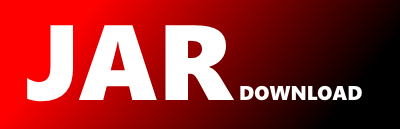
org.eclipse.epsilon.flexmi.AttributeStructuralFeatureAllocator Maven / Gradle / Ivy
The newest version!
/*********************************************************************
* Copyright (c) 2008 The University of York.
*
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
**********************************************************************/
package org.eclipse.epsilon.flexmi;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.eclipse.emf.ecore.EAttribute;
import org.eclipse.emf.ecore.EStructuralFeature;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
public class AttributeStructuralFeatureAllocator {
protected StringSimilarityProvider stringSimilarityProvider = new CachedStringSimilarityProvider(new DefaultStringSimilarityProvider());
public static void main(String[] args) {
Map allocation = new AttributeStructuralFeatureAllocator().allocate(Arrays.asList("b", "c", "name"), Arrays.asList("a", "b", "nc"));
for (String key : allocation.keySet()) {
System.out.println(key + "->" + allocation.get(key));
}
}
public Map allocate(NamedNodeMap attributes, List structuralFeatures) {
List attributeNames = new ArrayList();
for (int i=0;i structuralFeatureNames = new ArrayList();
for (EStructuralFeature feature : structuralFeatures) {
structuralFeatureNames.add(feature.getName());
}
Map nameAllocation = allocate(attributeNames, structuralFeatureNames);
HashMap allocation = new HashMap();
for (String attributeName : nameAllocation.keySet()) {
String structuralFeatureName = nameAllocation.get(attributeName);
EStructuralFeature feature = null;
for (EStructuralFeature f : structuralFeatures) {
if (structuralFeatureName.equals(f.getName())) {
feature = f;
break;
}
}
allocation.put(attributes.getNamedItem(attributeName), feature);
}
return allocation;
}
public Map allocate(List values, List slots) {
AllocationTree tree = new AllocationTree();
tree.allocate(values, slots);
int bestSimilarity = -1;
AllocationTree bestAllocation = null;
for (AllocationTree leaf : tree.getLeafs()) {
int similarity = 0;
for (Allocation allocation : leaf.getAllAllocations()) {
similarity += stringSimilarityProvider.getSimilarity(allocation.getSlot(), allocation.getValue());
}
if (similarity > bestSimilarity) {
bestAllocation = leaf;
bestSimilarity = similarity;
}
}
HashMap result = new HashMap();
if (bestAllocation != null) {
for (Allocation allocation : bestAllocation.getAllAllocations()) {
result.put(allocation.getValue(), allocation.getSlot());
}
}
return result;
}
class AllocationTree {
protected List children = new ArrayList();
protected Allocation allocation = null;
protected AllocationTree parent;
public AllocationTree() {
this(null);
}
public AllocationTree(AllocationTree parent) {
this.parent = parent;
if (parent != null) parent.children.add(this);
}
public void allocate(List values, List slots) {
for (String value : values) {
for (String slot : slots) {
AllocationTree child = new AllocationTree(this);
child.setAllocation(new Allocation(slot, value));
}
}
for (AllocationTree childTree : getChildren()) {
List newSlots = new ArrayList(slots);
newSlots.remove(childTree.getAllocation().getSlot());
List newValues = new ArrayList(values);
newValues.remove(childTree.getAllocation().getValue());
childTree.allocate(newValues, newSlots);
}
}
public Allocation getAllocation() {
return allocation;
}
public void setAllocation(Allocation allocation) {
this.allocation = allocation;
}
public List getChildren() {
return Collections.unmodifiableList(children);
}
public AllocationTree getParent() {
return parent;
}
public void print() {
print(-1);
}
protected void print(int indentation) {
for (int i=0;i" + allocation.getSlot());
for (AllocationTree child : getChildren()) {
child.print(indentation + 1);
}
}
public boolean isLeaf() {
return getChildren().isEmpty();
}
public List getLeafs() {
if (isLeaf()) {
return Arrays.asList(this);
}
else {
ArrayList leafs = new ArrayList();
for (AllocationTree childTree : getChildren()) {
leafs.addAll(childTree.getLeafs());
}
return leafs;
}
}
public List getAllAllocations() {
List allAllocations = new ArrayList();
if (allocation != null) allAllocations.add(allocation);
AllocationTree parent = this.parent;
while (parent != null) {
allAllocations.addAll(parent.getAllAllocations());
parent = parent.getParent();
}
return Collections.unmodifiableList(allAllocations);
}
}
class Allocation {
protected String value;
protected String slot;
public Allocation(String slot, String value) {
super();
this.slot = slot;
this.value = value;
}
public String getSlot() {
return slot;
}
public void setSlot(String slot) {
this.slot = slot;
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy