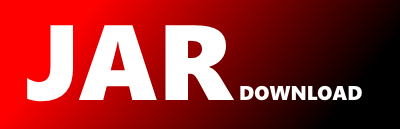
org.eclipse.jnosql.mapping.keyvalue.configuration.CollectionSupplier Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2023 Contributors to the Eclipse Foundation
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Apache License v2.0 is available at http://www.opensource.org/licenses/apache2.0.php.
*
* You may elect to redistribute this code under either of these licenses.
*
* Contributors:
*
* Otavio Santana
*/
package org.eclipse.jnosql.mapping.keyvalue.configuration;
import jakarta.data.exceptions.MappingException;
import jakarta.enterprise.context.ApplicationScoped;
import jakarta.enterprise.inject.Produces;
import jakarta.enterprise.inject.spi.InjectionPoint;
import jakarta.inject.Inject;
import org.eclipse.jnosql.communication.keyvalue.BucketManagerFactory;
import org.eclipse.jnosql.mapping.keyvalue.KeyValueDatabase;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.List;
import java.util.Map;
import java.util.Queue;
import java.util.Set;
@ApplicationScoped
public class CollectionSupplier {
@Inject
private BucketManagerFactory factory;
@Produces
@KeyValueDatabase("")
public List getList(InjectionPoint injectionPoint) {
CollectionElement element = CollectionElement.of(injectionPoint);
return factory.getList(element.bucketName, element.type);
}
@Produces
@KeyValueDatabase("")
public Queue getQueue(InjectionPoint injectionPoint) {
CollectionElement element = CollectionElement.of(injectionPoint);
return factory.getQueue(element.bucketName, element.type);
}
@Produces
@KeyValueDatabase("")
public Set getSet(InjectionPoint injectionPoint) {
CollectionElement element = CollectionElement.of(injectionPoint);
return factory.getSet(element.bucketName, element.type);
}
@Produces
@KeyValueDatabase("")
public Map getMap(InjectionPoint injectionPoint) {
MapElement element = MapElement.of(injectionPoint);
return factory.getMap(element.bucketName, element.key, element.value);
}
private record CollectionElement(String bucketName, Class type) {
@SuppressWarnings("unchecked")
private static CollectionElement of(InjectionPoint injectionPoint) {
KeyValueDatabase keyValue = injectionPoint.getQualifiers()
.stream().filter(KeyValueDatabase.class::isInstance)
.map(KeyValueDatabase.class::cast)
.findFirst().orElseThrow(() -> new MappingException("There is an issue to load " +
"a Collection from the database"));
String bucketName = keyValue.value();
if (injectionPoint.getType() instanceof ParameterizedType param) {
Type argument = param.getActualTypeArguments()[0];
return new CollectionElement<>(bucketName, (Class) argument);
}
throw new MappingException("There is an issue to load a Collection from the database");
}
}
private record MapElement(String bucketName, Class key, Class value) {
@SuppressWarnings("unchecked")
private static MapElement of(InjectionPoint injectionPoint) {
KeyValueDatabase keyValue = injectionPoint.getQualifiers()
.stream().filter(KeyValueDatabase.class::isInstance)
.map(KeyValueDatabase.class::cast)
.findFirst().orElseThrow(() -> new MappingException("There is an issue to load " +
"a Collection from the database"));
String bucketName = keyValue.value();
if (injectionPoint.getType() instanceof ParameterizedType param) {
Type key = param.getActualTypeArguments()[0];
Type value = param.getActualTypeArguments()[1];
return new MapElement<>(bucketName, (Class) key, (Class) value);
}
throw new MappingException("There is an issue to load the Map from the database");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy