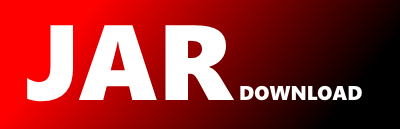
org.eclipse.packager.rpm.parse.RpmInputStream Maven / Gradle / Ivy
/**
* Copyright (c) 2015, 2019 Contributors to the Eclipse Foundation
*
* See the NOTICE file(s) distributed with this work for additional
* information regarding copyright ownership.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0
*
* SPDX-License-Identifier: EPL-2.0
*/
package org.eclipse.packager.rpm.parse;
import java.io.DataInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.ByteBuffer;
import java.nio.charset.StandardCharsets;
import java.util.Arrays;
import java.util.Optional;
import org.apache.commons.compress.archivers.cpio.CpioArchiveInputStream;
import org.eclipse.packager.rpm.RpmBaseTag;
import org.eclipse.packager.rpm.RpmLead;
import org.eclipse.packager.rpm.RpmSignatureTag;
import org.eclipse.packager.rpm.RpmTag;
import org.eclipse.packager.rpm.Rpms;
import org.eclipse.packager.rpm.coding.PayloadCoding;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.io.CountingInputStream;
public class RpmInputStream extends InputStream
{
private final static Logger logger = LoggerFactory.getLogger ( RpmInputStream.class );
private static final byte[] DUMMY = new byte[128];
private final DataInputStream in;
private boolean closed;
private RpmLead lead;
private InputHeader signatureHeader;
private InputHeader payloadHeader;
private InputStream payloadStream;
private CpioArchiveInputStream cpioStream;
private final CountingInputStream count;
public RpmInputStream ( final InputStream in )
{
this.count = new CountingInputStream ( in );
this.in = new DataInputStream ( this.count );
}
@Override
public void close () throws IOException
{
if ( !this.closed )
{
this.in.close ();
this.closed = true;
}
}
protected void ensureInit () throws IOException
{
if ( this.lead == null )
{
this.lead = readLead ();
}
if ( this.signatureHeader == null )
{
this.signatureHeader = readHeader ( true );
}
if ( this.payloadHeader == null )
{
this.payloadHeader = readHeader ( false );
}
// set up content stream
if ( this.payloadStream == null )
{
this.payloadStream = setupPayloadStream ();
this.cpioStream = new CpioArchiveInputStream ( this.payloadStream, "UTF-8" ); // we did ensure that we only support CPIO before
}
}
private InputStream setupPayloadStream () throws IOException
{
// payload format
final Object payloadFormatValue = this.payloadHeader.getTag ( RpmTag.PAYLOAD_FORMAT );
if ( payloadFormatValue != null && ! ( payloadFormatValue instanceof String ) )
{
throw new IOException ( "Payload format must be a single string" );
}
String payloadFormat = (String)payloadFormatValue;
if ( payloadFormat == null )
{
payloadFormat = "cpio";
}
if ( !"cpio".equals ( payloadFormat ) )
{
throw new IOException ( String.format ( "Unknown payload format: %s", payloadFormat ) );
}
// payload coding
final Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy