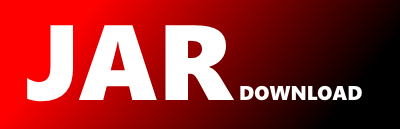
org.eclipse.epsilon.flexmi.AssignmentCalculator Maven / Gradle / Ivy
/*********************************************************************
* Copyright (c) 2008 The University of York.
*
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
**********************************************************************/
package org.eclipse.epsilon.flexmi;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class AssignmentCalculator {
public static void main(String[] args) {
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy