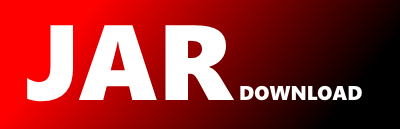
org.eclipse.jnosql.communication.keyvalue.BucketManager Maven / Gradle / Ivy
/*
*
* Copyright (c) 2023 Contributors to the Eclipse Foundation
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Apache License v2.0 is available at http://www.opensource.org/licenses/apache2.0.php.
*
* You may elect to redistribute this code under either of these licenses.
*
* Contributors:
*
* Otavio Santana
*
*/
package org.eclipse.jnosql.communication.keyvalue;
import org.eclipse.jnosql.communication.Value;
import java.time.Duration;
import java.util.Optional;
/**
* Interface used to interact with the persistence context to {@link KeyValueEntity}
* The BucketManager API is used to create and remove persistent {@link KeyValueEntity}.
*/
public interface BucketManager extends AutoCloseable {
/**
* Returns the bucket name of this {@link BucketManager}
*
* @return the bucket name
*/
String name();
/**
* Associates the specified value with the specified key and then storage
*
* @param key the key
* @param value the value
* @param the key type
* @param the value type
* @throws NullPointerException when either key or value are null
*/
void put(K key, V value);
/**
* Saves the {@link KeyValueEntity}
*
* @param entity the entity to be inserted
* @throws NullPointerException when entity is null
*/
void put(KeyValueEntity entity);
/**
* Saves the {@link KeyValueEntity} with time to live
*
* @param entity the entity to be inserted
* @param ttl the defined time to live
* @throws NullPointerException when entity is null
* @throws UnsupportedOperationException when expired time is not supported
*/
void put(KeyValueEntity entity, Duration ttl);
/**
* Saves the {@link Iterable} of keys
*
* @param entities keys to be inserted
* @throws NullPointerException when the iterable is null
*/
void put(Iterable entities);
/**
* Saves the {@link Iterable} of keys with a defined time to live
*
* @param entities keys to be inserted
* @param ttl the time to entity expire
* @throws NullPointerException when the iterable is null
* @throws UnsupportedOperationException when expired time is not supported
*/
void put(Iterable entities, Duration ttl);
/**
* Finds the Value from a key
*
* @param key the key
* @param the key type
* @return the {@link Optional} when is not found will return a {@link Optional#empty()}
* @throws NullPointerException when the key is null
*/
Optional get(K key);
/**
* Finds a list of values from keys
*
* @param keys the keys to be used in this query
* @param the key type
* @return the list of result
* @throws NullPointerException when either the keys or the entities values are null
*/
Iterable get(Iterable keys);
/**
* Removes an entity from key
*
* @param key the key bo be used
* @param the key type
* @throws NullPointerException when the key is null
*/
void delete(K key);
/**
* Removes entities from keys
*
* @param keys the keys to be used
* @param the key type
* @throws NullPointerException when the key is null
*/
void delete(Iterable keys);
/**
* closes a resource
*/
@Override
void close();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy