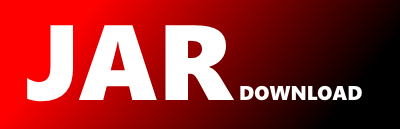
org.eclipse.collections.impl.lazy.primitive.CollectIntIterable Maven / Gradle / Ivy
/*
* Copyright (c) 2017 Goldman Sachs.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.lazy.primitive;
import java.util.Arrays;
import java.util.Iterator;
import org.eclipse.collections.api.IntIterable;
import org.eclipse.collections.api.LazyIterable;
import org.eclipse.collections.api.bag.primitive.MutableIntBag;
import org.eclipse.collections.api.block.function.primitive.IntFunction;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.predicate.primitive.IntPredicate;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.block.procedure.primitive.IntProcedure;
import org.eclipse.collections.api.block.procedure.primitive.ObjectIntProcedure;
import org.eclipse.collections.api.iterator.IntIterator;
import org.eclipse.collections.api.list.primitive.MutableIntList;
import org.eclipse.collections.api.set.primitive.MutableIntSet;
import org.eclipse.collections.impl.bag.mutable.primitive.IntHashBag;
import org.eclipse.collections.impl.list.mutable.primitive.IntArrayList;
import org.eclipse.collections.impl.set.mutable.primitive.IntHashSet;
import net.jcip.annotations.Immutable;
/**
* This file was automatically generated from template file collectPrimitiveIterable.stg.
*/
@Immutable
public class CollectIntIterable
extends AbstractLazyIntIterable
{
private final LazyIterable iterable;
private final IntFunction super T> function;
private final IntFunctionToProcedure intFunctionToProcedure;
public CollectIntIterable(LazyIterable adapted, IntFunction super T> function)
{
this.iterable = adapted;
this.function = function;
this.intFunctionToProcedure = new IntFunctionToProcedure(function);
}
public IntIterator intIterator()
{
return new IntIterator()
{
private final Iterator iterator = CollectIntIterable.this.iterable.iterator();
public int next()
{
return CollectIntIterable.this.function.intValueOf(this.iterator.next());
}
public boolean hasNext()
{
return this.iterator.hasNext();
}
};
}
public void forEach(IntProcedure procedure)
{
this.each(procedure);
}
/**
* @since 7.0.
*/
public void each(IntProcedure procedure)
{
this.iterable.forEachWith(this.intFunctionToProcedure, procedure);
}
@Override
public int size()
{
return this.iterable.size();
}
@Override
public boolean isEmpty()
{
return this.iterable.isEmpty();
}
@Override
public boolean notEmpty()
{
return this.iterable.notEmpty();
}
@Override
public int count(final IntPredicate predicate)
{
return this.iterable.count((T each) -> predicate.accept(this.function.intValueOf(each)));
}
@Override
public boolean anySatisfy(final IntPredicate predicate)
{
return this.iterable.anySatisfy((T each) -> predicate.accept(this.function.intValueOf(each)));
}
@Override
public boolean allSatisfy(final IntPredicate predicate)
{
return this.iterable.allSatisfy((T each) -> predicate.accept(this.function.intValueOf(each)));
}
@Override
public boolean noneSatisfy(final IntPredicate predicate)
{
return this.iterable.allSatisfy((T each) -> !predicate.accept(this.function.intValueOf(each)));
}
@Override
public int[] toArray()
{
final int[] array = new int[this.size()];
this.iterable.forEachWithIndex((T each, int index) -> array[index] = this.function.intValueOf(each));
return array;
}
@Override
public int[] toSortedArray()
{
int[] array = this.toArray();
Arrays.sort(array);
return array;
}
@Override
public MutableIntList toList()
{
return IntArrayList.newList(this);
}
@Override
public MutableIntSet toSet()
{
return IntHashSet.newSet(this);
}
@Override
public MutableIntBag toBag()
{
return IntHashBag.newBag(this);
}
@Override
public boolean containsAll(int... source)
{
for (int value : source)
{
if (!this.contains(value))
{
return false;
}
}
return true;
}
@Override
public boolean containsAll(IntIterable source)
{
for (IntIterator iterator = source.intIterator(); iterator.hasNext(); )
{
if (!this.contains(iterator.next()))
{
return false;
}
}
return true;
}
private static final class IntFunctionToProcedure implements Procedure2
{
private static final long serialVersionUID = 1L;
private final IntFunction super T> function;
private IntFunctionToProcedure(IntFunction super T> function)
{
this.function = function;
}
public void value(T each, IntProcedure procedure)
{
procedure.value(this.function.intValueOf(each));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy