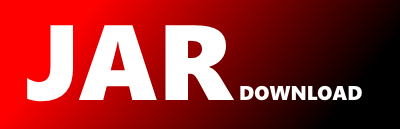
org.eclipse.emf.teneo.hibernate.HbUtil Maven / Gradle / Ivy
/**
*
*
* Copyright (c) 2005, 2006, 2007, 2008 Springsite BV (The Netherlands) and others
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Martin Taal
*
*
* $Id: HbUtil.java,v 1.38 2010/11/12 14:39:12 mtaal Exp $
*/
package org.eclipse.emf.teneo.hibernate;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.eclipse.emf.ecore.EAttribute;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.EReference;
import org.eclipse.emf.ecore.EStructuralFeature;
import org.eclipse.emf.ecore.util.FeatureMapUtil;
import org.eclipse.emf.teneo.Constants;
import org.eclipse.emf.teneo.ERuntime;
import org.eclipse.emf.teneo.PackageRegistryProvider;
import org.eclipse.emf.teneo.hibernate.mapper.HbMapperConstants;
import org.eclipse.emf.teneo.hibernate.mapping.econtainer.NewEContainerFeatureIDPropertyHandler;
import org.eclipse.emf.teneo.hibernate.mapping.identifier.IdentifierCacheHandler;
import org.eclipse.emf.teneo.hibernate.mapping.identifier.IdentifierPropertyHandler;
import org.eclipse.emf.teneo.hibernate.mapping.identifier.IdentifierUtil;
import org.eclipse.emf.teneo.hibernate.mapping.property.EAttributePropertyHandler;
import org.eclipse.emf.teneo.hibernate.mapping.property.EReferencePropertyHandler;
import org.eclipse.emf.teneo.hibernate.mapping.property.SyntheticIndexPropertyHandler;
import org.eclipse.emf.teneo.hibernate.mapping.property.SyntheticPropertyHandler;
import org.eclipse.emf.teneo.util.StoreUtil;
import org.hibernate.Session;
import org.hibernate.cfg.Environment;
import org.hibernate.engine.spi.SessionImplementor;
import org.hibernate.mapping.Collection;
import org.hibernate.mapping.Component;
import org.hibernate.mapping.MetaAttribute;
import org.hibernate.mapping.PersistentClass;
import org.hibernate.mapping.Property;
import org.hibernate.persister.entity.EntityPersister;
import org.hibernate.property.EmbeddedPropertyAccessor;
import org.hibernate.property.PropertyAccessor;
import org.hibernate.proxy.HibernateProxy;
import org.hibernate.type.IdentifierType;
import org.hibernate.type.PrimitiveType;
import org.hibernate.type.StringType;
import org.hibernate.type.Type;
/**
* Contains some utility methods.
*
* @author Martin Taal
* @version $Revision: 1.38 $
*/
public class HbUtil {
/** The logger */
private static Log log = LogFactory.getLog(HbUtil.class);
/**
* Returns the name stored in the teneo annotation using the
* {@link Constants#ANNOTATION_KEY_ENTITY_NAME} key.
*/
public static String getEntityName(EClass eClass) {
return eClass.getEAnnotation(Constants.ANNOTATION_SOURCE_TENEO_JPA).getDetails()
.get(Constants.ANNOTATION_KEY_ENTITY_NAME);
}
public static EClass getEClassFromMeta(Component component) {
final MetaAttribute ePackageMetaAttribute = component
.getMetaAttribute(HbMapperConstants.EPACKAGE_PARAM);
if (ePackageMetaAttribute == null) {
return null;
}
final EPackage epackage = PackageRegistryProvider.getInstance().getPackageRegistry()
.getEPackage(ePackageMetaAttribute.getValue());
if (epackage == null) {
throw new IllegalArgumentException("Could not find ePackage using nsuri "
+ ePackageMetaAttribute.getValue());
}
final MetaAttribute eClassMetaAttribute = component
.getMetaAttribute(HbMapperConstants.ECLASS_NAME_META);
if (eClassMetaAttribute == null) {
return null;
}
return (EClass) epackage.getEClassifier(eClassMetaAttribute.getValue());
}
public static EClass getEClassFromMeta(EPackage.Registry registry, PersistentClass persistentClass) {
final MetaAttribute ePackageMetaAttribute = persistentClass
.getMetaAttribute(HbMapperConstants.EPACKAGE_PARAM);
if (ePackageMetaAttribute == null) {
return null;
}
final EPackage epackage = registry.getEPackage(ePackageMetaAttribute.getValue());
if (epackage == null) {
throw new IllegalArgumentException("Could not find ePackage using nsuri "
+ ePackageMetaAttribute.getValue());
}
final MetaAttribute eClassMetaAttribute = persistentClass
.getMetaAttribute(HbMapperConstants.ECLASS_NAME_META);
if (eClassMetaAttribute == null) {
return null;
}
return (EClass) epackage.getEClassifier(eClassMetaAttribute.getValue());
}
/**
* A merge method which does not use Session.merge but uses the EMF api to travers object
* references and execute merge through the object tree. The merge will traverse all EReferences.
* The maximum level it will traverse is passed in as the maxMergeLevel parameters.
*
* @param session
* the hibernate session
* @param eObject
* the eObject to merge
* @return the object read from the database with Merge support.
*/
public static EObject merge(Session session, EObject eObject, int maxMergeLevel) {
SessionImplementor sessionImplementor = (SessionImplementor) session;
return merge(sessionImplementor, eObject, new HashMap(), maxMergeLevel, 0);
}
private static EObject merge(SessionImplementor sessionImplementor, EObject eObject,
Map copyCache, int maxMergeLevel, int currentMergeLevel) {
if (copyCache.containsKey(eObject)) {
return copyCache.get(eObject);
}
final String entityName = sessionImplementor.guessEntityName(eObject);
final EntityPersister persister = sessionImplementor.getEntityPersister(entityName, eObject);
final Serializable id = persister.getIdentifier(eObject, sessionImplementor);
if (id != null) {
final EObject source = (EObject) ((Session) sessionImplementor).get(entityName, id);
copyCache.put(eObject, source);
if (maxMergeLevel == currentMergeLevel) {
return source;
}
// now copy the features
for (EStructuralFeature eFeature : source.eClass().getEAllStructuralFeatures()) {
if (eFeature instanceof EAttribute) {
source.eSet(eFeature, eObject.eGet(eFeature));
} else if (eFeature.isMany()) {
final List newValues = new ArrayList();
@SuppressWarnings("unchecked")
final List currentValues = (List) eObject.eGet(eFeature);
for (EObject currentValue : currentValues) {
newValues.add(merge(sessionImplementor, currentValue, copyCache, maxMergeLevel,
currentMergeLevel + 1));
}
source.eSet(eFeature, newValues);
} else {
source.eSet(
eFeature,
merge(sessionImplementor, (EObject) eObject.eGet(eFeature), copyCache, maxMergeLevel,
currentMergeLevel + 1));
}
}
return source;
} else {
return eObject;
}
}
public static boolean isEAVMapped(PersistentClass mappedEntity) {
if (mappedEntity.getEntityName() != null
&& mappedEntity.getEntityName().equals(Constants.EAV_EOBJECT_ENTITY_NAME)) {
return true;
}
if (mappedEntity.getSuperclass() == null) {
return false;
}
return isEAVMapped(mappedEntity.getSuperclass());
}
/** Encode the id of an eobject */
@SuppressWarnings("unchecked")
public static String idToString(EObject eobj, HbDataStore hd, Session session) {
final PersistentClass pc = hd.getPersistentClass(hd.toEntityName(
eobj.eClass()));
if (pc == null) { // can happen with map entries
return null;
}
Object id;
if (eobj instanceof HibernateProxy) {
id = ((HibernateProxy) eobj).getHibernateLazyInitializer().getIdentifier();
} else {
id = IdentifierUtil.getID(eobj, hd, (SessionImplementor) session);
}
if (id == null) {
id = IdentifierCacheHandler.getInstance().getID(eobj);
if (id == null) {
return null;
}
}
final Type t = pc.getIdentifierProperty().getType();
if (t instanceof PrimitiveType) {
return ((PrimitiveType
© 2015 - 2025 Weber Informatics LLC | Privacy Policy