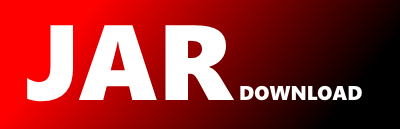
org.eclipse.collections.impl.factory.Sets Maven / Gradle / Ivy
/*
* Copyright (c) 2016 Goldman Sachs.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.factory;
import java.util.Arrays;
import java.util.Collection;
import java.util.Comparator;
import java.util.Set;
import java.util.SortedSet;
import java.util.TreeSet;
import org.eclipse.collections.api.LazyIterable;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.factory.set.FixedSizeSetFactory;
import org.eclipse.collections.api.factory.set.ImmutableSetFactory;
import org.eclipse.collections.api.factory.set.MutableSetFactory;
import org.eclipse.collections.api.set.MutableSet;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.impl.block.factory.Comparators;
import org.eclipse.collections.impl.set.fixed.FixedSizeSetFactoryImpl;
import org.eclipse.collections.impl.set.immutable.ImmutableSetFactoryImpl;
import org.eclipse.collections.impl.set.mutable.MutableSetFactoryImpl;
import org.eclipse.collections.impl.set.mutable.SetAdapter;
import org.eclipse.collections.impl.set.mutable.UnifiedSet;
import org.eclipse.collections.impl.tuple.Tuples;
import org.eclipse.collections.impl.utility.ArrayIterate;
import org.eclipse.collections.impl.utility.Iterate;
import org.eclipse.collections.impl.utility.LazyIterate;
/**
* Set algebra operations are available in this class as static utility.
*
* Most operations are non-destructive, i.e. no input sets are modified during execution.
* The exception is operations ending in "Into." These accept the target collection of
* the final calculation as the first parameter.
*
* Some effort is made to return a {@code SortedSet} if any input set is sorted, but
* this is not guaranteed (e.g., this will not be the case for collections proxied by
* Hibernate). When in doubt, specify the target collection explicitly with the "Into"
* version.
*
* This class should be used to create instances of MutableSet, ImmutableSet and FixedSizeSet
*
* Mutable Examples:
*
*
* MutableSet<String> emptySet = Sets.mutable.empty();
* MutableSet<String> setWith = Sets.mutable.with("a", "b", "c");
* MutableSet<String> setOf = Sets.mutable.of("a", "b", "c");
*
*
* Immutable Examples:
*
*
* ImmutableSet<String> emptySet = Sets.immutable.empty();
* ImmutableSet<String> setWith = Sets.immutable.with("a", "b", "c");
* ImmutableSet<String> setOf = Sets.immutable.of("a", "b", "c");
*
*
* FixedSize Examples:
*
*
* FixedSizeList<String> emptySet = Sets.fixedSize.empty();
* FixedSizeList<String> setWith = Sets.fixedSize.with("a", "b", "c");
* FixedSizeList<String> setOf = Sets.fixedSize.of("a", "b", "c");
*
*/
@SuppressWarnings("ConstantNamingConvention")
public final class Sets
{
public static final ImmutableSetFactory immutable = new ImmutableSetFactoryImpl();
public static final FixedSizeSetFactory fixedSize = new FixedSizeSetFactoryImpl();
public static final MutableSetFactory mutable = new MutableSetFactoryImpl();
private static final Predicate> INSTANCE_OF_SORTED_SET_PREDICATE = set -> set instanceof SortedSet;
private static final Predicate> HAS_NON_NULL_COMPARATOR = set -> ((SortedSet>) set).comparator() != null;
private Sets()
{
throw new AssertionError("Suppress default constructor for noninstantiability");
}
public static MutableSet union(
Set extends E> setA,
Set extends E> setB)
{
return unionInto(newSet(setA, setB), setA, setB);
}
public static > R unionInto(
R targetSet,
Set extends E> setA,
Set extends E> setB)
{
return setA.size() > setB.size() ? fillSet(targetSet, Sets.addAllProcedure(), setA, setB)
: fillSet(targetSet, Sets.addAllProcedure(), setB, setA);
}
public static MutableSet unionAll(Set extends E>... sets)
{
return unionAllInto(newSet(sets), sets);
}
public static > R unionAllInto(
R targetSet,
Set extends E>... sets)
{
Arrays.sort(sets, 0, sets.length, Comparators.descendingCollectionSizeComparator());
return fillSet(targetSet, Sets.addAllProcedure(), sets);
}
public static MutableSet intersect(
Set extends E> setA,
Set extends E> setB)
{
return intersectInto(newSet(setA, setB), setA, setB);
}
public static > R intersectInto(
R targetSet,
Set extends E> setA,
Set extends E> setB)
{
return setA.size() < setB.size() ? fillSet(targetSet, Sets.retainAllProcedure(), setA, setB)
: fillSet(targetSet, Sets.retainAllProcedure(), setB, setA);
}
public static MutableSet intersectAll(Set extends E>... sets)
{
return intersectAllInto(newSet(sets), sets);
}
public static > R intersectAllInto(
R targetSet,
Set extends E>... sets)
{
Arrays.sort(sets, 0, sets.length, Comparators.ascendingCollectionSizeComparator());
return fillSet(targetSet, Sets.retainAllProcedure(), sets);
}
public static MutableSet difference(
Set extends E> minuendSet,
Set extends E> subtrahendSet)
{
return differenceInto(newSet(minuendSet, subtrahendSet), minuendSet, subtrahendSet);
}
public static > R differenceInto(
R targetSet,
Set extends E> minuendSet,
Set extends E> subtrahendSet)
{
return fillSet(targetSet, Sets.removeAllProcedure(), minuendSet, subtrahendSet);
}
public static MutableSet differenceAll(Set extends E>... sets)
{
return differenceAllInto(newSet(sets), sets);
}
public static > R differenceAllInto(
R targetSet,
Set extends E>... sets)
{
return fillSet(targetSet, Sets.removeAllProcedure(), sets);
}
public static MutableSet symmetricDifference(
Set extends E> setA,
Set extends E> setB)
{
return symmetricDifferenceInto(newSet(setA, setB), setA, setB);
}
public static > R symmetricDifferenceInto(
R targetSet,
Set extends E> setA,
Set extends E> setB)
{
return unionInto(
targetSet,
differenceInto(newSet(setA, setB), setA, setB),
differenceInto(newSet(setA, setB), setB, setA));
}
public static boolean isSubsetOf(
Set extends E> candidateSubset,
Set extends E> candidateSuperset)
{
return candidateSubset.size() <= candidateSuperset.size()
&& candidateSuperset.containsAll(candidateSubset);
}
public static boolean isProperSubsetOf(
Set extends E> candidateSubset,
Set extends E> candidateSuperset)
{
return candidateSubset.size() < candidateSuperset.size()
&& candidateSuperset.containsAll(candidateSubset);
}
private static MutableSet newSet(Set extends E>... sets)
{
Comparator super E> comparator = extractComparator(sets);
if (comparator != null)
{
// TODO: this should return a SortedSetAdapter once implemented
return SetAdapter.adapt(new TreeSet<>(comparator));
}
return UnifiedSet.newSet();
}
private static Comparator super E> extractComparator(Set extends E>... sets)
{
Collection> sortedSetCollection = ArrayIterate.select(sets, INSTANCE_OF_SORTED_SET_PREDICATE);
if (sortedSetCollection.isEmpty())
{
return null;
}
SortedSet sortedSetWithComparator = (SortedSet) Iterate.detect(sortedSetCollection, HAS_NON_NULL_COMPARATOR);
if (sortedSetWithComparator != null)
{
return sortedSetWithComparator.comparator();
}
return Comparators.safeNullsLow(Comparators.naturalOrder());
}
private static > R fillSet(
R targetSet,
Procedure2, R> procedure,
Set extends E>... sets)
{
targetSet.addAll(sets[0]);
for (int i = 1; i < sets.length; i++)
{
procedure.value(sets[i], targetSet);
}
return targetSet;
}
private static > Procedure2, R> addAllProcedure()
{
return (argumentSet, targetSet) -> targetSet.addAll(argumentSet);
}
private static > Procedure2, R> retainAllProcedure()
{
return (argumentSet, targetSet) -> targetSet.retainAll(argumentSet);
}
private static > Procedure2, R> removeAllProcedure()
{
return (argumentSet, targetSet) -> targetSet.removeAll(argumentSet);
}
public static MutableSet> powerSet(Set set)
{
MutableSet> seed = UnifiedSet.newSetWith(UnifiedSet.newSet());
return Iterate.injectInto(seed, set, (accumulator, element) -> Sets.union(accumulator, accumulator.collect(innerSet -> innerSet.toSet().with(element))));
}
public static LazyIterable> cartesianProduct(Set set1, Set set2)
{
return LazyIterate.flatCollect(set1, first -> LazyIterate.collect(set2, second -> Tuples.pair(first, second)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy