBy(function);
}
}
public MutableBag toBag()
{
synchronized (this.lock)
{
return this.iterable.toBag();
}
}
public MutableSortedBag toSortedBag()
{
synchronized (this.lock)
{
return this.iterable.toSortedBag();
}
}
public MutableSortedBag toSortedBag(Comparator super T> comparator)
{
synchronized (this.lock)
{
return this.iterable.toSortedBag(comparator);
}
}
public > MutableSortedBag toSortedBagBy(Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.iterable.toSortedBagBy(function);
}
}
public MutableMap toMap(Function super T, ? extends NK> keyFunction, Function super T, ? extends NV> valueFunction)
{
synchronized (this.lock)
{
return this.iterable.toMap(keyFunction, valueFunction);
}
}
public MutableSortedMap toSortedMap(Function super T, ? extends NK> keyFunction, Function super T, ? extends NV> valueFunction)
{
synchronized (this.lock)
{
return this.iterable.toSortedMap(keyFunction, valueFunction);
}
}
public MutableSortedMap toSortedMap(Comparator super NK> comparator, Function super T, ? extends NK> keyFunction, Function super T, ? extends NV> valueFunction)
{
synchronized (this.lock)
{
return this.iterable.toSortedMap(comparator, keyFunction, valueFunction);
}
}
public LazyIterable asLazy()
{
synchronized (this.lock)
{
return this.iterable.asLazy();
}
}
public Object[] toArray()
{
synchronized (this.lock)
{
return this.iterable.toArray();
}
}
public T1[] toArray(T1[] target)
{
synchronized (this.lock)
{
return this.iterable.toArray(target);
}
}
public T min(Comparator super T> comparator)
{
synchronized (this.lock)
{
return this.iterable.min(comparator);
}
}
public T max(Comparator super T> comparator)
{
synchronized (this.lock)
{
return this.iterable.max(comparator);
}
}
public T min()
{
synchronized (this.lock)
{
return this.iterable.min();
}
}
public T max()
{
synchronized (this.lock)
{
return this.iterable.max();
}
}
public > T minBy(Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.iterable.minBy(function);
}
}
public > T maxBy(Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.iterable.maxBy(function);
}
}
public long sumOfInt(IntFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.sumOfInt(function);
}
}
public double sumOfFloat(FloatFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.sumOfFloat(function);
}
}
public long sumOfLong(LongFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.sumOfLong(function);
}
}
public double sumOfDouble(DoubleFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.sumOfDouble(function);
}
}
public ObjectLongMap sumByInt(Function groupBy, IntFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.sumByInt(groupBy, function);
}
}
public ObjectDoubleMap sumByFloat(Function groupBy, FloatFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.sumByFloat(groupBy, function);
}
}
public ObjectLongMap sumByLong(Function groupBy, LongFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.sumByLong(groupBy, function);
}
}
public ObjectDoubleMap sumByDouble(Function groupBy, DoubleFunction super T> function)
{
synchronized (this.lock)
{
return this.iterable.sumByDouble(groupBy, function);
}
}
public String makeString()
{
synchronized (this.lock)
{
return this.iterable.makeString();
}
}
public String makeString(String separator)
{
synchronized (this.lock)
{
return this.iterable.makeString(separator);
}
}
public String makeString(String start, String separator, String end)
{
synchronized (this.lock)
{
return this.iterable.makeString(start, separator, end);
}
}
public void appendString(Appendable appendable)
{
synchronized (this.lock)
{
this.appendString(appendable, ", ");
}
}
public void appendString(Appendable appendable, String separator)
{
synchronized (this.lock)
{
this.appendString(appendable, "", separator, "");
}
}
public void appendString(Appendable appendable, String start, String separator, String end)
{
synchronized (this.lock)
{
this.iterable.appendString(appendable, start, separator, end);
}
}
public Multimap groupBy(Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.iterable.groupBy(function);
}
}
public > R groupBy(Function super T, ? extends V> function, R target)
{
synchronized (this.lock)
{
return this.iterable.groupBy(function, target);
}
}
public Multimap groupByEach(Function super T, ? extends Iterable> function)
{
synchronized (this.lock)
{
return this.iterable.groupByEach(function);
}
}
public > R groupByEach(Function super T, ? extends Iterable> function, R target)
{
synchronized (this.lock)
{
return this.iterable.groupByEach(function, target);
}
}
public MapIterable groupByUniqueKey(Function super T, ? extends V> function)
{
synchronized (this.lock)
{
return this.iterable.groupByUniqueKey(function);
}
}
public > R groupByUniqueKey(Function super T, ? extends V> function, R target)
{
synchronized (this.lock)
{
return this.iterable.groupByUniqueKey(function, target);
}
}
@Override
public String toString()
{
synchronized (this.lock)
{
return this.iterable.toString();
}
}
public RichIterable> zip(Iterable that)
{
synchronized (this.lock)
{
return this.iterable.zip(that);
}
}
public >> R zip(Iterable that, R target)
{
synchronized (this.lock)
{
return this.iterable.zip(that, target);
}
}
public RichIterable> zipWithIndex()
{
synchronized (this.lock)
{
return this.iterable.zipWithIndex();
}
}
public >> R zipWithIndex(R target)
{
synchronized (this.lock)
{
return this.iterable.zipWithIndex(target);
}
}
public RichIterable> chunk(int size)
{
synchronized (this.lock)
{
return this.iterable.chunk(size);
}
}
public MapIterable aggregateInPlaceBy(Function super T, ? extends K> groupBy, Function0 extends V> zeroValueFactory, Procedure2 super V, ? super T> mutatingAggregator)
{
synchronized (this.lock)
{
return this.iterable.aggregateInPlaceBy(groupBy, zeroValueFactory, mutatingAggregator);
}
}
public MapIterable aggregateBy(Function super T, ? extends K> groupBy, Function0 extends V> zeroValueFactory, Function2 super V, ? super T, ? extends V> nonMutatingAggregator)
{
synchronized (this.lock)
{
return this.iterable.aggregateBy(groupBy, zeroValueFactory, nonMutatingAggregator);
}
}
public RichIterable tap(Procedure super T> procedure)
{
synchronized (this.lock)
{
this.forEach(procedure);
return this;
}
}
public void forEach(Procedure super T> procedure)
{
this.each(procedure);
}
public void each(Procedure super T> procedure)
{
synchronized (this.lock)
{
this.iterable.forEach(procedure);
}
}
public void forEachWithIndex(ObjectIntProcedure super T> objectIntProcedure)
{
synchronized (this.lock)
{
this.iterable.forEachWithIndex(objectIntProcedure);
}
}
public void forEachWith(Procedure2 super T, ? super P> procedure, P parameter)
{
synchronized (this.lock)
{
this.iterable.forEachWith(procedure, parameter);
}
}
/**
* Must be called in a synchronized block.
*/
public Iterator iterator()
{
return this.iterable.iterator();
}
}