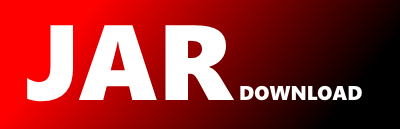
org.eclipse.jkube.maven.enricher.specific.KarafHealthCheckEnricher Maven / Gradle / Ivy
/**
* Copyright (c) 2019 Red Hat, Inc.
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at:
*
* https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* Red Hat, Inc. - initial API and implementation
*/
package org.eclipse.jkube.maven.enricher.specific;
import io.fabric8.kubernetes.api.model.Probe;
import io.fabric8.kubernetes.api.model.ProbeBuilder;
import org.eclipse.jkube.kit.common.Configs;
import org.eclipse.jkube.maven.enricher.api.JKubeEnricherContext;
import java.util.List;
import java.util.Map;
import java.util.Optional;
/**
* Enriches Karaf containers with health check probes.
*/
public class KarafHealthCheckEnricher extends AbstractHealthCheckEnricher {
private static final int DEFAULT_HEALTH_CHECK_PORT = 8181;
public KarafHealthCheckEnricher(JKubeEnricherContext buildContext) {
super(buildContext, "jkube-healthcheck-karaf");
}
private enum Config implements Configs.Key {
failureThreshold {{ d = "3"; }},
successThreshold {{ d = "1"; }};
protected String d;
public String def() {
return d;
}
}
@Override
protected Probe getReadinessProbe() {
return discoverKarafProbe("/readiness-check", 10);
}
@Override
protected Probe getLivenessProbe() {
return discoverKarafProbe("/health-check", 180);
}
//
// Karaf has a readiness/health URL exposed if the jkube-karaf-check feature is installed.
//
private Probe discoverKarafProbe(String path, int initialDelay) {
final Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy