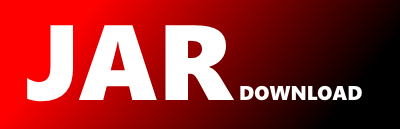
org.eclipse.collections.impl.utility.MapIterate Maven / Gradle / Ivy
/*
* Copyright (c) 2015 Goldman Sachs.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.utility;
import java.util.Collection;
import java.util.Comparator;
import java.util.Map;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function0;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.function.primitive.BooleanFunction;
import org.eclipse.collections.api.block.function.primitive.ByteFunction;
import org.eclipse.collections.api.block.function.primitive.CharFunction;
import org.eclipse.collections.api.block.function.primitive.DoubleFunction;
import org.eclipse.collections.api.block.function.primitive.FloatFunction;
import org.eclipse.collections.api.block.function.primitive.IntFunction;
import org.eclipse.collections.api.block.function.primitive.LongFunction;
import org.eclipse.collections.api.block.function.primitive.ShortFunction;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.block.procedure.Procedure;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.collection.primitive.MutableBooleanCollection;
import org.eclipse.collections.api.collection.primitive.MutableByteCollection;
import org.eclipse.collections.api.collection.primitive.MutableCharCollection;
import org.eclipse.collections.api.collection.primitive.MutableDoubleCollection;
import org.eclipse.collections.api.collection.primitive.MutableFloatCollection;
import org.eclipse.collections.api.collection.primitive.MutableIntCollection;
import org.eclipse.collections.api.collection.primitive.MutableLongCollection;
import org.eclipse.collections.api.collection.primitive.MutableShortCollection;
import org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.api.map.ImmutableMap;
import org.eclipse.collections.api.map.MapIterable;
import org.eclipse.collections.api.map.MutableMap;
import org.eclipse.collections.api.map.UnsortedMapIterable;
import org.eclipse.collections.api.map.sorted.SortedMapIterable;
import org.eclipse.collections.api.multimap.set.MutableSetMultimap;
import org.eclipse.collections.api.multimap.sortedset.MutableSortedSetMultimap;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.impl.block.factory.Predicates;
import org.eclipse.collections.impl.block.procedure.CollectProcedure;
import org.eclipse.collections.impl.block.procedure.CollectionAddProcedure;
import org.eclipse.collections.impl.block.procedure.CountProcedure;
import org.eclipse.collections.impl.block.procedure.MapEntryToProcedure2;
import org.eclipse.collections.impl.block.procedure.MapPutProcedure;
import org.eclipse.collections.impl.block.procedure.RejectProcedure;
import org.eclipse.collections.impl.block.procedure.SelectProcedure;
import org.eclipse.collections.impl.block.procedure.primitive.CollectBooleanProcedure;
import org.eclipse.collections.impl.block.procedure.primitive.CollectByteProcedure;
import org.eclipse.collections.impl.block.procedure.primitive.CollectCharProcedure;
import org.eclipse.collections.impl.block.procedure.primitive.CollectDoubleProcedure;
import org.eclipse.collections.impl.block.procedure.primitive.CollectFloatProcedure;
import org.eclipse.collections.impl.block.procedure.primitive.CollectIntProcedure;
import org.eclipse.collections.impl.block.procedure.primitive.CollectLongProcedure;
import org.eclipse.collections.impl.block.procedure.primitive.CollectShortProcedure;
import org.eclipse.collections.impl.factory.Multimaps;
import org.eclipse.collections.impl.list.mutable.FastList;
import org.eclipse.collections.impl.list.mutable.primitive.BooleanArrayList;
import org.eclipse.collections.impl.list.mutable.primitive.ByteArrayList;
import org.eclipse.collections.impl.list.mutable.primitive.CharArrayList;
import org.eclipse.collections.impl.list.mutable.primitive.DoubleArrayList;
import org.eclipse.collections.impl.list.mutable.primitive.FloatArrayList;
import org.eclipse.collections.impl.list.mutable.primitive.IntArrayList;
import org.eclipse.collections.impl.list.mutable.primitive.LongArrayList;
import org.eclipse.collections.impl.list.mutable.primitive.ShortArrayList;
import org.eclipse.collections.impl.map.mutable.MapAdapter;
import org.eclipse.collections.impl.map.mutable.UnifiedMap;
import org.eclipse.collections.impl.multimap.set.sorted.TreeSortedSetMultimap;
import org.eclipse.collections.impl.tuple.AbstractImmutableEntry;
import org.eclipse.collections.impl.tuple.Tuples;
import org.eclipse.collections.impl.utility.internal.IterableIterate;
/**
* The MapIterate class provides a few of the methods from the Smalltalk Collection Protocol. This includes:
*
* - select: -- a.k.a. filter
* - reject: -- a.k.a. not-filter
* - collect: -- a.k.a. transform, map, tear-off
* - inject:into: -- closely related to reduce and fold
* - detect: -- a.k.a. find, search
* - detect:ifNone:
* - anySatisfy: -- a.k.a. exists
* - allSatisfy:
*
* Since Maps have two data-points per entry (i.e. key and value), most of the implementations in this class
* iterates over the values only, unless otherwise specified.
* To iterate over the keys, use keySet() with standard {@link Iterate} methods.
*
* @see Iterate
* @since 1.0
*/
public final class MapIterate
{
private MapIterate()
{
throw new AssertionError("Suppress default constructor for noninstantiability");
}
/**
* A null-safe check on a map to see if it isEmpty. A null collection results in {@code true}.
*/
public static boolean isEmpty(Map, ?> map)
{
return map == null || map.isEmpty();
}
/**
* A null-safe check on a map to see if it notEmpty. A null collection results in {@code false}.
*/
public static boolean notEmpty(Map, ?> map)
{
return map != null && !map.isEmpty();
}
/**
* Get and return the value in the Map at the specified key, or if there is no value at the key, return the result
* of evaluating the specified {@link Function0}, and put that value in the map at the specified key.
*
* This method handles the {@code null}-value-at-key case correctly.
*/
public static V getIfAbsentPut(Map map, K key, Function0 extends V> instanceBlock)
{
if (map instanceof MutableMap)
{
return ((MutableMap) map).getIfAbsentPut(key, instanceBlock);
}
V result = map.get(key);
if (MapIterate.isAbsent(result, map, key))
{
result = instanceBlock.value();
map.put(key, result);
}
return result;
}
/**
* Get and return the value in the Map at the specified key, or if there is no value at the key, return the result
* of evaluating the specified {@link Function} with the {@code parameter}, and put that value in the map at
* the specified key.
*/
public static V getIfAbsentPutWith(
Map map,
K key,
Function super P, ? extends V> function,
P parameter)
{
V result = map.get(key);
if (MapIterate.isAbsent(result, map, key))
{
result = function.valueOf(parameter);
map.put(key, result);
}
return result;
}
/**
* Get and return the value in the Map that corresponds to the specified key, or if there is no value
* at the key, return the result of evaluating the specified {@link Function0}.
*/
public static V getIfAbsent(Map map, K key, Function0 extends V> instanceBlock)
{
if (map instanceof UnsortedMapIterable)
{
return ((MapIterable) map).getIfAbsent(key, instanceBlock);
}
V result = map.get(key);
if (MapIterate.isAbsent(result, map, key))
{
result = instanceBlock.value();
}
return result;
}
/**
* Get and return the value in the Map that corresponds to the specified key, or if there is no value
* at the key, return the result of evaluating the specified {@link Function} with the specified parameter.
*/
public static V getIfAbsentWith(
Map map,
K key,
Function super P, ? extends V> function,
P parameter)
{
if (map instanceof UnsortedMapIterable)
{
return ((MapIterable) map).getIfAbsentWith(key, function, parameter);
}
V result = map.get(key);
if (MapIterate.isAbsent(result, map, key))
{
result = function.valueOf(parameter);
}
return result;
}
/**
* Get and return the value in the Map at the specified key, or if there is no value at the key, return the
* {@code defaultValue}.
*/
public static V getIfAbsentDefault(Map map, K key, V defaultValue)
{
V result = map.get(key);
if (MapIterate.isAbsent(result, map, key))
{
result = defaultValue;
}
return result;
}
private static boolean isAbsent(V result, Map map, K key)
{
return result == null && !map.containsKey(key);
}
/**
* If there is a value in the Map tat the specified key, return the result of applying the specified Function
* on the value, otherwise return null.
*/
public static A ifPresentApply(
Map map,
K key,
Function super V, ? extends A> function)
{
if (map instanceof UnsortedMapIterable)
{
return ((MapIterable) map).ifPresentApply(key, function);
}
V result = map.get(key);
return MapIterate.isAbsent(result, map, key) ? null : function.valueOf(result);
}
/**
* @see Iterate#select(Iterable, Predicate)
*/
public static MutableList select(Map map, Predicate super V> predicate)
{
return MapIterate.select(map, predicate, FastList.newList());
}
/**
* @see Iterate#select(Iterable, Predicate, Collection)
*/
public static > R select(
Map map,
Predicate super V> predicate,
R targetCollection)
{
Procedure procedure = new SelectProcedure(predicate, targetCollection);
MapIterate.forEachValue(map, procedure);
return targetCollection;
}
/**
* @see Iterate#count(Iterable, Predicate)
*/
public static int count(Map map, Predicate super V> predicate)
{
CountProcedure procedure = new CountProcedure(predicate);
MapIterate.forEachValue(map, procedure);
return procedure.getCount();
}
/**
* For each entry of the source map, the Predicate2 is evaluated.
* If the result of the evaluation is true, the map entry is moved to a result map.
* The result map is returned containing all entries in the source map that evaluated to true.
*/
public static MutableMap selectMapOnEntry(
Map map,
Predicate2 super K, ? super V> predicate)
{
return MapIterate.selectMapOnEntry(map, predicate, UnifiedMap.newMap());
}
/**
* For each entry of the source map, the Predicate2 is evaluated.
* If the result of the evaluation is true, the map entry is moved to a result map.
* The result map is returned containing all entries in the source map that evaluated to true.
*/
public static > R selectMapOnEntry(
Map map,
final Predicate2 super K, ? super V> predicate,
R target)
{
final Procedure2 mapTransferProcedure = new MapPutProcedure(target);
Procedure2 procedure = new Procedure2()
{
public void value(K key, V value)
{
if (predicate.accept(key, value))
{
mapTransferProcedure.value(key, value);
}
}
};
MapIterate.forEachKeyValue(map, procedure);
return target;
}
/**
* For each key of the source map, the Predicate is evaluated.
* If the result of the evaluation is true, the map entry is moved to a result map.
* The result map is returned containing all entries in the source map that evaluated to true.
*/
public static MutableMap selectMapOnKey(Map map, final Predicate super K> predicate)
{
MutableMap resultMap = UnifiedMap.newMap();
final Procedure2 mapTransferProcedure = new MapPutProcedure(resultMap);
Procedure2 procedure = new Procedure2()
{
public void value(K key, V value)
{
if (predicate.accept(key))
{
mapTransferProcedure.value(key, value);
}
}
};
MapIterate.forEachKeyValue(map, procedure);
return resultMap;
}
/**
* For each value of the source map, the Predicate is evaluated.
* If the result of the evaluation is true, the map entry is moved to a result map.
* The result map is returned containing all entries in the source map that evaluated to true.
*/
public static MutableMap selectMapOnValue(Map map, final Predicate super V> predicate)
{
MutableMap resultMap = UnifiedMap.newMap();
final Procedure2 mapTransferProcedure = new MapPutProcedure(resultMap);
Procedure2 procedure = new Procedure2()
{
public void value(K key, V value)
{
if (predicate.accept(value))
{
mapTransferProcedure.value(key, value);
}
}
};
MapIterate.forEachKeyValue(map, procedure);
return resultMap;
}
/**
* @see Iterate#reject(Iterable, Predicate)
*/
public static MutableList reject(Map map, Predicate super V> predicate)
{
return MapIterate.reject(map, predicate, FastList.newList());
}
/**
* @see Iterate#reject(Iterable, Predicate, Collection)
*/
public static > R reject(
Map map,
Predicate super V> predicate,
R targetCollection)
{
Procedure procedure = new RejectProcedure(predicate, targetCollection);
MapIterate.forEachValue(map, procedure);
return targetCollection;
}
/**
* For each value of the map, predicate is evaluated with the element as the parameter.
* Each element which causes predicate to evaluate to false is included in the new collection.
*/
public static MutableMap rejectMapOnEntry(
Map map,
Predicate2 super K, ? super V> predicate)
{
return MapIterate.rejectMapOnEntry(map, predicate, UnifiedMap.newMap());
}
/**
* For each value of the map, predicate is evaluated with the element as the parameter.
* Each element which causes predicate to evaluate to false is added to the targetCollection.
*/
public static > R rejectMapOnEntry(
Map map,
final Predicate2 super K, ? super V> predicate,
final R target)
{
MapIterate.forEachKeyValue(map, new Procedure2()
{
public void value(K argument1, V argument2)
{
if (!predicate.accept(argument1, argument2))
{
target.put(argument1, argument2);
}
}
});
return target;
}
/**
* Adds all the keys from map to a the specified targetCollection.
*/
public static Collection addAllKeysTo(Map map, Collection targetCollection)
{
MapIterate.forEachKey(map, CollectionAddProcedure.on(targetCollection));
return targetCollection;
}
/**
* Adds all the values from map to a the specified targetCollection.
*/
public static Collection addAllValuesTo(Map map, Collection targetCollection)
{
MapIterate.forEachValue(map, CollectionAddProcedure.on(targetCollection));
return targetCollection;
}
/**
* @see Iterate#collect(Iterable, Function)
*/
public static MutableList collect(
Map map,
Function super V, ? extends A> function)
{
return collect(map, function, FastList.newList(map.size()));
}
/**
* @see RichIterable#collectBoolean(BooleanFunction)
*/
public static MutableBooleanCollection collectBoolean(
Map map,
BooleanFunction super V> booleanFunction)
{
return collectBoolean(map, booleanFunction, new BooleanArrayList(map.size()));
}
/**
* @see RichIterable#collectBoolean(BooleanFunction, MutableBooleanCollection)
*/
public static R collectBoolean(
Map map,
BooleanFunction super V> booleanFunction,
R target)
{
MapIterate.forEachValue(map, new CollectBooleanProcedure(booleanFunction, target));
return target;
}
/**
* @see RichIterable#collectByte(ByteFunction)
*/
public static MutableByteCollection collectByte(
Map map,
ByteFunction super V> byteFunction)
{
return collectByte(map, byteFunction, new ByteArrayList(map.size()));
}
/**
* @see RichIterable#collectByte(ByteFunction, MutableByteCollection)
*/
public static R collectByte(
Map map,
ByteFunction super V> byteFunction,
R target)
{
MapIterate.forEachValue(map, new CollectByteProcedure(byteFunction, target));
return target;
}
/**
* @see RichIterable#collectChar(CharFunction)
*/
public static MutableCharCollection collectChar(
Map map,
CharFunction super V> charFunction)
{
return collectChar(map, charFunction, new CharArrayList(map.size()));
}
/**
* @see RichIterable#collectChar(CharFunction, MutableCharCollection)
*/
public static R collectChar(
Map map,
CharFunction super V> charFunction,
R target)
{
MapIterate.forEachValue(map, new CollectCharProcedure(charFunction, target));
return target;
}
/**
* @see RichIterable#collectDouble(DoubleFunction)
*/
public static MutableDoubleCollection collectDouble(
Map map,
DoubleFunction super V> doubleFunction)
{
return collectDouble(map, doubleFunction, new DoubleArrayList(map.size()));
}
/**
* @see RichIterable#collectDouble(DoubleFunction, MutableDoubleCollection)
*/
public static R collectDouble(
Map map,
DoubleFunction super V> doubleFunction,
R target)
{
MapIterate.forEachValue(map, new CollectDoubleProcedure(doubleFunction, target));
return target;
}
/**
* @see RichIterable#collectFloat(FloatFunction)
*/
public static MutableFloatCollection collectFloat(
Map map,
FloatFunction super V> floatFunction)
{
return collectFloat(map, floatFunction, new FloatArrayList(map.size()));
}
/**
* @see RichIterable#collectFloat(FloatFunction, MutableFloatCollection)
*/
public static R collectFloat(
Map map,
FloatFunction super V> floatFunction,
R target)
{
MapIterate.forEachValue(map, new CollectFloatProcedure(floatFunction, target));
return target;
}
/**
* @see RichIterable#collectInt(IntFunction)
*/
public static MutableIntCollection collectInt(
Map map,
IntFunction super V> intFunction)
{
return collectInt(map, intFunction, new IntArrayList(map.size()));
}
/**
* @see RichIterable#collectInt(IntFunction, MutableIntCollection)
*/
public static R collectInt(
Map map,
IntFunction super V> intFunction,
R target)
{
MapIterate.forEachValue(map, new CollectIntProcedure(intFunction, target));
return target;
}
/**
* @see RichIterable#collectLong(LongFunction)
*/
public static MutableLongCollection collectLong(
Map map,
LongFunction super V> longFunction)
{
return collectLong(map, longFunction, new LongArrayList(map.size()));
}
/**
* @see RichIterable#collectLong(LongFunction, MutableLongCollection)
*/
public static R collectLong(
Map map,
LongFunction super V> longFunction,
R target)
{
MapIterate.forEachValue(map, new CollectLongProcedure(longFunction, target));
return target;
}
/**
* @see RichIterable#collectShort(ShortFunction)
*/
public static MutableShortCollection collectShort(
Map map,
ShortFunction super V> shortFunction)
{
return collectShort(map, shortFunction, new ShortArrayList(map.size()));
}
/**
* @see RichIterable#collectShort(ShortFunction, MutableShortCollection)
*/
public static R collectShort(
Map map,
ShortFunction super V> shortFunction,
R target)
{
MapIterate.forEachValue(map, new CollectShortProcedure(shortFunction, target));
return target;
}
/**
* For each value of the map, the function is evaluated with the key and value as the parameter.
* The results of these evaluations are collected into a new UnifiedMap.
*/
public static MutableMap collect(
Map map,
Function2 super K, ? super V, Pair> function)
{
return MapIterate.collect(map, function, UnifiedMap.newMap(map.size()));
}
/**
* For each value of the map, the function is evaluated with the key and value as the parameter.
* The results of these evaluations are collected into the target map.
*/
public static > R collect(
Map map,
final Function2 super K1, ? super V1, Pair> function,
final R target)
{
MapIterate.forEachKeyValue(map, new Procedure2()
{
public void value(K1 key, V1 value)
{
Pair pair = function.value(key, value);
target.put(pair.getOne(), pair.getTwo());
}
});
return target;
}
/**
* For each key and value of the map, the function is evaluated with the key and value as the parameter.
* The results of these evaluations are collected into the target map.
*/
public static MutableMap collectValues(
Map map,
Function2 super K, ? super V, ? extends V2> function)
{
return MapIterate.collectValues(map, function, UnifiedMap.newMap(map.size()));
}
/**
* For each key and value of the map, the function is evaluated with the key and value as the parameter.
* The results of these evaluations are collected into the target map.
*/
public static > R collectValues(
Map map,
final Function2 super K, ? super V, ? extends V2> function,
final R target)
{
MapIterate.forEachKeyValue(map, new Procedure2()
{
public void value(K key, V value)
{
target.put(key, function.value(key, value));
}
});
return target;
}
/**
* For each value of the map, the Predicate2 is evaluated with the key and value as the parameter,
* and if true, then {@code function} is applied.
* The results of these evaluations are collected into a new map.
*/
public static MutableMap collectIf(
Map map,
Function2 super K1, ? super V1, Pair> function,
Predicate2 super K1, ? super V1> predicate)
{
return MapIterate.collectIf(map, function, predicate, UnifiedMap.newMap());
}
/**
* For each value of the map, the Predicate2 is evaluated with the key and value as the parameter,
* and if true, then {@code function} is applied.
* The results of these evaluations are collected into the target map.
*/
public static MutableMap collectIf(
Map map,
final Function2 super K1, ? super V1, Pair> function,
final Predicate2 super K1, ? super V1> predicate,
Map target)
{
final MutableMap result = MapAdapter.adapt(target);
MapIterate.forEachKeyValue(map, new Procedure2()
{
public void value(K1 key, V1 value)
{
if (predicate.accept(key, value))
{
Pair pair = function.value(key, value);
result.put(pair.getOne(), pair.getTwo());
}
}
});
return result;
}
/**
* For each key-value entry of a map, applies a function to each, and adds the transformed entry to a new Map.
*/
public static MutableMap collect(
Map map,
Function super K1, ? extends K2> keyFunction,
Function super V1, ? extends V2> valueFunction)
{
return MapIterate.collect(map, keyFunction, valueFunction, UnifiedMap.newMap());
}
/**
* For each key-value entry of a map, applies a function to each, and adds the transformed entry to the target Map.
*/
public static MutableMap collect(
Map map,
final Function super K1, ? extends K2> keyFunction,
final Function super V1, ? extends V2> valueFunction,
Map target)
{
return MapIterate.collect(map, new Function2>()
{
public Pair value(K1 key, V1 value)
{
return Tuples.pair(keyFunction.valueOf(key), valueFunction.valueOf(value));
}
}, MapAdapter.adapt(target));
}
/**
* @see Iterate#collect(Iterable, Function, Collection)
*/
public static > R collect(
Map map,
Function super V, ? extends A> function,
R targetCollection)
{
Procedure procedure = new CollectProcedure(function, targetCollection);
MapIterate.forEachValue(map, procedure);
return targetCollection;
}
/**
* For each value of the map, {@code procedure} is evaluated with the value as the parameter.
*/
public static void forEachValue(Map map, Procedure super V> procedure)
{
if (map == null)
{
throw new IllegalArgumentException("Cannot perform a forEachValue on null");
}
if (MapIterate.notEmpty(map))
{
if (map instanceof UnsortedMapIterable)
{
((MapIterable) map).forEachValue(procedure);
}
else
{
IterableIterate.forEach(map.values(), procedure);
}
}
}
/**
* For each key of the map, {@code procedure} is evaluated with the key as the parameter.
*/
public static void forEachKey(Map map, Procedure super K> procedure)
{
if (map == null)
{
throw new IllegalArgumentException("Cannot perform a forEachKey on null");
}
if (MapIterate.notEmpty(map))
{
if (map instanceof UnsortedMapIterable)
{
((MapIterable) map).forEachKey(procedure);
}
else
{
IterableIterate.forEach(map.keySet(), procedure);
}
}
}
/**
* For each entry of the map, {@code procedure} is evaluated with the element as the parameter.
*/
public static void forEachKeyValue(Map map, Procedure2 super K, ? super V> procedure)
{
if (map == null)
{
throw new IllegalArgumentException("Cannot perform a forEachKeyValue on null");
}
if (MapIterate.notEmpty(map))
{
if (map instanceof UnsortedMapIterable)
{
((MapIterable) map).forEachKeyValue(procedure);
}
else
{
IterableIterate.forEach(map.entrySet(), new MapEntryToProcedure2(procedure));
}
}
}
/**
* @see MapIterable#flipUniqueValues()
*/
public static MutableMap flipUniqueValues(MapIterable mapIterable)
{
final MutableMap result = UnifiedMap.newMap();
mapIterable.forEachKeyValue(new Procedure2()
{
public void value(K key, V value)
{
K oldKey = result.put(value, key);
if (oldKey != null)
{
throw new IllegalStateException("Duplicate value: " + value + " found at key: " + oldKey + " and key: " + key);
}
}
});
return result;
}
public static Pair detect(
Map map,
final Predicate2 super K, ? super V> predicate)
{
if (map == null)
{
throw new IllegalArgumentException("Cannot perform a detect on null");
}
if (map instanceof ImmutableMap || map instanceof MutableMap)
{
RichIterable> entries;
if (map instanceof ImmutableMap)
{
entries = ((ImmutableMap) map).keyValuesView();
}
else
{
entries = LazyIterate.adapt(map.entrySet()).collect(AbstractImmutableEntry.getPairFunction());
}
return entries.detect(new Predicate>()
{
public boolean accept(Pair each)
{
return predicate.accept(each.getOne(), each.getTwo());
}
});
}
for (Map.Entry entry : map.entrySet())
{
if (predicate.accept(entry.getKey(), entry.getValue()))
{
return Tuples.pairFrom(entry);
}
}
return null;
}
/**
* @see Iterate#detect(Iterable, Predicate)
*/
public static V detect(Map map, Predicate super V> predicate)
{
return IterableIterate.detect(map.values(), predicate);
}
/**
* @see Iterate#detectIfNone(Iterable, Predicate, Object)
*/
public static V detectIfNone(Map map, Predicate super V> predicate, V ifNone)
{
return Iterate.detectIfNone(map.values(), predicate, ifNone);
}
/**
* @see Iterate#injectInto(Object, Iterable, Function2)
*/
public static IV injectInto(
IV injectValue,
Map map,
Function2 super IV, ? super V, ? extends IV> function)
{
return Iterate.injectInto(injectValue, map.values(), function);
}
/**
* Same as {@link #injectInto(Object, Map, Function2)}, but only applies the value to the function
* if the predicate returns true for the value.
*
* @see #injectInto(Object, Map, Function2)
*/
public static IV injectIntoIf(
IV initialValue,
Map map,
final Predicate super V> predicate,
final Function2 super IV, ? super V, ? extends IV> function)
{
Function2 ifFunction = new Function2()
{
public IV value(IV accumulator, V item)
{
if (predicate.accept(item))
{
return function.value(accumulator, item);
}
return accumulator;
}
};
return Iterate.injectInto(initialValue, map.values(), ifFunction);
}
/**
* @see Iterate#anySatisfy(Iterable, Predicate)
*/
public static boolean anySatisfy(Map map, Predicate super V> predicate)
{
return IterableIterate.anySatisfy(map.values(), predicate);
}
/**
* @see Iterate#allSatisfy(Iterable, Predicate)
*/
public static boolean allSatisfy(Map map, Predicate super V> predicate)
{
return IterableIterate.allSatisfy(map.values(), predicate);
}
/**
* @see Iterate#noneSatisfy(Iterable, Predicate)
*/
public static boolean noneSatisfy(Map map, Predicate super V> predicate)
{
return IterableIterate.noneSatisfy(map.values(), predicate);
}
/**
* Iterate over the specified map applying the specified Function to each value
* and return the results as a List.
*/
public static MutableList> toListOfPairs(Map map)
{
final MutableList> pairs = FastList.newList(map.size());
MapIterate.forEachKeyValue(map, new Procedure2()
{
public void value(K key, V value)
{
pairs.add(Tuples.pair(key, value));
}
});
return pairs;
}
/**
* Iterate over the specified map applying the specified Function to each value
* and return the results as a sorted List using the specified Comparator.
*/
public static MutableList toSortedList(
Map map,
Comparator super V> comparator)
{
return Iterate.toSortedList(map.values(), comparator);
}
/**
* Return a new map swapping key-value for value-key.
* If the original map contains entries with the same value, the result mapping is undefined,
* in that the last entry applied wins (the order of application is undefined).
*/
public static MutableMap reverseMapping(Map map)
{
final MutableMap reverseMap = UnifiedMap.newMap(map.size());
MapIterate.forEachKeyValue(map, new Procedure2()
{
public void value(K sourceKey, V sourceValue)
{
reverseMap.put(sourceValue, sourceKey);
}
});
return reverseMap;
}
/**
* Return the number of occurrences of object in the specified map.
*/
public static int occurrencesOf(Map map, V object)
{
return Iterate.count(map.values(), Predicates.equal(object));
}
/**
* Return the number of occurrences where object is equal to the specified attribute in the specified map.
*/
public static int occurrencesOfAttribute(
Map map,
Function super V, ? extends A> function,
A object)
{
return Iterate.count(map.values(), Predicates.attributeEqual(function, object));
}
public static MutableSetMultimap flip(MapIterable iMap)
{
final MutableSetMultimap result = Multimaps.mutable.set.with();
iMap.forEachKeyValue(new Procedure2()
{
public void value(K key, V val)
{
result.put(val, key);
}
});
return result;
}
public static MutableSortedSetMultimap flip(SortedMapIterable iMap)
{
final MutableSortedSetMultimap result = new TreeSortedSetMultimap(iMap.comparator());
iMap.forEachKeyValue(new Procedure2()
{
public void value(K key, V val)
{
result.put(val, key);
}
});
return result;
}
}