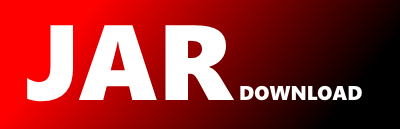
hudson.MarkupText Maven / Gradle / Ivy
Show all versions of hudson-core Show documentation
/*******************************************************************************
*
* Copyright (c) 2004-2010 Oracle Corporation.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
*
* Kohsuke Kawaguchi
*
*
*******************************************************************************/
package hudson;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Mutable representation of string with HTML mark up.
*
*
* This class is used to put mark up on plain text.
* See
* the test code for a typical usage and its result.
*
* @author Kohsuke Kawaguchi
* @since 1.70
*/
public class MarkupText extends AbstractMarkupText {
private final String text;
/**
* Added mark up tags.
*/
private final List tags = new ArrayList();
/**
* Represents one mark up inserted into text.
*/
private static final class Tag implements Comparable {
/**
* Char position of this tag in {@link MarkupText#text}.
* This tag is placed in front of the character of this index.
*/
private final int pos;
private final String markup;
public Tag(int pos, String markup) {
this.pos = pos;
this.markup = markup;
}
public int compareTo(Tag that) {
return this.pos-that.pos;
}
}
/**
* Represents a substring of a {@link MarkupText}.
*/
public final class SubText extends AbstractMarkupText {
private final int start,end;
private final int[] groups;
public SubText(Matcher m, int textOffset) {
start = m.start() + textOffset;
end = m.end() + textOffset;
int cnt = m.groupCount();
groups = new int[cnt*2];
for( int i=0; i
* Start/end tag text can contain special tokens "$0", "$1", ...
* and they will be replaced by their {@link #group(int) group match}.
* "\$" can be used to escape characters.
*/
public void surroundWith(String startTag, String endTag) {
addMarkup(0,length(),replace(startTag),replace(endTag));
}
/**
* Works like {@link #surroundWith(String, String)} except
* that the token replacement is not performed on parameters.
*/
public void surroundWithLiteral(String startTag, String endTag) {
addMarkup(0,length(),startTag,endTag);
}
/**
* Surrounds this subtext with <a>...</a>.
*/
public void href(String url) {
addHyperlink(0,length(),url);
}
/**
* Gets the start index of the captured group within {@link MarkupText#getText()}.
*
* @param groupIndex
* 0 means the start of the whole subtext. 1, 2, ... are
* groups captured by '(...)' in the regexp.
*/
public int start(int groupIndex) {
if(groupIndex==0) return start;
return groups[groupIndex*2-2];
}
/**
* Gets the start index of this subtext within {@link MarkupText#getText()}.
*/
public int start() {
return start;
}
/**
* Gets the end index of the captured group within {@link MarkupText#getText()}.
*/
public int end(int groupIndex) {
if(groupIndex==0) return end;
return groups[groupIndex*2-1];
}
/**
* Gets the end index of this subtext within {@link MarkupText#getText()}.
*/
public int end() {
return end;
}
/**
* Gets the text that represents the captured group.
*/
public String group(int groupIndex) {
if(start(groupIndex)==-1)
return null;
return text.substring(start(groupIndex),end(groupIndex));
}
/**
* How many captured groups are in this subtext.
* @since 1.357
*/
public int groupCount() {
return groups.length / 2;
}
/**
* Replaces the group tokens like "$0", "$1", and etc with their actual matches.
*/
public String replace(String s) {
StringBuffer buf = new StringBuffer();
for( int i=0; i 9) {
buf.append('$').append(ch);
} else {
// add the group text
String group = group(groupId);
if (group != null)
buf.append(group);
}
} else {
// other chars
buf.append(ch);
}
}
return buf.toString();
}
@Override
protected SubText createSubText(Matcher m) {
return new SubText(m,start);
}
}
/**
*
* @param text
* Plain text. This shouldn't include any markup nor escape. Those are done later in {@link #toString(boolean)}.
*/
public MarkupText(String text) {
this.text = text;
}
@Override
public String getText() {
return text;
}
/**
* Returns a subtext.
*
* @param end
* If negative, -N means "trim the last N-1 chars". That is, (s,-1) is the same as (s,length)
*/
public SubText subText(int start, int end) {
return new SubText(start, end<0 ? text.length()+1+end : end);
}
@Override
public void addMarkup( int startPos, int endPos, String startTag, String endTag ) {
rangeCheck(startPos);
rangeCheck(endPos);
if(startPos>endPos) throw new IndexOutOfBoundsException();
// when multiple tags are added to the same range, we want them to show up like
// abc, not abc. Also, we'd like abcdef,
// not abcdef. Do this by inserting them to different places.
tags.add(new Tag(startPos, startTag));
tags.add(0,new Tag(endPos,endTag));
}
public void addMarkup(int pos, String tag) {
rangeCheck(pos);
tags.add(new Tag(pos,tag));
}
private void rangeCheck(int pos) {
if(pos<0 || pos>text.length())
throw new IndexOutOfBoundsException();
}
/**
* Returns the fully marked-up text.
*
* @deprecated as of 1.350.
* Use {@link #toString(boolean)} to be explicit about the escape mode.
*/
@Override
public String toString() {
return toString(false);
}
/**
* Returns the fully marked-up text.
*
* @param preEscape
* If true, the escaping is for the <PRE> context. This leave SP and CR/LF intact.
* If false, the escape is for the normal HTML, thus SP becomes and CR/LF becomes <BR>
*/
public String toString(boolean preEscape) {
if(tags.isEmpty())
return preEscape? Util.xmlEscape(text) : Util.escape(text); // the most common case
Collections.sort(tags);
StringBuilder buf = new StringBuilder();
int copied = 0; // # of chars already copied from text to buf
for (Tag tag : tags) {
if (copied findTokens(Pattern pattern) {
return super.findTokens(pattern);
}
@Override
protected SubText createSubText(Matcher m) {
return new SubText(m,0);
}
}