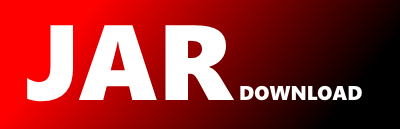
org.eclipse.collections.impl.utility.Iterate Maven / Gradle / Ivy
/*
* Copyright (c) 2015 Goldman Sachs.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.utility;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.RandomAccess;
import java.util.SortedSet;
import org.eclipse.collections.api.InternalIterable;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function0;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.function.Function3;
import org.eclipse.collections.api.block.function.primitive.BooleanFunction;
import org.eclipse.collections.api.block.function.primitive.ByteFunction;
import org.eclipse.collections.api.block.function.primitive.CharFunction;
import org.eclipse.collections.api.block.function.primitive.DoubleFunction;
import org.eclipse.collections.api.block.function.primitive.DoubleObjectToDoubleFunction;
import org.eclipse.collections.api.block.function.primitive.FloatFunction;
import org.eclipse.collections.api.block.function.primitive.FloatObjectToFloatFunction;
import org.eclipse.collections.api.block.function.primitive.IntFunction;
import org.eclipse.collections.api.block.function.primitive.IntObjectToIntFunction;
import org.eclipse.collections.api.block.function.primitive.LongFunction;
import org.eclipse.collections.api.block.function.primitive.LongObjectToLongFunction;
import org.eclipse.collections.api.block.function.primitive.ShortFunction;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.block.procedure.Procedure;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.block.procedure.primitive.ObjectIntProcedure;
import org.eclipse.collections.api.collection.MutableCollection;
import org.eclipse.collections.api.collection.primitive.MutableBooleanCollection;
import org.eclipse.collections.api.collection.primitive.MutableByteCollection;
import org.eclipse.collections.api.collection.primitive.MutableCharCollection;
import org.eclipse.collections.api.collection.primitive.MutableDoubleCollection;
import org.eclipse.collections.api.collection.primitive.MutableFloatCollection;
import org.eclipse.collections.api.collection.primitive.MutableIntCollection;
import org.eclipse.collections.api.collection.primitive.MutableLongCollection;
import org.eclipse.collections.api.collection.primitive.MutableShortCollection;
import org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.api.map.MutableMap;
import org.eclipse.collections.api.map.primitive.ObjectDoubleMap;
import org.eclipse.collections.api.map.primitive.ObjectLongMap;
import org.eclipse.collections.api.multimap.MutableMultimap;
import org.eclipse.collections.api.multimap.bag.BagMultimap;
import org.eclipse.collections.api.multimap.list.ListMultimap;
import org.eclipse.collections.api.multimap.set.SetMultimap;
import org.eclipse.collections.api.partition.PartitionIterable;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.api.tuple.Twin;
import org.eclipse.collections.impl.block.factory.Comparators;
import org.eclipse.collections.impl.block.factory.Functions;
import org.eclipse.collections.impl.block.factory.Predicates;
import org.eclipse.collections.impl.block.factory.Procedures2;
import org.eclipse.collections.impl.block.procedure.MapCollectProcedure;
import org.eclipse.collections.impl.block.procedure.MaxComparatorProcedure;
import org.eclipse.collections.impl.block.procedure.MinComparatorProcedure;
import org.eclipse.collections.impl.factory.Lists;
import org.eclipse.collections.impl.list.mutable.FastList;
import org.eclipse.collections.impl.map.mutable.UnifiedMap;
import org.eclipse.collections.impl.multimap.bag.HashBagMultimap;
import org.eclipse.collections.impl.multimap.list.FastListMultimap;
import org.eclipse.collections.impl.multimap.set.UnifiedSetMultimap;
import org.eclipse.collections.impl.utility.internal.DefaultSpeciesNewStrategy;
import org.eclipse.collections.impl.utility.internal.IterableIterate;
import org.eclipse.collections.impl.utility.internal.RandomAccessListIterate;
/**
* The Iterate utility class acts as a router to other utility classes to provide optimized iteration pattern
* implementations based on the type of iterable. The lowest common denominator used will normally be IterableIterate.
* Iterate can be used when a JDK interface is the only type available to the developer, as it can
* determine the best way to iterate based on instanceof checks.
*
* @since 1.0
*/
public final class Iterate
{
private Iterate()
{
throw new AssertionError("Suppress default constructor for noninstantiability");
}
/**
* The procedure is evaluated for each element of the iterable.
*
* Example using a Java 8 lambda expression:
*
* Iterate.forEach(people, person -> LOGGER.info(person.getName());
*
*
* Example using an anonymous inner class:
*
* Iterate.forEach(people, new Procedure()
* {
* public void value(Person person)
* {
* LOGGER.info(person.getName());
* }
* });
*
*/
public static void forEach(Iterable iterable, Procedure super T> procedure)
{
if (iterable instanceof InternalIterable)
{
((InternalIterable) iterable).forEach(procedure);
}
else if (iterable instanceof ArrayList)
{
ArrayListIterate.forEach((ArrayList) iterable, procedure);
}
else if (iterable instanceof List)
{
ListIterate.forEach((List) iterable, procedure);
}
else if (iterable != null)
{
IterableIterate.forEach(iterable, procedure);
}
else
{
throw new IllegalArgumentException("Cannot perform a forEach on null");
}
}
/**
* The procedure2 is evaluated for each element of the iterable with the specified parameter passed
* as the second argument.
*
* Example using a Java 8 lambda expression:
*
* Iterate.forEachWith(people, (Person person, Person other) ->
* {
* if (other.equals(person))
* {
* LOGGER.info(person.getName());
* }
* }, fred);
*
*
*
e.g.
* Iterate.forEachWith(people, new Procedure2()
* {
* public void value(Person person, Person other)
* {
* if (person.isRelatedTo(other))
* {
* LOGGER.info(person.getName());
* }
* }
* }, fred);
*
*/
public static void forEachWith(
Iterable iterable,
Procedure2 super T, ? super P> procedure,
P parameter)
{
if (iterable instanceof InternalIterable)
{
((InternalIterable) iterable).forEachWith(procedure, parameter);
}
else if (iterable instanceof ArrayList)
{
ArrayListIterate.forEachWith((ArrayList) iterable, procedure, parameter);
}
else if (iterable instanceof List)
{
ListIterate.forEachWith((List) iterable, procedure, parameter);
}
else if (iterable != null)
{
IterableIterate.forEachWith(iterable, procedure, parameter);
}
else
{
throw new IllegalArgumentException("Cannot perform a forEachWith on null");
}
}
/**
* Iterates over a collection passing each element and the current relative int index to the specified instance of
* ObjectIntProcedure.
*
* Example using a Java 8 lambda expression:
*
* Iterate.forEachWithIndex(people, (Person person, int index) -> LOGGER.info("Index: " + index + " person: " + person.getName()));
*
*
* Example using anonymous inner class:
*
* Iterate.forEachWithIndex(people, new ObjectIntProcedure()
* {
* public void value(Person person, int index)
* {
* LOGGER.info("Index: " + index + " person: " + person.getName());
* }
* });
*
*/
public static void forEachWithIndex(Iterable iterable, ObjectIntProcedure super T> objectIntProcedure)
{
if (iterable instanceof InternalIterable)
{
((InternalIterable) iterable).forEachWithIndex(objectIntProcedure);
}
else if (iterable instanceof ArrayList)
{
ArrayListIterate.forEachWithIndex((ArrayList) iterable, objectIntProcedure);
}
else if (iterable instanceof List)
{
ListIterate.forEachWithIndex((List) iterable, objectIntProcedure);
}
else if (iterable != null)
{
IterableIterate.forEachWithIndex(iterable, objectIntProcedure);
}
else
{
throw new IllegalArgumentException("Cannot perform a forEachWithIndex on null");
}
}
/**
* Returns a new collection with only the elements that evaluated to true for the specified predicate.
*
* Example using a Java 8 lambda expression:
*
* Collection<Person> selected =
* Iterate.select(people, person -> person.getAddress().getCity().equals("Metuchen"));
*
*
* Example using an anonymous inner class:
*
e.g.
* Collection<Person> selected =
* Iterate.select(people, new Predicate<Person>()
* {
* public boolean value(Person person)
* {
* return person.getAddress().getCity().equals("Metuchen");
* }
* });
*
*/
public static Collection select(Iterable iterable, Predicate super T> predicate)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).select(predicate);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.select((ArrayList) iterable, predicate);
}
if (iterable instanceof List)
{
return ListIterate.select((List) iterable, predicate);
}
if (iterable instanceof Collection)
{
return IterableIterate.select(
iterable,
predicate,
DefaultSpeciesNewStrategy.INSTANCE.speciesNew((Collection) iterable));
}
if (iterable != null)
{
return IterableIterate.select(iterable, predicate);
}
throw new IllegalArgumentException("Cannot perform a select on null");
}
/**
* Returns a new collection with only elements that evaluated to true for the specified predicate and parameter.
*
* Example using a Java 8 lambda expression:
*
* Collection<Person> selected =
* Iterate.selectWith(people, (Person person, Integer age) -> person.getAge() >= age, Integer.valueOf(18));
*
*
* Example using an anonymous inner class:
*
* Collection<Person> selected =
* Iterate.selectWith(people, new Predicate2<Person, Integer>()
* {
* public boolean accept(Person person, Integer age)
* {
* return person.getAge() >= age;
* }
* }, Integer.valueOf(18));
*
*/
public static Collection selectWith(
Iterable iterable,
Predicate2 super T, ? super IV> predicate,
IV parameter)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).selectWith(predicate, parameter);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.selectWith((ArrayList) iterable, predicate, parameter);
}
if (iterable instanceof List)
{
return ListIterate.selectWith((List) iterable, predicate, parameter);
}
if (iterable instanceof Collection)
{
return IterableIterate.selectWith(
iterable,
predicate,
parameter,
DefaultSpeciesNewStrategy.INSTANCE.speciesNew((Collection) iterable));
}
if (iterable != null)
{
return IterableIterate.selectWith(iterable, predicate, parameter, FastList.newList());
}
throw new IllegalArgumentException("Cannot perform a selectWith on null");
}
/**
* Filters a collection into two separate collections based on a predicate returned via a Twin.
*
* Example using a Java 8 lambda expression:
*
* Twin<MutableList<Person>>selectedRejected =
* Iterate.selectAndRejectWith(people, (Person person, String lastName) -> lastName.equals(person.getLastName()), "Mason");
*
*
* Example using an anonymous inner class:
*
* Twin<MutableList<Person>>selectedRejected =
* Iterate.selectAndRejectWith(people, new Predicate2<String, String>()
* {
* public boolean accept(Person person, String lastName)
* {
* return lastName.equals(person.getLastName());
* }
* }, "Mason");
*
*/
public static Twin> selectAndRejectWith(
Iterable iterable,
Predicate2 super T, ? super IV> predicate,
IV injectedValue)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).selectAndRejectWith(predicate, injectedValue);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.selectAndRejectWith((ArrayList) iterable, predicate, injectedValue);
}
if (iterable instanceof List)
{
return ListIterate.selectAndRejectWith((List) iterable, predicate, injectedValue);
}
if (iterable != null)
{
return IterableIterate.selectAndRejectWith(iterable, predicate, injectedValue);
}
throw new IllegalArgumentException("Cannot perform a selectAndRejectWith on null");
}
/**
* Filters a collection into a PartitionIterable based on a predicate.
*
* Example using a Java 8 lambda expression:
*
* PartitionIterable<Person> newYorkersAndNonNewYorkers =
* Iterate.partition(people, person -> person.getAddress().getState().getName().equals("New York"));
*
*
* Example using an anonymous inner class:
*
* PartitionIterable<Person> newYorkersAndNonNewYorkers =
* Iterate.partition(people, new Predicate<Person>()
* {
* public boolean value(Person person)
* {
* return person.getAddress().getState().getName().equals("New York");
* }
* });
*
*/
public static PartitionIterable partition(Iterable iterable, Predicate super T> predicate)
{
if (iterable instanceof RichIterable>)
{
return ((RichIterable) iterable).partition(predicate);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.partition((ArrayList) iterable, predicate);
}
if (iterable instanceof List)
{
return ListIterate.partition((List) iterable, predicate);
}
if (iterable != null)
{
return IterableIterate.partition(iterable, predicate);
}
throw new IllegalArgumentException("Cannot perform a partition on null");
}
/**
* Filters a collection into a PartitionIterable based on a predicate.
*
* Example using a Java 8 lambda expression:
*
* PartitionIterable<Person>> newYorkersAndNonNewYorkers =
* Iterate.partitionWith(people, (Person person, String state) -> person.getAddress().getState().getName().equals(state), "New York");
*
*
* Example using an anonymous inner class:
*
* PartitionIterable<Person> newYorkersAndNonNewYorkers =
* Iterate.partitionWith(people, new Predicate<Person, String>()
* {
* public boolean value(Person person, String state)
* {
* return person.getAddress().getState().getName().equals(state);
* }
* }, "New York");
*
*
* @since 5.0.
*/
public static PartitionIterable partitionWith(Iterable iterable, Predicate2 super T, ? super P> predicate, P parameter)
{
if (iterable instanceof RichIterable>)
{
return ((RichIterable) iterable).partitionWith(predicate, parameter);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.partitionWith((ArrayList) iterable, predicate, parameter);
}
if (iterable instanceof List)
{
return ListIterate.partitionWith((List) iterable, predicate, parameter);
}
if (iterable != null)
{
return IterableIterate.partitionWith(iterable, predicate, parameter);
}
throw new IllegalArgumentException("Cannot perform a partition on null");
}
/**
* Returns a new collection with only the elements that are instances of the Class {@code clazz}.
*/
public static Collection selectInstancesOf(Iterable> iterable, Class clazz)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection>) iterable).selectInstancesOf(clazz);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.selectInstancesOf((ArrayList>) iterable, clazz);
}
if (iterable instanceof List)
{
return ListIterate.selectInstancesOf((List>) iterable, clazz);
}
if (iterable instanceof Collection)
{
return IterableIterate.selectInstancesOf(
iterable,
clazz,
DefaultSpeciesNewStrategy.INSTANCE.speciesNew((Collection>) iterable));
}
if (iterable != null)
{
return IterableIterate.selectInstancesOf(iterable, clazz);
}
throw new IllegalArgumentException("Cannot perform a selectInstancesOf on null");
}
/**
* Returns the total number of elements that evaluate to true for the specified predicate.
*
* Example using a Java 8 lambda expression:
*
* int count = Iterate.count(people, person -> person.getAddress().getState().getName().equals("New York"));
*
*
* Example using anonymous inner class
*
* int count = Iterate.count(people, new Predicate<Person>()
* {
* public boolean accept(Person person)
* {
* return person.getAddress().getState().getName().equals("New York");
* }
* });
*
*/
public static int count(Iterable iterable, Predicate super T> predicate)
{
if (iterable instanceof RichIterable)
{
return ((RichIterable) iterable).count(predicate);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.count((ArrayList) iterable, predicate);
}
if (iterable instanceof List)
{
return ListIterate.count((List) iterable, predicate);
}
if (iterable != null)
{
return IterableIterate.count(iterable, predicate);
}
throw new IllegalArgumentException("Cannot get a count from null");
}
/**
* Returns the total number of elements that evaluate to true for the specified predicate2 and parameter.
*
*
e.g.
* return Iterate.countWith(lastNames, Predicates2.equal(), "Smith");
*
*/
public static int countWith(
Iterable iterable,
Predicate2 super T, ? super IV> predicate,
IV injectedValue)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).countWith(predicate, injectedValue);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.countWith((ArrayList) iterable, predicate, injectedValue);
}
if (iterable instanceof List)
{
return ListIterate.countWith((List) iterable, predicate, injectedValue);
}
if (iterable != null)
{
return IterableIterate.countWith(iterable, predicate, injectedValue);
}
throw new IllegalArgumentException("Cannot get a count from null");
}
/**
* @see RichIterable#collectIf(Predicate, Function)
*/
public static Collection collectIf(
Iterable iterable,
Predicate super T> predicate,
Function super T, ? extends V> function)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectIf(predicate, function);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectIf((ArrayList) iterable, predicate, function);
}
if (iterable instanceof List)
{
return ListIterate.collectIf((List) iterable, predicate, function);
}
if (iterable instanceof Collection)
{
return IterableIterate.collectIf(
iterable,
predicate,
function,
DefaultSpeciesNewStrategy.INSTANCE.speciesNew((Collection) iterable));
}
if (iterable != null)
{
return IterableIterate.collectIf(iterable, predicate, function);
}
throw new IllegalArgumentException("Cannot perform a collectIf on null");
}
/**
* @see RichIterable#collectIf(Predicate, Function, Collection)
*/
public static > R collectIf(
Iterable iterable,
Predicate super T> predicate,
Function super T, ? extends V> function,
R target)
{
if (iterable instanceof RichIterable)
{
return ((RichIterable) iterable).collectIf(predicate, function, target);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectIf((ArrayList) iterable, predicate, function, target);
}
if (iterable instanceof List)
{
return ListIterate.collectIf((List) iterable, predicate, function, target);
}
if (iterable != null)
{
return IterableIterate.collectIf(iterable, predicate, function, target);
}
throw new IllegalArgumentException("Cannot perform a collectIf on null");
}
/**
* Same as the select method with two parameters but uses the specified target collection
*
* Example using Java 8 lambda:
*
* MutableList<Person> selected =
* Iterate.select(people, person -> person.person.getLastName().equals("Smith"), FastList.newList());
*
*
* Example using anonymous inner class:
*
* MutableList<Person> selected =
* Iterate.select(people, new Predicate<Person>()
* {
* public boolean accept(Person person)
* {
* return person.person.getLastName().equals("Smith");
* }
* }, FastList.newList());
*
*
* Example using Predicates factory:
*
* MutableList<Person> selected = Iterate.select(collection, Predicates.attributeEqual("lastName", "Smith"), FastList.newList());
*
*/
public static > R select(
Iterable iterable,
Predicate super T> predicate,
R targetCollection)
{
if (iterable instanceof RichIterable)
{
return ((RichIterable) iterable).select(predicate, targetCollection);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.select((ArrayList) iterable, predicate, targetCollection);
}
if (iterable instanceof List)
{
return ListIterate.select((List) iterable, predicate, targetCollection);
}
if (iterable != null)
{
return IterableIterate.select(iterable, predicate, targetCollection);
}
throw new IllegalArgumentException("Cannot perform a select on null");
}
/**
* Same as the selectWith method with two parameters but uses the specified target collection.
*/
public static > R selectWith(
Iterable iterable,
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
if (iterable instanceof RichIterable)
{
return ((RichIterable) iterable).selectWith(predicate, parameter, targetCollection);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.selectWith((ArrayList) iterable, predicate, parameter, targetCollection);
}
if (iterable instanceof List)
{
return ListIterate.selectWith((List) iterable, predicate, parameter, targetCollection);
}
if (iterable != null)
{
return IterableIterate.selectWith(iterable, predicate, parameter, targetCollection);
}
throw new IllegalArgumentException("Cannot perform a selectWith on null");
}
/**
* Returns the first {@code count} elements of the iterable
* or all the elements in the iterable if {@code count} is greater than the length of
* the iterable.
*
* @param iterable the collection to take from.
* @param count the number of items to take.
* @return a new list with the items take from the given collection.
* @throws IllegalArgumentException if {@code count} is less than zero
*/
public static Collection take(Iterable iterable, int count)
{
if (iterable instanceof ArrayList)
{
return ArrayListIterate.take((ArrayList) iterable, count);
}
if (iterable instanceof RandomAccess)
{
return RandomAccessListIterate.take((List) iterable, count);
}
if (iterable instanceof Collection)
{
return IterableIterate.take(
iterable,
count,
DefaultSpeciesNewStrategy.INSTANCE.speciesNew((Collection) iterable, count));
}
if (iterable != null)
{
return IterableIterate.take(iterable, count);
}
throw new IllegalArgumentException("Cannot perform a take on null");
}
/**
* Returns a collection without the first {@code count} elements of the iterable
* or an empty iterable if the {@code count} is greater than the length of the iterable.
*
* @param iterable the collection to drop from.
* @param count the number of items to drop.
* @return a new list with the items dropped from the given collection.
* @throws IllegalArgumentException if {@code count} is less than zero
*/
public static Collection drop(Iterable iterable, int count)
{
if (iterable instanceof ArrayList)
{
return ArrayListIterate.drop((ArrayList) iterable, count);
}
if (iterable instanceof RandomAccess)
{
return RandomAccessListIterate.drop((List) iterable, count);
}
if (iterable instanceof Collection)
{
return IterableIterate.drop(
iterable,
count,
DefaultSpeciesNewStrategy.INSTANCE.speciesNew((Collection) iterable, count));
}
if (iterable != null)
{
return IterableIterate.drop(iterable, count);
}
throw new IllegalArgumentException("Cannot perform a drop on null");
}
/**
* Returns all elements of the iterable that evaluate to false for the specified predicate.
*
* Example using Java 8 lambda:
*
* Collection<Person> rejected =
* Iterate.reject(people, person -> person.person.getLastName().equals("Smith"));
*
*
* Example using anonymous inner class:
*
* Collection<Person> rejected =
* Iterate.reject(people,
* new Predicate<Person>()
* {
* public boolean accept(Person person)
* {
* return person.person.getLastName().equals("Smith");
* }
* });
*
*
* Example using Predicates factory:
*
* Collection<Person> rejected =
* Iterate.reject(people, Predicates.attributeEqual("lastName", "Smith"));
*
*/
public static Collection reject(Iterable iterable, Predicate super T> predicate)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).reject(predicate);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.reject((ArrayList) iterable, predicate);
}
if (iterable instanceof List)
{
return ListIterate.reject((List) iterable, predicate);
}
if (iterable instanceof Collection)
{
return IterableIterate.reject(
iterable,
predicate,
DefaultSpeciesNewStrategy.INSTANCE.speciesNew((Collection) iterable));
}
if (iterable != null)
{
return IterableIterate.reject(iterable, predicate);
}
throw new IllegalArgumentException("Cannot perform a reject on null");
}
/**
* SortThis is a mutating method. The List passed in is also returned.
*/
public static , L extends List> L sortThis(L list)
{
if (list instanceof MutableList>)
{
((MutableList) list).sortThis();
}
else if (list instanceof ArrayList)
{
ArrayListIterate.sortThis((ArrayList) list);
}
else
{
if (list.size() > 1)
{
Collections.sort(list);
}
}
return list;
}
/**
* SortThis is a mutating method. The List passed in is also returned.
*/
public static > L sortThis(L list, Comparator super T> comparator)
{
if (list instanceof MutableList)
{
((MutableList) list).sortThis(comparator);
}
else if (list instanceof ArrayList)
{
ArrayListIterate.sortThis((ArrayList) list, comparator);
}
else
{
if (list.size() > 1)
{
Collections.sort(list, comparator);
}
}
return list;
}
/**
* SortThis is a mutating method. The List passed in is also returned.
*/
public static > L sortThis(L list, final Predicate2 super T, ? super T> predicate)
{
return Iterate.sortThis(list, new Comparator()
{
public int compare(T o1, T o2)
{
if (predicate.accept(o1, o2))
{
return -1;
}
if (predicate.accept(o2, o1))
{
return 1;
}
return 0;
}
});
}
/**
* Sort the list by comparing an attribute defined by the function.
* SortThisBy is a mutating method. The List passed in is also returned.
*/
public static , L extends List> L sortThisBy(L list, Function super T, ? extends V> function)
{
return Iterate.sortThis(list, Comparators.byFunction(function));
}
/**
* Removes all elements from the iterable that evaluate to true for the specified predicate.
*/
public static boolean removeIf(Iterable iterable, Predicate super T> predicate)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).removeIf(predicate);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.removeIf((ArrayList) iterable, predicate);
}
if (iterable instanceof List)
{
return ListIterate.removeIf((List) iterable, predicate);
}
if (iterable != null)
{
return IterableIterate.removeIf(iterable, predicate);
}
throw new IllegalArgumentException("Cannot perform a remove on null");
}
/**
* Removes all elements of the iterable that evaluate to true for the specified predicate2 and parameter.
*/
public static boolean removeIfWith(
Iterable iterable,
Predicate2 super T, ? super P> predicate,
P parameter)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).removeIfWith(predicate, parameter);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.removeIfWith((ArrayList) iterable, predicate, parameter);
}
if (iterable instanceof List)
{
return ListIterate.removeIfWith((List) iterable, predicate, parameter);
}
if (iterable != null)
{
return IterableIterate.removeIfWith(iterable, predicate, parameter);
}
throw new IllegalArgumentException("Cannot perform a remove on null");
}
/**
* Similar to {@link #reject(Iterable, Predicate)}, except with an evaluation parameter for the second generic argument in {@link Predicate2}.
*
* Example using a Java 8 lambda expression:
*
* Collection<Person> rejected =
* Iterate.rejectWith(people, (Person person, Integer age) -> person.getAge() >= age, Integer.valueOf(18));
*
*
* Example using an anonymous inner class:
*
* Collection<Person> rejected =
* Iterate.rejectWith(people, new Predicate2<Person, Integer>()
* {
* public boolean accept(Person person, Integer age)
* {
* return person.getAge() >= age;
* }
* }, Integer.valueOf(18));
*
*/
public static Collection rejectWith(
Iterable iterable,
Predicate2 super T, ? super P> predicate,
P parameter)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).rejectWith(predicate, parameter);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.rejectWith((ArrayList) iterable, predicate, parameter);
}
if (iterable instanceof List)
{
return ListIterate.rejectWith((List) iterable, predicate, parameter);
}
if (iterable instanceof Collection)
{
return IterableIterate.rejectWith(
iterable,
predicate,
parameter,
DefaultSpeciesNewStrategy.INSTANCE.speciesNew((Collection) iterable));
}
if (iterable != null)
{
return IterableIterate.rejectWith(iterable, predicate, parameter, FastList.newList());
}
throw new IllegalArgumentException("Cannot perform a rejectWith on null");
}
/**
* Same as the reject method with one parameter but uses the specified target collection for the results.
*
* Example using Java 8 lambda:
*
* MutableList<Person> rejected =
* Iterate.reject(people, person -> person.person.getLastName().equals("Smith"), FastList.newList());
*
*
* Example using anonymous inner class:
*
* MutableList<Person> rejected =
* Iterate.reject(people,
* new Predicate<Person>()
* {
* public boolean accept(Person person)
* {
* return person.person.getLastName().equals("Smith");
* }
* }, FastList.newList());
*
*
* Example using Predicates factory:
*
* MutableList<Person> rejected =
* Iterate.reject(people, Predicates.attributeEqual("lastName", "Smith"), FastList.newList());
*
*/
public static > R reject(
Iterable iterable,
Predicate super T> predicate,
R targetCollection)
{
if (iterable instanceof RichIterable)
{
return ((RichIterable) iterable).reject(predicate, targetCollection);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.reject((ArrayList) iterable, predicate, targetCollection);
}
if (iterable instanceof List)
{
return ListIterate.reject((List) iterable, predicate, targetCollection);
}
if (iterable != null)
{
return IterableIterate.reject(iterable, predicate, targetCollection);
}
throw new IllegalArgumentException("Cannot perform a reject on null");
}
/**
* Same as the reject method with two parameters but uses the specified target collection.
*/
public static > R rejectWith(
Iterable iterable,
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
if (iterable instanceof RichIterable)
{
return ((RichIterable) iterable).rejectWith(predicate, parameter, targetCollection);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.rejectWith(
(ArrayList) iterable,
predicate,
parameter,
targetCollection);
}
if (iterable instanceof RandomAccess)
{
return RandomAccessListIterate.rejectWith((List) iterable, predicate, parameter, targetCollection);
}
if (iterable != null)
{
return IterableIterate.rejectWith(iterable, predicate, parameter, targetCollection);
}
throw new IllegalArgumentException("Cannot perform a rejectWith on null");
}
/**
* Add all elements from the source Iterable to the target collection, return the target collection.
*/
public static > R addAllTo(Iterable extends T> iterable, R targetCollection)
{
Iterate.addAllIterable(iterable, targetCollection);
return targetCollection;
}
/**
* Add all elements from the source Iterable to the target collection, returns true if any element was added.
*/
public static boolean addAllIterable(Iterable extends T> iterable, Collection targetCollection)
{
if (iterable == null)
{
throw new NullPointerException();
}
if (iterable instanceof Collection>)
{
return targetCollection.addAll((Collection) iterable);
}
int oldSize = targetCollection.size();
Iterate.forEachWith(iterable, Procedures2.addToCollection(), targetCollection);
return targetCollection.size() != oldSize;
}
/**
* Remove all elements present in Iterable from the target collection, return the target collection.
*/
public static > R removeAllFrom(Iterable extends T> iterable, R targetCollection)
{
Iterate.removeAllIterable(iterable, targetCollection);
return targetCollection;
}
/**
* Remove all elements present in Iterable from the target collection, returns true if any element was removed.
*/
public static boolean removeAllIterable(Iterable extends T> iterable, Collection targetCollection)
{
if (iterable == null)
{
throw new NullPointerException();
}
if (iterable instanceof Collection>)
{
return targetCollection.removeAll((Collection) iterable);
}
int oldSize = targetCollection.size();
Iterate.forEachWith(iterable, Procedures2.removeFromCollection(), targetCollection);
return targetCollection.size() != oldSize;
}
/**
* Returns a new collection with the results of applying the specified function for each element of the iterable.
*
* Example using a Java 8 lambda expression:
*
* Collection<String> names =
* Iterate.collect(people, person -> person.getFirstName() + " " + person.getLastName());
*
*
* Example using an anonymous inner class:
*
* Collection<String> names =
* Iterate.collect(people,
* new Function<Person, String>()
* {
* public String value(Person person)
* {
* return person.getFirstName() + " " + person.getLastName();
* }
* });
*
*/
public static Collection collect(
Iterable iterable,
Function super T, ? extends V> function)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collect(function);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collect((ArrayList) iterable, function);
}
if (iterable instanceof RandomAccess)
{
return RandomAccessListIterate.collect((List) iterable, function);
}
if (iterable instanceof Collection)
{
return IterableIterate.collect(
iterable,
function,
DefaultSpeciesNewStrategy.INSTANCE.speciesNew(
(Collection) iterable,
((Collection) iterable).size()));
}
if (iterable != null)
{
return IterableIterate.collect(iterable, function);
}
throw new IllegalArgumentException("Cannot perform a collect on null");
}
/**
* Same as the {@link #collect(Iterable, Function)} method with two parameters, except that the results are gathered into the specified
* targetCollection
*
* Example using a Java 8 lambda expression:
*
* MutableList<String> names =
* Iterate.collect(people, person -> person.getFirstName() + " " + person.getLastName(), FastList.newList());
*
*
* Example using an anonymous inner class:
*
* MutableList<String> names =
* Iterate.collect(people,
* new Function<Person, String>()
* {
* public String value(Person person)
* {
* return person.getFirstName() + " " + person.getLastName();
* }
* }, FastList.newList());
*
*/
public static > R collect(
Iterable iterable,
Function super T, ? extends A> function,
R targetCollection)
{
if (iterable instanceof RichIterable)
{
return ((RichIterable) iterable).collect(function, targetCollection);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collect((ArrayList) iterable, function, targetCollection);
}
if (iterable instanceof RandomAccess)
{
return RandomAccessListIterate.collect((List) iterable, function, targetCollection);
}
if (iterable != null)
{
return IterableIterate.collect(iterable, function, targetCollection);
}
throw new IllegalArgumentException("Cannot perform a collect on null");
}
/**
* Returns a new primitive {@code boolean} collection with the results of applying the specified booleanFunction for each element of the iterable.
*
* Example using Java 8 lambda:
*
* MutableBooleanCollection voters =
* Iterable.collectBoolean(people, person -> person.canVote());
*
*
* Example using anonymous inner class:
*
* MutableBooleanCollection voters =
* Iterate.collectBoolean(people,
* new BooleanFunction<Person>()
* {
* public boolean booleanValueOf(Person person)
* {
* return person.canVote();
* }
* });
*
*/
public static MutableBooleanCollection collectBoolean(
Iterable iterable,
BooleanFunction super T> booleanFunction)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectBoolean(booleanFunction);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectBoolean((ArrayList) iterable, booleanFunction);
}
if (iterable instanceof List)
{
return ListIterate.collectBoolean((List) iterable, booleanFunction);
}
if (iterable != null)
{
return IterableIterate.collectBoolean(iterable, booleanFunction);
}
throw new IllegalArgumentException("Cannot perform a collectBoolean on null");
}
/**
* Same as {@link #collectBoolean(Iterable, BooleanFunction)}, except that the results are gathered into the specified {@code target}
* collection.
*
* Example using Java 8 lambda:
*
* BooleanArrayList voters =
* Iterable.collectBoolean(people, person -> person.canVote(), new BooleanArrayList());
*
*
* Example using an anonymous inner class:
*
* BooleanArrayList voters =
* Iterate.collectBoolean(people,
* new BooleanFunction<Person>()
* {
* public boolean booleanValueOf(Person person)
* {
* return person.canVote();
* }
* }, new BooleanArrayList());
*
*/
public static R collectBoolean(
Iterable iterable,
BooleanFunction super T> booleanFunction,
R target)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectBoolean(booleanFunction, target);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectBoolean((ArrayList) iterable, booleanFunction, target);
}
if (iterable instanceof List)
{
return ListIterate.collectBoolean((List) iterable, booleanFunction, target);
}
if (iterable != null)
{
return IterableIterate.collectBoolean(iterable, booleanFunction, target);
}
throw new IllegalArgumentException("Cannot perform a collectBoolean on null");
}
/**
* Returns a new {@code byte} collection with the results of applying the specified byteFunction for each element of the iterable.
*
* Example using Java 8 lambda:
*
* MutableByteCollection bytes =
* Iterate.collectByte(people, person -> person.getCode());
*
*
* Example using anonymous inner class:
*
* MutableByteCollection bytes =
* Iterate.collectByte(people,
* new ByteFunction<Person>()
* {
* public byte byteValueOf(Person person)
* {
* return person.getCode();
* }
* });
*
*/
public static MutableByteCollection collectByte(
Iterable iterable,
ByteFunction super T> byteFunction)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectByte(byteFunction);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectByte((ArrayList) iterable, byteFunction);
}
if (iterable instanceof List)
{
return ListIterate.collectByte((List) iterable, byteFunction);
}
if (iterable != null)
{
return IterableIterate.collectByte(iterable, byteFunction);
}
throw new IllegalArgumentException("Cannot perform a collectByte on null");
}
/**
* Same as {@link #collectByte(Iterable, ByteFunction)}, except that the results are gathered into the specified {@code target}
* collection.
*
* Example using a Java 8 lambda expression:
*
* ByteArrayList bytes =
* Iterate.collectByte(people, person -> person.getCode(), new ByteArrayList());
*
*
* Example using an anonymous inner class:
*
* ByteArrayList bytes =
* Iterate.collectByte(people,
* new ByteFunction<Person>()
* {
* public byte byteValueOf(Person person)
* {
* return person.getCode();
* }
* }, new ByteArrayList());
*
*/
public static R collectByte(
Iterable iterable,
ByteFunction super T> byteFunction,
R target)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectByte(byteFunction, target);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectByte((ArrayList) iterable, byteFunction, target);
}
if (iterable instanceof List)
{
return ListIterate.collectByte((List) iterable, byteFunction, target);
}
if (iterable != null)
{
return IterableIterate.collectByte(iterable, byteFunction, target);
}
throw new IllegalArgumentException("Cannot perform a collectByte on null");
}
/**
* Returns a new {@code char} collection with the results of applying the specified charFunction for each element of the iterable.
*
* Example using Java 8 lambda:
*
* MutableCharCollection chars =
* Iterate.collectChar(people, person -> person.getMiddleInitial());
*
*
* Example using anonymous inner class:
*
* MutableCharCollection chars =
* Iterate.collectChar(people,
* new CharFunction<Person>()
* {
* public char charValueOf(Person person)
* {
* return person.getMiddleInitial();
* }
* });
*
*/
public static MutableCharCollection collectChar(
Iterable iterable,
CharFunction super T> charFunction)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectChar(charFunction);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectChar((ArrayList) iterable, charFunction);
}
if (iterable instanceof List)
{
return ListIterate.collectChar((List) iterable, charFunction);
}
if (iterable != null)
{
return IterableIterate.collectChar(iterable, charFunction);
}
throw new IllegalArgumentException("Cannot perform a collectChar on null");
}
/**
* Same as {@link #collectChar(Iterable, CharFunction)}, except that the results are gathered into the specified {@code target}
* collection.
*
*
* CharArrayList chars =
* Iterate.collectChar(people, person -> person.getMiddleInitial());
*
*
* Example using anonymous inner class:
*
* CharArrayList chars =
* Iterate.collectChar(people,
* new CharFunction<Person>()
* {
* public char charValueOf(Person person)
* {
* return person.getMiddleInitial();
* }
* }, new CharArrayList());
*
*/
public static R collectChar(
Iterable iterable,
CharFunction super T> charFunction,
R target)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectChar(charFunction, target);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectChar((ArrayList) iterable, charFunction, target);
}
if (iterable instanceof List)
{
return ListIterate.collectChar((List) iterable, charFunction, target);
}
if (iterable != null)
{
return IterableIterate.collectChar(iterable, charFunction, target);
}
throw new IllegalArgumentException("Cannot perform a collectChar on null");
}
/**
* Returns a new {@code double} collection with the results of applying the specified doubleFunction for each element of the iterable.
*
* Example using a Java 8 lambda expression:
*
* MutableDoubleCollection doubles =
* Iterate.collectDouble(people, person -> person.getMilesFromNorthPole());
*
* Example using an anonymous inner class:
*
* MutableDoubleCollection doubles =
* Iterate.collectDouble(people,
* new DoubleFunction<Person>()
* {
* public double doubleValueOf(Person person)
* {
* return person.getMilesFromNorthPole();
* }
* });
*
*/
public static MutableDoubleCollection collectDouble(
Iterable iterable,
DoubleFunction super T> doubleFunction)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectDouble(doubleFunction);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectDouble((ArrayList) iterable, doubleFunction);
}
if (iterable instanceof List)
{
return ListIterate.collectDouble((List) iterable, doubleFunction);
}
if (iterable != null)
{
return IterableIterate.collectDouble(iterable, doubleFunction);
}
throw new IllegalArgumentException("Cannot perform a collectDouble on null");
}
/**
* Same as {@link #collectDouble(Iterable, DoubleFunction)}, except that the results are gathered into the specified {@code target}
* collection.
*
* Example using a Java 8 lambda expression:
*
* DoubleArrayList doubles =
* Iterate.collectDouble(people, person -> person.getMilesFromNorthPole());
*
*
* Example using an anonymous inner class:
*
* DoubleArrayList doubles =
* Iterate.collectDouble(people,
* new DoubleFunction<Person>()
* {
* public double doubleValueOf(Person person)
* {
* return person.getMilesFromNorthPole();
* }
* }, new DoubleArrayList());
*
*/
public static R collectDouble(
Iterable iterable,
DoubleFunction super T> doubleFunction,
R target)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectDouble(doubleFunction, target);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectDouble((ArrayList) iterable, doubleFunction, target);
}
if (iterable instanceof List)
{
return ListIterate.collectDouble((List) iterable, doubleFunction, target);
}
if (iterable != null)
{
return IterableIterate.collectDouble(iterable, doubleFunction, target);
}
throw new IllegalArgumentException("Cannot perform a collectDouble on null");
}
/**
* Returns a new {@code float} collection with the results of applying the specified floatFunction for each element of the iterable.
*
* Example using a Java 8 lambda expression:
*
* MutableFloatCollection floats =
* Iterate.collectFloat(people, person -> person.getHeightInInches());
*
*
* Example using an anonymous inner class:
*
* MutableFloatCollection floats =
* Iterate.collectFloat(people,
* new FloatFunction<Person>()
* {
* public float floatValueOf(Person person)
* {
* return person.getHeightInInches();
* }
* });
*
*/
public static MutableFloatCollection collectFloat(
Iterable iterable,
FloatFunction super T> floatFunction)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectFloat(floatFunction);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectFloat((ArrayList) iterable, floatFunction);
}
if (iterable instanceof List)
{
return ListIterate.collectFloat((List) iterable, floatFunction);
}
if (iterable != null)
{
return IterableIterate.collectFloat(iterable, floatFunction);
}
throw new IllegalArgumentException("Cannot perform a collectFloat on null");
}
/**
* Same as {@link #collectFloat(Iterable, FloatFunction)}, except that the results are gathered into the specified {@code target}
* collection.
*
* Example using a Java 8 lambda expression:
*
* FloatArrayList floats =
* Iterate.collectFloat(people, person -> person.getHeightInInches(), new FloatArrayList());
*
*
* Example using an anonymous inner class:
*
* FloatArrayList floats =
* Iterate.collectFloat(people,
* new FloatFunction<Person>()
* {
* public float floatValueOf(Person person)
* {
* return person.getHeightInInches();
* }
* }, new FloatArrayList());
*
*/
public static R collectFloat(
Iterable iterable,
FloatFunction super T> floatFunction,
R target)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectFloat(floatFunction, target);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectFloat((ArrayList) iterable, floatFunction, target);
}
if (iterable instanceof List)
{
return ListIterate.collectFloat((List) iterable, floatFunction, target);
}
if (iterable != null)
{
return IterableIterate.collectFloat(iterable, floatFunction, target);
}
throw new IllegalArgumentException("Cannot perform a collectFloat on null");
}
/**
* Returns a new {@code int} collection with the results of applying the specified intFunction for each element of the iterable.
*
* Example using a Java 8 lambda expression:
*
* MutableIntCollection ages =
* Iterate.collectInt(people, person -> person.getAge());
*
*
* Example using an anonymous inner class:
*
* MutableIntCollection ages =
* Iterate.collectInt(people,
* new IntFunction<Person>()
* {
* public int intValueOf(Person person)
* {
* return person.getAge();
* }
* });
*
*/
public static MutableIntCollection collectInt(
Iterable iterable,
IntFunction super T> intFunction)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectInt(intFunction);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectInt((ArrayList) iterable, intFunction);
}
if (iterable instanceof List)
{
return ListIterate.collectInt((List) iterable, intFunction);
}
if (iterable != null)
{
return IterableIterate.collectInt(iterable, intFunction);
}
throw new IllegalArgumentException("Cannot perform a collectInt on null");
}
/**
* Same as {@link #collectInt(Iterable, IntFunction)}, except that the results are gathered into the specified {@code target}
* collection.
*
* Example using a Java 8 lambda expression:
*
* IntArrayList ages =
* Iterate.collectInt(people, person -> person.getAge(), new IntArrayList());
*
*
* Example using an anonymous inner class:
*
* IntArrayList ages =
* Iterate.collectInt(people,
* new IntFunction<Person>()
* {
* public int intValueOf(Person person)
* {
* return person.getAge();
* }
* }, new IntArrayList());
*
*/
public static R collectInt(
Iterable iterable,
IntFunction super T> intFunction,
R target)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectInt(intFunction, target);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectInt((ArrayList) iterable, intFunction, target);
}
if (iterable instanceof List)
{
return ListIterate.collectInt((List) iterable, intFunction, target);
}
if (iterable != null)
{
return IterableIterate.collectInt(iterable, intFunction, target);
}
throw new IllegalArgumentException("Cannot perform a collectInt on null");
}
/**
* Returns a new {@code long} collection with the results of applying the specified longFunction for each element of the iterable.
*
* Example using a Java 8 lambda expression:
*
* MutableLongCollection longs =
* Iterate.collectLong(people, person -> person.getGuid());
*
*
* Example using an anonymous inner class:
*
* MutableLongCollection longs =
* Iterate.collectLong(people,
* new LongFunction<Person>()
* {
* public long longValueOf(Person person)
* {
* return person.getGuid();
* }
* });
*
*/
public static MutableLongCollection collectLong(
Iterable iterable,
LongFunction super T> longFunction)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectLong(longFunction);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectLong((ArrayList) iterable, longFunction);
}
if (iterable instanceof List)
{
return ListIterate.collectLong((List) iterable, longFunction);
}
if (iterable != null)
{
return IterableIterate.collectLong(iterable, longFunction);
}
throw new IllegalArgumentException("Cannot perform a collectLong on null");
}
/**
* Same as {@link #collectLong(Iterable, LongFunction)}, except that the results are gathered into the specified {@code target}
* collection.
*
* Example using a Java 8 lambda expression:
*
* LongArrayList longs =
* Iterate.collectLong(people, person -> person.getGuid(), new LongArrayList());
*
*
* Example using an anonymous inner class:
*
* LongArrayList longs =
* Iterate.collectLong(people,
* new LongFunction<Person>()
* {
* public long longValueOf(Person person)
* {
* return person.getGuid();
* }
* }, new LongArrayList());
*
*/
public static R collectLong(
Iterable iterable,
LongFunction super T> longFunction,
R target)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectLong(longFunction, target);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectLong((ArrayList) iterable, longFunction, target);
}
if (iterable instanceof List)
{
return ListIterate.collectLong((List) iterable, longFunction, target);
}
if (iterable != null)
{
return IterableIterate.collectLong(iterable, longFunction, target);
}
throw new IllegalArgumentException("Cannot perform a collectLong on null");
}
/**
* Returns a new {@code short} collection with the results of applying the specified shortFunction for each element of the iterable.
*
* Example using a Java 8 lambda expression:
*
* MutableShortCollection shorts =
* Iterate.collectShort(people, person -> person.getNumberOfJunkMailItemsReceivedPerMonth());
*
*
* Example using an anonymous inner class:
*
* MutableShortCollection shorts =
* Iterate.collectShort(people,
* new ShortFunction<Person>()
* {
* public short shortValueOf(Person person)
* {
* return person.getNumberOfJunkMailItemsReceivedPerMonth();
* }
* });
*
*/
public static MutableShortCollection collectShort(
Iterable iterable,
ShortFunction super T> shortFunction)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection) iterable).collectShort(shortFunction);
}
if (iterable instanceof ArrayList)
{
return ArrayListIterate.collectShort((ArrayList) iterable, shortFunction);
}
if (iterable instanceof List)
{
return ListIterate.collectShort((List) iterable, shortFunction);
}
if (iterable != null)
{
return IterableIterate.collectShort(iterable, shortFunction);
}
throw new IllegalArgumentException("Cannot perform a collectShort on null");
}
/**
* Same as {@link #collectShort(Iterable, ShortFunction)}, except that the results are gathered into the specified {@code target}
* collection.
*
* Example using a Java 8 lambda expression:
*
* ShortArrayList shorts =
* Iterate.collectShort(people, person -> person.getNumberOfJunkMailItemsReceivedPerMonth(), new ShortArrayList());
*
*
* Example using an anonymous inner class:
*
* ShortArrayList shorts =
* Iterate.collectShort(people,
* new ShortFunction<Person>()
* {
* public short shortValueOf(Person person)
* {
* return person.getNumberOfJunkMailItemsReceivedPerMonth();
* }
* }, new ShortArrayList());
*
*/
public static R collectShort(
Iterable iterable,
ShortFunction super T> shortFunction,
R target)
{
if (iterable instanceof MutableCollection)
{
return ((MutableCollection