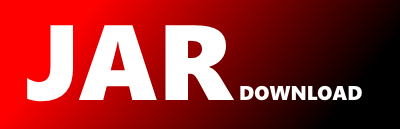
hudson.model.ParameterDefinition Maven / Gradle / Ivy
Show all versions of hudson-core Show documentation
/*******************************************************************************
*
* Copyright (c) 2004-2009 Oracle Corporation.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
*
* Kohsuke Kawaguchi, Luca Domenico Milanesio, Tom Huybrechts
*
*
*******************************************************************************/
package hudson.model;
import hudson.DescriptorExtensionList;
import hudson.Extension;
import hudson.ExtensionPoint;
import hudson.AbortException;
import hudson.cli.CLICommand;
import hudson.util.DescriptorList;
import java.io.Serializable;
import java.io.IOException;
import net.sf.json.JSONObject;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.kohsuke.stapler.StaplerRequest;
import org.kohsuke.stapler.export.Exported;
import org.kohsuke.stapler.export.ExportedBean;
/**
* Defines a parameter for a build.
*
*
* In Hudson, a user can configure a job to require parameters for a build.
* For example, imagine a test job that takes the bits to be tested as a parameter.
*
*
* The actual meaning and the purpose of parameters are entirely up to users, so
* what the concrete parameter implmentation is pluggable. Write subclasses
* in a plugin and put {@link Extension} on the descriptor to register them.
*
*
* Three classes are used to model build parameters. First is the
* {@link ParameterDescriptor}, which tells Hudson what kind of implementations are
* available. From {@link ParameterDescriptor#newInstance(StaplerRequest, JSONObject)},
* Hudson creates {@link ParameterDefinition}s based on the job configuration.
* For example, if the user defines two string parameters "database-type" and
* "appserver-type", we'll get two {@link StringParameterDefinition} instances
* with their respective names.
*
*
* When a job is configured with {@link ParameterDefinition} (or more precisely,
* {@link ParametersDefinitionProperty}, which in turns retains {@link ParameterDefinition}s),
* user would have to enter the values for the defined build parameters.
* The {@link #createValue(StaplerRequest, JSONObject)} method is used to convert this
* form submission into {@link ParameterValue} objects, which are then accessible
* during a build.
*
*
*
*
Persistence
*
* Instances of {@link ParameterDefinition}s are persisted into job config.xml
* through XStream.
*
*
*
Assocaited Views
* config.jelly
*
* {@link ParameterDefinition} class uses config.jelly to provide contribute a form
* fragment in the job configuration screen. Values entered there is fed back to
* {@link ParameterDescriptor#newInstance(StaplerRequest, JSONObject)} to create {@link ParameterDefinition}s.
*
*
index.jelly
* The index.jelly view contributes a form fragment in the page where the user
* enters actual values of parameters for a build. The result of this form submission
* is then fed to {@link ParameterDefinition#createValue(StaplerRequest, JSONObject)} to
* create {@link ParameterValue}s.
*
* TODO: what Jelly pages does this object need for rendering UI?
* TODO: {@link ParameterValue} needs to have some mechanism to expose values to the build
* @see StringParameterDefinition
*/
@ExportedBean(defaultVisibility=3)
public abstract class ParameterDefinition implements
Describable, ExtensionPoint, Serializable {
private final String name;
private final String description;
public ParameterDefinition(String name) {
this(name, null);
}
public ParameterDefinition(String name, String description) {
this.name = name;
this.description = description;
}
@Exported
public String getType() {
return this.getClass().getSimpleName();
}
@Exported
public String getName() {
return name;
}
@Exported
public String getDescription() {
return description;
}
/**
* {@inheritDoc}
*/
public ParameterDescriptor getDescriptor() {
return (ParameterDescriptor)Hudson.getInstance().getDescriptorOrDie(getClass());
}
/**
* Create a parameter value from a form submission.
*
*
* This method is invoked when the user fills in the parameter values in the HTML form
* and submits it to the server.
*/
public abstract ParameterValue createValue(StaplerRequest req, JSONObject jo);
/**
* Create a parameter value from a GET with query string.
* If no value is available in the request, it returns a default value if possible, or null.
*
*
* Unlike {@link #createValue(StaplerRequest, JSONObject)}, this method is intended to support
* the programmatic POST-ing of the build URL. This form is less expressive (as it doesn't support
* the tree form), but it's more scriptable.
*
*
* If a {@link ParameterDefinition} can't really support this mode of creating a value,
* you may just always return null.
*/
public abstract ParameterValue createValue(StaplerRequest req);
/**
* Create a parameter value from the string given in the CLI.
*
* @param command
* This is the command that got the parameter. You can use its {@link CLICommand#channel}
* for interacting with the CLI JVM.
* @throws AbortException
* If the CLI processing should be aborted. Hudson will report the error message
* without stack trace, and then exits this command. Useful for graceful termination.
* @throws Exception
* All the other exceptions cause the stack trace to be dumped, and then
* the command exits with an error code.
* @since 1.334
*/
public ParameterValue createValue(CLICommand command, String value) throws IOException, InterruptedException {
throw new AbortException("CLI parameter submission is not supported for the "+getClass()+" type. Please file a bug report for this");
}
/**
* Returns default parameter value for this definition.
*
* @return default parameter value or null if no defaults are available
* @since 1.253
*/
@Exported
public ParameterValue getDefaultParameterValue() {
return null;
}
/**
* Returns all the registered {@link ParameterDefinition} descriptors.
*/
public static DescriptorExtensionList all() {
return Hudson.getInstance().getDescriptorList(ParameterDefinition.class);
}
/**
* A list of available parameter definition types
* @deprecated as of 1.286
* Use {@link #all()} for read access, and {@link Extension} for registration.
*/
public static final DescriptorList LIST = new DescriptorList(ParameterDefinition.class);
public abstract static class ParameterDescriptor extends
Descriptor {
protected ParameterDescriptor(Class extends ParameterDefinition> klazz) {
super(klazz);
}
/**
* Infers the type of the corresponding {@link ParameterDescriptor} from the outer class.
* This version works when you follow the common convention, where a descriptor
* is written as the static nested class of the describable class.
*
* @since 1.278
*/
protected ParameterDescriptor() {
}
public String getValuePage() {
return getViewPage(clazz, "index.jelly");
}
@Override
public String getDisplayName() {
return "Parameter";
}
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ParameterDefinition that = (ParameterDefinition) o;
if (name != null ? !name.equals(that.name) : that.name != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(getName())
.append(getClass())
.toHashCode();
}
}