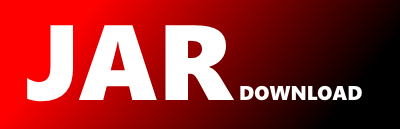
org.eclipse.jetty.npn.NextProtoNego Maven / Gradle / Ivy
/*
* Copyright (c) 2012 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.eclipse.jetty.npn;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.WeakHashMap;
import javax.net.ssl.SSLEngine;
import javax.net.ssl.SSLSocket;
/**
* {@link NextProtoNego} provides an API to applications that want to make use of the
* Next Protocol Negotiation.
* The NPN extension is only available when using the TLS protocol, therefore applications must
* ensure that the TLS protocol is used:
*
* SSLContext context = SSLContext.getInstance("TLSv1");
*
* Refer to the
* list
* of standard SSLContext protocol names for further information on TLS protocol versions supported.
* Applications must register instances of either {@link SSLSocket} or {@link SSLEngine} with a
* {@link ClientProvider} or with a {@link ServerProvider}, depending whether they are on client or
* server side.
* The NPN implementation will invoke the provider callbacks to allow applications to interact
* with the negotiation of the next protocol.
* Client side typical usage:
*
* SSLSocket sslSocket = ...;
* NextProtoNego.put(sslSocket, new NextProtoNego.ClientProvider()
* {
* @Override
* public boolean supports()
* {
* return true;
* }
*
* @Override
* public void unsupported()
* {
* }
*
* @Override
* public String selectProtocol(List<String> protocols)
* {
* return protocols.get(0);
* }
* });
*
* Server side typical usage:
*
* SSLSocket sslSocket = ...;
* NextProtoNego.put(sslSocket, new NextProtoNego.ServerProvider()
* {
* @Override
* public void unsupported()
* {
* }
*
* @Override
* public List protocols()
* {
* return Arrays.asList("http/1.1");
* }
*
* @Override
* public void protocolSelected(String protocol)
* {
* System.out.println("Protocol Selected is: " + protocol);
* }
* });
*
* There is no need to unregister {@link SSLSocket} or {@link SSLEngine} instances, as they
* are kept in a {@link WeakHashMap} and will be garbage collected when the application does not
* hard reference them anymore.
* In order to help application development, you can set the {@link NextProtoNego#debug} field
* to {@code true} to have debug code printed to {@link System#err}.
*/
public class NextProtoNego
{
/**
* Enables debug logging on {@link System#err}.
*/
public static boolean debug = false;
private static Map