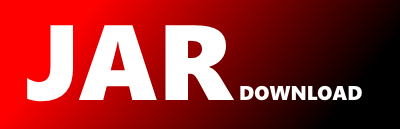
org.eclipse.collections.impl.map.mutable.AbstractMutableMapIterable Maven / Gradle / Ivy
/*
* Copyright (c) 2017 Goldman Sachs and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.map.mutable;
import java.util.Iterator;
import java.util.Optional;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.bag.MutableBag;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function0;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.function.primitive.DoubleFunction;
import org.eclipse.collections.api.block.function.primitive.FloatFunction;
import org.eclipse.collections.api.block.function.primitive.IntFunction;
import org.eclipse.collections.api.block.function.primitive.LongFunction;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.map.MutableMap;
import org.eclipse.collections.api.map.MutableMapIterable;
import org.eclipse.collections.api.map.primitive.MutableObjectDoubleMap;
import org.eclipse.collections.api.map.primitive.MutableObjectLongMap;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.impl.block.factory.PrimitiveFunctions;
import org.eclipse.collections.impl.block.procedure.MutatingAggregationProcedure;
import org.eclipse.collections.impl.block.procedure.NonMutatingAggregationProcedure;
import org.eclipse.collections.impl.factory.Bags;
import org.eclipse.collections.impl.map.AbstractMapIterable;
import org.eclipse.collections.impl.map.mutable.primitive.ObjectDoubleHashMap;
import org.eclipse.collections.impl.map.mutable.primitive.ObjectLongHashMap;
import org.eclipse.collections.impl.tuple.AbstractImmutableEntry;
import org.eclipse.collections.impl.utility.LazyIterate;
import org.eclipse.collections.impl.utility.MapIterate;
public abstract class AbstractMutableMapIterable extends AbstractMapIterable implements MutableMapIterable
{
@Override
public Iterator iterator()
{
return this.values().iterator();
}
@Override
public V getIfAbsentPut(K key, Function0 extends V> function)
{
V result = this.get(key);
if (this.isAbsent(result, key))
{
result = function.value();
this.put(key, result);
}
return result;
}
@Override
public V getIfAbsentPut(K key, V value)
{
V result = this.get(key);
if (this.isAbsent(result, key))
{
result = value;
this.put(key, result);
}
return result;
}
@Override
public V getIfAbsentPutWithKey(K key, Function super K, ? extends V> function)
{
return this.getIfAbsentPutWith(key, function, key);
}
@Override
public V getIfAbsentPutWith(K key, Function super P, ? extends V> function, P parameter)
{
V result = this.get(key);
if (this.isAbsent(result, key))
{
result = function.valueOf(parameter);
this.put(key, result);
}
return result;
}
@Override
public V updateValue(K key, Function0 extends V> factory, Function super V, ? extends V> function)
{
V oldValue = this.getIfAbsent(key, factory);
V newValue = function.valueOf(oldValue);
this.put(key, newValue);
return newValue;
}
@Override
public
V updateValueWith(K key, Function0 extends V> factory, Function2 super V, ? super P, ? extends V> function, P parameter)
{
V oldValue = this.getIfAbsent(key, factory);
V newValue = function.value(oldValue, parameter);
this.put(key, newValue);
return newValue;
}
@Override
public MutableMapIterable groupByUniqueKey(Function super V, ? extends VV> function)
{
return this.groupByUniqueKey(function, UnifiedMap.newMap());
}
@Override
public MutableMap aggregateInPlaceBy(
Function super V, ? extends K2> groupBy,
Function0 extends V2> zeroValueFactory,
Procedure2 super V2, ? super V> mutatingAggregator)
{
MutableMap map = UnifiedMap.newMap();
this.forEach(new MutatingAggregationProcedure<>(map, groupBy, zeroValueFactory, mutatingAggregator));
return map;
}
@Override
public MutableMap aggregateBy(
Function super V, ? extends K2> groupBy,
Function0 extends V2> zeroValueFactory,
Function2 super V2, ? super V, ? extends V2> nonMutatingAggregator)
{
MutableMap map = UnifiedMap.newMap();
this.forEach(new NonMutatingAggregationProcedure<>(map, groupBy, zeroValueFactory, nonMutatingAggregator));
return map;
}
@Override
public RichIterable keysView()
{
return LazyIterate.adapt(this.keySet());
}
@Override
public RichIterable valuesView()
{
return LazyIterate.adapt(this.values());
}
@Override
public RichIterable> keyValuesView()
{
return LazyIterate.adapt(this.entrySet()).collect(AbstractImmutableEntry.getPairFunction());
}
@Override
public MutableMap collect(Function2 super K, ? super V, Pair> function)
{
return MapIterate.collect(this, function, UnifiedMap.newMap(this.size()));
}
@Override
public MutableMap flipUniqueValues()
{
return MapIterate.flipUniqueValues(this);
}
@Override
public Pair detect(Predicate2 super K, ? super V> predicate)
{
return MapIterate.detect(this, predicate);
}
@Override
public Optional> detectOptional(Predicate2 super K, ? super V> predicate)
{
return MapIterate.detectOptional(this, predicate);
}
@Override
public MutableObjectLongMap sumByInt(Function super V, ? extends V1> groupBy, IntFunction super V> function)
{
MutableObjectLongMap result = ObjectLongHashMap.newMap();
return this.injectInto(result, PrimitiveFunctions.sumByIntFunction(groupBy, function));
}
@Override
public MutableObjectDoubleMap sumByFloat(Function super V, ? extends V1> groupBy, FloatFunction super V> function)
{
MutableObjectDoubleMap result = ObjectDoubleHashMap.newMap();
return this.injectInto(result, PrimitiveFunctions.sumByFloatFunction(groupBy, function));
}
@Override
public MutableObjectLongMap sumByLong(Function super V, ? extends V1> groupBy, LongFunction super V> function)
{
MutableObjectLongMap result = ObjectLongHashMap.newMap();
return this.injectInto(result, PrimitiveFunctions.sumByLongFunction(groupBy, function));
}
@Override
public MutableObjectDoubleMap sumByDouble(Function super V, ? extends V1> groupBy, DoubleFunction super V> function)
{
MutableObjectDoubleMap result = ObjectDoubleHashMap.newMap();
return this.injectInto(result, PrimitiveFunctions.sumByDoubleFunction(groupBy, function));
}
/**
* @since 9.0
*/
@Override
public MutableBag countBy(Function super V, ? extends V1> function)
{
return this.collect(function, Bags.mutable.empty());
}
/**
* @since 9.0
*/
@Override
public MutableBag countByWith(Function2 super V, ? super P, ? extends V1> function, P parameter)
{
return this.collectWith(function, parameter, Bags.mutable.empty());
}
}