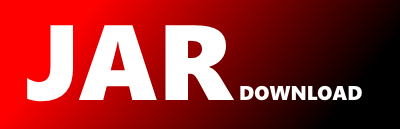
org.eclipse.collections.impl.set.immutable.ImmutableEmptySet Maven / Gradle / Ivy
/*
* Copyright (c) 2017 Goldman Sachs and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompany this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*/
package org.eclipse.collections.impl.set.immutable;
import java.io.IOException;
import java.io.Serializable;
import java.util.Collection;
import java.util.Comparator;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.Optional;
import java.util.Set;
import org.eclipse.collections.api.bag.ImmutableBag;
import org.eclipse.collections.api.bag.MutableBagIterable;
import org.eclipse.collections.api.block.function.Function;
import org.eclipse.collections.api.block.function.Function0;
import org.eclipse.collections.api.block.function.Function2;
import org.eclipse.collections.api.block.function.primitive.DoubleObjectToDoubleFunction;
import org.eclipse.collections.api.block.function.primitive.IntObjectToIntFunction;
import org.eclipse.collections.api.block.function.primitive.LongObjectToLongFunction;
import org.eclipse.collections.api.block.predicate.Predicate;
import org.eclipse.collections.api.block.predicate.Predicate2;
import org.eclipse.collections.api.block.procedure.Procedure;
import org.eclipse.collections.api.block.procedure.Procedure2;
import org.eclipse.collections.api.block.procedure.primitive.ObjectIntProcedure;
import org.eclipse.collections.api.multimap.MutableMultimap;
import org.eclipse.collections.api.ordered.OrderedIterable;
import org.eclipse.collections.api.partition.set.PartitionImmutableSet;
import org.eclipse.collections.api.set.ImmutableSet;
import org.eclipse.collections.api.tuple.Pair;
import org.eclipse.collections.impl.EmptyIterator;
import org.eclipse.collections.impl.factory.Bags;
import org.eclipse.collections.impl.factory.Sets;
import org.eclipse.collections.impl.partition.set.PartitionUnifiedSet;
/**
* This is a zero element {@link ImmutableSet} which is created by calling the Sets.immutable.empty() method.
*/
final class ImmutableEmptySet
extends AbstractImmutableSet
implements Serializable
{
static final ImmutableSet> INSTANCE = new ImmutableEmptySet<>();
private static final PartitionImmutableSet> EMPTY = new PartitionUnifiedSet<>().toImmutable();
private static final long serialVersionUID = 1L;
@Override
public boolean equals(Object other)
{
if (other == this)
{
return true;
}
return other instanceof Set && ((Set>) other).isEmpty();
}
@Override
public int hashCode()
{
return 0;
}
@Override
public ImmutableSet newWith(T element)
{
return Sets.immutable.with(element);
}
@Override
public ImmutableSet newWithAll(Iterable extends T> elements)
{
return Sets.immutable.withAll(elements);
}
@Override
public ImmutableSet newWithout(T element)
{
return this;
}
@Override
public ImmutableSet newWithoutAll(Iterable extends T> elements)
{
return this;
}
@Override
public int size()
{
return 0;
}
@Override
public boolean contains(Object obj)
{
return false;
}
@Override
public ImmutableSet tap(Procedure super T> procedure)
{
return this;
}
@Override
public void each(Procedure super T> procedure)
{
}
@Override
public void forEachWithIndex(ObjectIntProcedure super T> objectIntProcedure)
{
}
@Override
public void forEachWith(Procedure2 super T, ? super P> procedure, P parameter)
{
}
@Override
public T getFirst()
{
return null;
}
@Override
public T getLast()
{
return null;
}
@Override
public T getOnly()
{
throw new IllegalStateException("Size must be 1 but was " + this.size());
}
@Override
public Iterator iterator()
{
return EmptyIterator.getInstance();
}
@Override
public T min(Comparator super T> comparator)
{
throw new NoSuchElementException();
}
@Override
public T max(Comparator super T> comparator)
{
throw new NoSuchElementException();
}
@Override
public T min()
{
throw new NoSuchElementException();
}
@Override
public T max()
{
throw new NoSuchElementException();
}
@Override
public > T minBy(Function super T, ? extends V> function)
{
throw new NoSuchElementException();
}
@Override
public > T maxBy(Function super T, ? extends V> function)
{
throw new NoSuchElementException();
}
/**
* @deprecated in 6.0. Use {@link OrderedIterable#zip(Iterable)} instead.
*/
@Deprecated
@Override
public ImmutableSet> zip(Iterable that)
{
return Sets.immutable.empty();
}
@Override
public >> R zip(Iterable that, R target)
{
return target;
}
/**
* @deprecated in 6.0. Use {@link OrderedIterable#zipWithIndex()} instead.
*/
@Deprecated
@Override
public ImmutableSet> zipWithIndex()
{
return Sets.immutable.empty();
}
@Override
public >> R zipWithIndex(R target)
{
return target;
}
@Override
public ImmutableSet select(Predicate super T> predicate)
{
return this;
}
@Override
public ImmutableSet reject(Predicate super T> predicate)
{
return this;
}
@Override
public PartitionImmutableSet partition(Predicate super T> predicate)
{
return (PartitionImmutableSet) EMPTY;
}
@Override
public ImmutableSet collect(Function super T, ? extends V> function)
{
return Sets.immutable.empty();
}
@Override
public ImmutableSet collectIf(Predicate super T> predicate, Function super T, ? extends V> function)
{
return Sets.immutable.empty();
}
@Override
public ImmutableSet flatCollect(Function super T, ? extends Iterable> function)
{
return Sets.immutable.empty();
}
/**
* @since 9.0
*/
@Override
public ImmutableBag countBy(Function super T, ? extends V> function)
{
return Bags.immutable.empty();
}
/**
* @since 9.0
*/
@Override
public > R countBy(Function super T, ? extends V> function, R target)
{
return target;
}
/**
* @since 9.0
*/
@Override
public ImmutableBag countByWith(Function2 super T, ? super P, ? extends V> function, P parameter)
{
return Bags.immutable.empty();
}
/**
* @since 9.0
*/
@Override
public > R countByWith(Function2 super T, ? super P, ? extends V> function, P parameter, R target)
{
return target;
}
@Override
public > R groupBy(Function super T, ? extends V> function, R target)
{
return target;
}
@Override
public > R groupByEach(Function super T, ? extends Iterable> function, R target)
{
return target;
}
@Override
public boolean isEmpty()
{
return true;
}
@Override
public boolean notEmpty()
{
return false;
}
@Override
public > R select(Predicate super T> predicate, R target)
{
return target;
}
@Override
public > R selectWith(Predicate2 super T, ? super P> predicate, P parameter, R target)
{
return target;
}
@Override
public > R reject(Predicate super T> predicate, R target)
{
return target;
}
@Override
public > R rejectWith(Predicate2 super T, ? super P> predicate, P parameter, R target)
{
return target;
}
@Override
public > R collect(Function super T, ? extends V> function, R target)
{
return target;
}
@Override
public > R collectWith(Function2 super T, ? super P, ? extends V> function, P parameter, R target)
{
return target;
}
@Override
public > R collectIf(Predicate super T> predicate, Function super T, ? extends V> function, R target)
{
return target;
}
@Override
public T detectIfNone(Predicate super T> predicate, Function0 extends T> function)
{
return function.value();
}
@Override
public > R flatCollect(Function super T, ? extends Iterable> function, R target)
{
return target;
}
@Override
public T detect(Predicate super T> predicate)
{
return null;
}
@Override
public Optional detectOptional(Predicate super T> predicate)
{
return Optional.empty();
}
@Override
public int count(Predicate super T> predicate)
{
return 0;
}
@Override
public int countWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return 0;
}
@Override
public boolean anySatisfy(Predicate super T> predicate)
{
return false;
}
@Override
public boolean allSatisfy(Predicate super T> predicate)
{
return true;
}
@Override
public boolean noneSatisfy(Predicate super T> predicate)
{
return true;
}
@Override
public
boolean anySatisfyWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return false;
}
@Override
public
boolean allSatisfyWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return true;
}
@Override
public
boolean noneSatisfyWith(Predicate2 super T, ? super P> predicate, P parameter)
{
return true;
}
@Override
public IV injectInto(IV injectedValue, Function2 super IV, ? super T, ? extends IV> function)
{
return injectedValue;
}
@Override
public int injectInto(int injectedValue, IntObjectToIntFunction super T> intObjectToIntFunction)
{
return injectedValue;
}
@Override
public long injectInto(long injectedValue, LongObjectToLongFunction super T> longObjectToLongFunction)
{
return injectedValue;
}
@Override
public double injectInto(double injectedValue, DoubleObjectToDoubleFunction super T> doubleObjectToDoubleFunction)
{
return injectedValue;
}
@Override
public String toString()
{
return "[]";
}
@Override
public String makeString()
{
return "";
}
@Override
public String makeString(String separator)
{
return "";
}
@Override
public String makeString(String start, String separator, String end)
{
return start + end;
}
@Override
public void appendString(Appendable appendable)
{
}
@Override
public void appendString(Appendable appendable, String separator)
{
}
@Override
public void appendString(Appendable appendable, String start, String separator, String end)
{
try
{
appendable.append(start);
appendable.append(end);
}
catch (IOException e)
{
throw new RuntimeException(e);
}
}
private Object writeReplace()
{
return new ImmutableSetSerializationProxy<>(this);
}
}