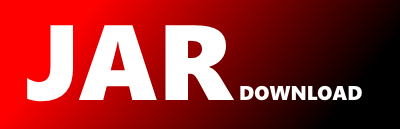
org.eclipse.microprofile.config.spi.ConfigSource Maven / Gradle / Ivy
/*
******************************************************************************
* Copyright (c) 2009-2018 Contributors to the Eclipse Foundation
*
* See the NOTICE file(s) distributed with this work for additional
* information regarding copyright ownership.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* You may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Contributors:
* 2009 - Mark Struberg
* Ordinal solution in Apache OpenWebBeans
* 2011-12-28 - Mark Struberg & Gerhard Petracek
* Contributed to Apache DeltaSpike fb0131106481f0b9a8fd
* 2016-11-14 - Emily Jiang / IBM Corp
* Methods renamed, JavaDoc and cleanup
*
*******************************************************************************/
package org.eclipse.microprofile.config.spi;
import java.util.Map;
import java.util.Set;
/**
* A configuration source which provides configuration values from a specific place. Some examples of configuration
* sources may include:
*
* - a JNDI-backed naming service
* - a properties file
* - a database table
*
* Implementations of this interface have the responsibility to get the value corresponding to a property name, and to
* enumerate available property names.
*
* A configuration source is always read-only; any potential updates of the backing configuration values must be handled
* directly inside each configuration source instance.
*
*
Default configuration sources
* Some configuration sources are known as default configuration sources. These configuration sources are
* normally available in all automatically-created configurations, and can be
* {@linkplain ConfigBuilder#addDefaultSources() manually added} to manually-created configurations as well. The
* default configuration sources are:
*
* - {@linkplain System#getProperties() System properties}, with an {@linkplain #getOrdinal() ordinal value} of {@code 400}
* - {@linkplain System#getenv() Environment properties}, with an ordinal value of {@code 300}
* - The {@code /META-INF/microprofile-config.properties} {@linkplain ClassLoader#getResource(String) resource},
* with an ordinal value of {@code 100}
*
*
* Environment variable name mapping rules
*
* Some operating systems allow only alphabetic characters or an underscore ({@code _}) in environment variable names.
* Other characters such as {@code .}, {@code /}, etc. may be disallowed. In order to set a value for a config property
* that has a name containing such disallowed characters from an environment variable, the following rules are used.
* Three environment variables are searched for a given property name (e.g. "{@code com.ACME.size}"):
*
* - The exact name (i.e. "{@code com.ACME.size}")
* - The name, with each character that is neither alphanumeric nor _ replaced with _ (i.e. "{@code com_ACME_size}")
* - The name, with each character that is neither alphanumeric nor _ replaced with _ and then converted to upper case
* (i.e. "{@code COM_ACME_SIZE}")
*
* The first of these environment variables that is found for a given name is returned.
*
*
Configuration source discovery
*
* Discovered configuration sources are loaded via the {@link java.util.ServiceLoader} mechanism and and can be registered by
* providing a resource named {@code META-INF/services/org.eclipse.microprofile.config.spi.ConfigSource},
* which contains the fully qualified {@code ConfigSource} implementation class name as its content.
*
*
Configuration sources may also be added by defining
* {@link org.eclipse.microprofile.config.spi.ConfigSourceProvider} classes which are discoverable in this manner.
*
*
*
Closing configuration sources
*
* If a configuration source implements the {@link AutoCloseable} interface,
* then its {@linkplain AutoCloseable#close() close method} will be called when
* the underlying configuration is released.
*
* @author Mark Struberg
* @author Gerhard Petracek
* @author Emily Jiang
* @author John D. Ament
*/
public interface ConfigSource {
/**
* The name of the configuration ordinal property, "{@code config_ordinal}".
*/
String CONFIG_ORDINAL = "config_ordinal";
/**
* The default configuration ordinal value, {@code 100}.
*/
int DEFAULT_ORDINAL = 100;
/**
* Return the properties in this configuration source as a map.
*
* @return a map containing properties of this configuration source
*/
Map getProperties();
/**
* Gets all property names known to this configuration source, without evaluating the values.
*
* For backwards compatibility, there is a default implementation that just returns the keys of {@code getProperties()}.
* Implementations should consider replacing this with a more performant implementation.
*
* The returned property names may be a subset of the names of the total set of retrievable properties in this config source.
*
* @return a set of property names that are known to this configuration source
*/
default Set getPropertyNames() {
return getProperties().keySet();
}
/**
* Return the ordinal priority value of this configuration source.
* If a property is specified in multiple config sources, the value in the config source with the highest ordinal
* takes precedence.
* For configuration sources with the same ordinal value, the configuration source name will
* be used for sorting according to string sorting criteria.
*
* Note that this method is only evaluated during the construction of the configuration, and does not affect
* the ordering of configuration sources within a configuration after that time.
*
* The ordinal values for the default configuration sources can be found above.
*
* Any configuration source which is a part of an application will typically use an ordinal between 0 and 200.
* Configuration sources provided by the container or 'environment' typically use an ordinal higher than 200.
* A framework which intends have values overridden by the application will use ordinals between 0 and 100.
*
* The default implementation of this method looks for a configuration property named "{@link #CONFIG_ORDINAL config_ordinal}"
* to determine the ordinal value for this configuration source. If the property is not found, then the
* {@linkplain #DEFAULT_ORDINAL default ordinal value} is used.
*
* This method may be overridden by configuration source implementations to provide a different behavior.
*
* @return the ordinal value
*/
default int getOrdinal() {
String configOrdinal = getValue(CONFIG_ORDINAL);
if(configOrdinal != null) {
try {
return Integer.parseInt(configOrdinal);
}
catch (NumberFormatException ignored) {
}
}
return DEFAULT_ORDINAL;
}
/**
* Return the value for the specified property in this configuration source.
*
* @param propertyName the property name
* @return the property value, or {@code null} if the property is not present
*/
String getValue(String propertyName);
/**
* The name of the configuration source. The name might be used for logging or for analysis of configured values,
* and also may be used in {@linkplain #getOrdinal() ordering decisions}.
*
* An example of a configuration source name is "{@code property-file mylocation/myprops.properties}".
*
* @return the name of the configuration source
*/
String getName();
}