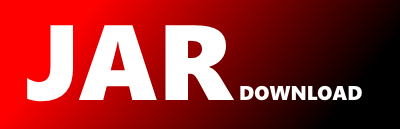
hudson.console.UrlAnnotator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hudson-core Show documentation
Show all versions of hudson-core Show documentation
Contains the core Hudson code and view files to render HTML.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2004-2010 Oracle Corporation.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
*
*
*******************************************************************************/
package hudson.console;
import hudson.Extension;
import hudson.MarkupText;
import hudson.MarkupText.SubText;
import java.util.regex.Pattern;
/**
* Annotates URLs in the console output to hyperlink.
*
* @author Kohsuke Kawaguchi
*/
@Extension
public class UrlAnnotator extends ConsoleAnnotatorFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy