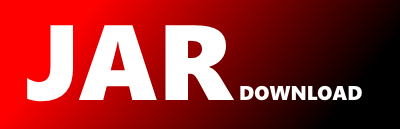
org.eclipse.collections.impl.lazy.AbstractLazyIterable Maven / Gradle / Ivy
/*
* Copyright (c) 2015 Goldman Sachs.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.lazy;
import java.util.Collection;
import net.jcip.annotations.Immutable;
import org.eclipse.collections.api.LazyBooleanIterable;
import org.eclipse.collections.api.LazyByteIterable;
import org.eclipse.collections.api.LazyCharIterable;
import org.eclipse.collections.api.LazyDoubleIterable;
import org.eclipse.collections.api.LazyFloatIterable;
import org.eclipse.collections.api.LazyIntIterable;
import org.eclipse.collections.api.LazyIterable;
import org.eclipse.collections.api.LazyLongIterable;
import org.eclipse.collections.api.LazyShortIterable;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function0;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.function.primitive.BooleanFunction;
import org.eclipse.collections.api.block.function.primitive.ByteFunction;
import org.eclipse.collections.api.block.function.primitive.CharFunction;
import org.eclipse.collections.api.block.function.primitive.DoubleFunction;
import org.eclipse.collections.api.block.function.primitive.FloatFunction;
import org.eclipse.collections.api.block.function.primitive.IntFunction;
import org.eclipse.collections.api.block.function.primitive.LongFunction;
import org.eclipse.collections.api.block.function.primitive.ShortFunction;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.block.procedure.Procedure;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.map.MapIterable;
import org.eclipse.collections.api.map.MutableMap;
import org.eclipse.collections.api.multimap.Multimap;
import org.eclipse.collections.api.partition.list.PartitionMutableList;
import org.eclipse.collections.api.stack.MutableStack;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.impl.AbstractRichIterable;
import org.eclipse.collections.impl.block.factory.Functions;
import org.eclipse.collections.impl.block.factory.Predicates;
import org.eclipse.collections.impl.block.factory.Procedures2;
import org.eclipse.collections.impl.block.procedure.MutatingAggregationProcedure;
import org.eclipse.collections.impl.block.procedure.NonMutatingAggregationProcedure;
import org.eclipse.collections.impl.block.procedure.PartitionProcedure;
import org.eclipse.collections.impl.lazy.primitive.CollectBooleanIterable;
import org.eclipse.collections.impl.lazy.primitive.CollectByteIterable;
import org.eclipse.collections.impl.lazy.primitive.CollectCharIterable;
import org.eclipse.collections.impl.lazy.primitive.CollectDoubleIterable;
import org.eclipse.collections.impl.lazy.primitive.CollectFloatIterable;
import org.eclipse.collections.impl.lazy.primitive.CollectIntIterable;
import org.eclipse.collections.impl.lazy.primitive.CollectLongIterable;
import org.eclipse.collections.impl.lazy.primitive.CollectShortIterable;
import org.eclipse.collections.impl.map.mutable.UnifiedMap;
import org.eclipse.collections.impl.multimap.list.FastListMultimap;
import org.eclipse.collections.impl.partition.list.PartitionFastList;
import org.eclipse.collections.impl.stack.mutable.ArrayStack;
import org.eclipse.collections.impl.utility.LazyIterate;
/**
* AbstractLazyIterable provides a base from which deferred iterables such as SelectIterable,
* RejectIterable and CollectIterable can be derived.
*/
@Immutable
public abstract class AbstractLazyIterable
extends AbstractRichIterable
implements LazyIterable
{
@Override
public LazyIterable asLazy()
{
return this;
}
public > R into(R target)
{
this.forEachWith(Procedures2.addToCollection(), target);
return target;
}
@Override
public E[] toArray(E[] array)
{
return this.toList().toArray(array);
}
public int size()
{
return this.count(Predicates.alwaysTrue());
}
@Override
public boolean isEmpty()
{
return !this.anySatisfy(Predicates.alwaysTrue());
}
public T getFirst()
{
return this.detect(Predicates.alwaysTrue());
}
public T getLast()
{
final T[] result = (T[]) new Object[1];
this.forEach(new Procedure()
{
public void value(T each)
{
result[0] = each;
}
});
return result[0];
}
public LazyIterable select(Predicate super T> predicate)
{
return LazyIterate.select(this, predicate);
}
public LazyIterable selectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return LazyIterate.select(this, Predicates.bind(predicate, parameter));
}
public LazyIterable reject(Predicate super T> predicate)
{
return LazyIterate.reject(this, predicate);
}
public LazyIterable rejectWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return LazyIterate.reject(this, Predicates.bind(predicate, parameter));
}
public PartitionMutableList partition(Predicate super T> predicate)
{
PartitionMutableList partitionMutableList = new PartitionFastList();
this.forEach(new PartitionProcedure(predicate, partitionMutableList));
return partitionMutableList;
}
public PartitionMutableList partitionWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return this.partition(Predicates.bind(predicate, parameter));
}
public LazyIterable selectInstancesOf(Class clazz)
{
return LazyIterate.selectInstancesOf(this, clazz);
}
public LazyIterable collect(Function super T, ? extends V> function)
{
return LazyIterate.collect(this, function);
}
public LazyBooleanIterable collectBoolean(BooleanFunction super T> booleanFunction)
{
return new CollectBooleanIterable(this, booleanFunction);
}
public LazyByteIterable collectByte(ByteFunction super T> byteFunction)
{
return new CollectByteIterable(this, byteFunction);
}
public LazyCharIterable collectChar(CharFunction super T> charFunction)
{
return new CollectCharIterable(this, charFunction);
}
public LazyDoubleIterable collectDouble(DoubleFunction super T> doubleFunction)
{
return new CollectDoubleIterable(this, doubleFunction);
}
public LazyFloatIterable collectFloat(FloatFunction super T> floatFunction)
{
return new CollectFloatIterable(this, floatFunction);
}
public LazyIntIterable collectInt(IntFunction super T> intFunction)
{
return new CollectIntIterable(this, intFunction);
}
public LazyLongIterable collectLong(LongFunction super T> longFunction)
{
return new CollectLongIterable(this, longFunction);
}
public LazyShortIterable collectShort(ShortFunction super T> shortFunction)
{
return new CollectShortIterable(this, shortFunction);
}
public LazyIterable collectWith(Function2 super T, ? super P, ? extends V> function, P parameter)
{
return LazyIterate.collect(this, Functions.bind(function, parameter));
}
public LazyIterable flatCollect(Function super T, ? extends Iterable> function)
{
return LazyIterate.flatCollect(this, function);
}
public LazyIterable concatenate(Iterable iterable)
{
return LazyIterate.concatenate(this, iterable);
}
public LazyIterable collectIf(Predicate super T> predicate, Function super T, ? extends V> function)
{
return LazyIterate.collectIf(this, predicate, function);
}
public LazyIterable take(int count)
{
return LazyIterate.take(this, count);
}
public LazyIterable drop(int count)
{
return LazyIterate.drop(this, count);
}
public LazyIterable distinct()
{
return LazyIterate.distinct(this);
}
public MutableStack toStack()
{
return ArrayStack.newStack(this);
}
public Multimap groupBy(Function super T, ? extends V> function)
{
return this.groupBy(function, FastListMultimap.newMultimap());
}
public Multimap groupByEach(Function super T, ? extends Iterable> function)
{
return this.groupByEach(function, FastListMultimap.newMultimap());
}
public MapIterable groupByUniqueKey(Function super T, ? extends V> function)
{
return this.groupByUniqueKey(function, UnifiedMap.newMap());
}
public LazyIterable> zip(Iterable that)
{
return LazyIterate.zip(this, that);
}
public LazyIterable> zipWithIndex()
{
return LazyIterate.zipWithIndex(this);
}
public LazyIterable> chunk(int size)
{
return LazyIterate.chunk(this, size);
}
public LazyIterable tap(Procedure super T> procedure)
{
return LazyIterate.tap(this, procedure);
}
public MapIterable aggregateInPlaceBy(
Function super T, ? extends K> groupBy,
Function0 extends V> zeroValueFactory,
Procedure2 super V, ? super T> mutatingAggregator)
{
MutableMap map = UnifiedMap.newMap();
this.forEach(new MutatingAggregationProcedure(map, groupBy, zeroValueFactory, mutatingAggregator));
return map;
}
public MapIterable aggregateBy(
Function super T, ? extends K> groupBy,
Function0 extends V> zeroValueFactory,
Function2 super V, ? super T, ? extends V> nonMutatingAggregator)
{
MutableMap map = UnifiedMap.newMap();
this.forEach(new NonMutatingAggregationProcedure(map, groupBy, zeroValueFactory, nonMutatingAggregator));
return map;
}
}