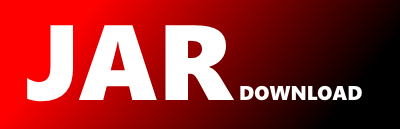
org.eclipse.collections.impl.multimap.set.SynchronizedSetMultimap Maven / Gradle / Ivy
/*
* Copyright (c) 2016 Shotaro Sano.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.multimap.set;
import java.io.Serializable;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.multimap.bag.MutableBagMultimap;
import org.eclipse.collections.api.multimap.set.ImmutableSetMultimap;
import org.eclipse.collections.api.multimap.set.MutableSetMultimap;
import org.eclipse.collections.api.set.MutableSet;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.impl.multimap.AbstractSynchronizedMultimap;
public class SynchronizedSetMultimap
extends AbstractSynchronizedMultimap
implements MutableSetMultimap, Serializable
{
private static final long serialVersionUID = 1L;
public SynchronizedSetMultimap(MutableSetMultimap multimap)
{
super(multimap);
}
public SynchronizedSetMultimap(MutableSetMultimap multimap, Object newLock)
{
super(multimap, newLock);
}
/**
* This method will take a Multimap and wrap it directly in a SynchronizedSetMultimap.
*/
public static SynchronizedSetMultimap of(MutableSetMultimap multimap)
{
if (multimap == null)
{
throw new IllegalArgumentException("cannot create a SynchronizedSetMultimap for null");
}
return new SynchronizedSetMultimap<>(multimap);
}
/**
* This method will take a Multimap and wrap it directly in a SynchronizedSetMultimap.
* Additionally, a developer specifies which lock to use with the collection.
*/
public static SynchronizedSetMultimap of(MutableSetMultimap multimap, Object lock)
{
if (multimap == null)
{
throw new IllegalArgumentException("cannot create a SynchronizedSetMultimap for null");
}
return new SynchronizedSetMultimap<>(multimap, lock);
}
@Override
public MutableSet replaceValues(K key, Iterable extends V> values)
{
synchronized (this.getLock())
{
return this.getDelegate().replaceValues(key, values);
}
}
@Override
public MutableSet removeAll(Object key)
{
synchronized (this.getLock())
{
return this.getDelegate().removeAll(key);
}
}
@Override
public MutableSetMultimap newEmpty()
{
synchronized (this.getLock())
{
return this.getDelegate().newEmpty().asSynchronized();
}
}
@Override
public MutableSet get(K key)
{
synchronized (this.getLock())
{
return this.getDelegate().get(key);
}
}
@Override
public MutableSetMultimap toMutable()
{
synchronized (this.getLock())
{
return this.getDelegate().toMutable();
}
}
@Override
public ImmutableSetMultimap toImmutable()
{
synchronized (this.getLock())
{
return this.getDelegate().toImmutable();
}
}
@Override
public MutableSetMultimap flip()
{
synchronized (this.getLock())
{
return this.getDelegate().flip();
}
}
@Override
public MutableSetMultimap selectKeysValues(Predicate2 super K, ? super V> predicate)
{
synchronized (this.getLock())
{
return this.getDelegate().selectKeysValues(predicate);
}
}
@Override
public MutableSetMultimap rejectKeysValues(Predicate2 super K, ? super V> predicate)
{
synchronized (this.getLock())
{
return this.getDelegate().rejectKeysValues(predicate);
}
}
@Override
public MutableSetMultimap selectKeysMultiValues(Predicate2 super K, ? super Iterable> predicate)
{
synchronized (this.getLock())
{
return this.getDelegate().selectKeysMultiValues(predicate);
}
}
@Override
public MutableSetMultimap rejectKeysMultiValues(Predicate2 super K, ? super Iterable> predicate)
{
synchronized (this.getLock())
{
return this.getDelegate().rejectKeysMultiValues(predicate);
}
}
@Override
public MutableBagMultimap collectKeysValues(Function2 super K, ? super V, Pair> function)
{
synchronized (this.getLock())
{
return this.getDelegate().collectKeysValues(function);
}
}
@Override
public MutableBagMultimap collectValues(Function super V, ? extends V2> function)
{
synchronized (this.getLock())
{
return this.getDelegate().collectValues(function);
}
}
@Override
public MutableSetMultimap asSynchronized()
{
return this;
}
@Override
protected MutableSetMultimap getDelegate()
{
return (MutableSetMultimap) super.getDelegate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy