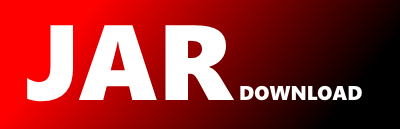
org.flowable.dmn.api.DecisionExecutionAuditContainer Maven / Gradle / Ivy
/* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.flowable.dmn.api;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.flowable.dmn.model.DecisionRule;
import org.flowable.dmn.model.HitPolicy;
import org.joda.time.DateTime;
import org.joda.time.LocalDate;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
/**
* @author Yvo Swillens
* @author Erik Winlof
*/
@JsonInclude(Include.NON_NULL)
public class DecisionExecutionAuditContainer {
protected String decisionKey;
protected String decisionName;
protected String hitPolicy;
protected String dmnDeploymentId;
protected Date startTime;
protected Date endTime;
protected Map inputVariables;
protected Map inputVariableTypes;
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy