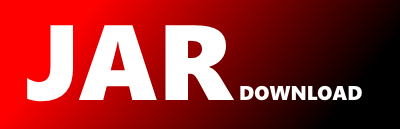
org.eclipse.collections.impl.map.AbstractSynchronizedMapIterable Maven / Gradle / Ivy
/*
* Copyright (c) 2015 Goldman Sachs.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.map;
import java.util.Map;
import java.util.Set;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function0;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.block.procedure.Procedure;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.map.MutableMapIterable;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.impl.collection.AbstractSynchronizedRichIterable;
import org.eclipse.collections.impl.tuple.AbstractImmutableEntry;
import org.eclipse.collections.impl.utility.Iterate;
import org.eclipse.collections.impl.utility.LazyIterate;
/**
* A synchronized view of a map.
*/
public abstract class AbstractSynchronizedMapIterable
extends AbstractSynchronizedRichIterable
implements MutableMapIterable
{
protected AbstractSynchronizedMapIterable(MutableMapIterable delegate)
{
super(delegate, null);
}
protected AbstractSynchronizedMapIterable(MutableMapIterable delegate, Object lock)
{
super(delegate, lock);
}
@Override
protected MutableMapIterable getDelegate()
{
return (MutableMapIterable) super.getDelegate();
}
public V get(Object key)
{
synchronized (this.lock)
{
return this.getDelegate().get(key);
}
}
public V getIfAbsent(K key, Function0 extends V> function)
{
synchronized (this.lock)
{
return this.getDelegate().getIfAbsent(key, function);
}
}
public V getIfAbsentValue(K key, V value)
{
synchronized (this.lock)
{
return this.getDelegate().getIfAbsentValue(key, value);
}
}
public
© 2015 - 2025 Weber Informatics LLC | Privacy Policy