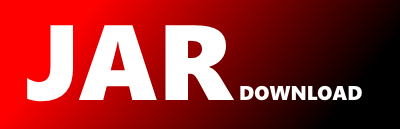
org.eclipse.epsilon.emc.emf.m0.EmfM0Model Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (c) 2008 The University of York.
* This program and the accompanying materials
* are made available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* Contributors:
* Dimitrios Kolovos - initial API and implementation
******************************************************************************/
package org.eclipse.epsilon.emc.emf.m0;
import java.io.File;
import java.util.ArrayList;
import java.util.Collection;
import java.util.ListIterator;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.epsilon.common.util.StringProperties;
import org.eclipse.epsilon.emc.emf.EmfModel;
import org.eclipse.epsilon.eol.EolModule;
import org.eclipse.epsilon.eol.IEolModule;
import org.eclipse.epsilon.eol.dom.Operation;
import org.eclipse.epsilon.eol.exceptions.EolIllegalPropertyException;
import org.eclipse.epsilon.eol.exceptions.EolRuntimeException;
import org.eclipse.epsilon.eol.exceptions.models.EolModelElementTypeNotFoundException;
import org.eclipse.epsilon.eol.exceptions.models.EolModelLoadingException;
import org.eclipse.epsilon.eol.execute.introspection.AbstractPropertyGetter;
import org.eclipse.epsilon.eol.execute.introspection.AbstractPropertySetter;
import org.eclipse.epsilon.eol.execute.introspection.IPropertyGetter;
import org.eclipse.epsilon.eol.execute.introspection.IReflectivePropertySetter;
import org.eclipse.epsilon.eol.models.IRelativePathResolver;
public class EmfM0Model extends EmfModel {
protected File m0SpecificationFile;
protected IEolModule eolModule;
public EmfM0Model() {
super();
}
@Override
public void load(StringProperties properties, IRelativePathResolver resolver) throws EolModelLoadingException {
this.m0SpecificationFile = new File(resolver.resolve(properties.getProperty("m0SpecificationFile")));
super.load(properties, resolver);
// this.name = properties.getProperty("name");
// String[] aliases = properties.getProperty("aliases").split(",");
// for (int i=0;i li = eolModule.getDeclaredOperations().listIterator();
while (li.hasNext()){
Operation helper = (Operation) li.next();
if (helper.getName().equals(name)){
return helper;
}
}
return null;
}
class EmfM0PropertyGetter extends AbstractPropertyGetter {
public Object invoke(Object object, String property) throws EolRuntimeException {
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy