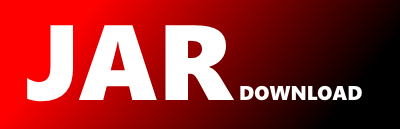
org.eclipse.swt.accessibility.AccessibleTableAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.eclipse.swt.gtk.linux.ppc64le Show documentation
Show all versions of org.eclipse.swt.gtk.linux.ppc64le Show documentation
Standard Widget Toolkit for GTK on ppc64le
The newest version!
/*******************************************************************************
* Copyright (c) 2009, 2016 IBM Corporation and others.
*
* This program and the accompanying materials
* are made available under the terms of the Eclipse Public License 2.0
* which accompanies this distribution, and is available at
* https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.swt.accessibility;
/**
* This adapter class provides default implementations for the
* methods in the AccessibleTableListener
interface.
*
* Classes that wish to deal with AccessibleTable
events can
* extend this class and override only the methods that they are
* interested in.
*
* Many methods in this adapter return cell accessible objects,
* which should implement AccessibleTableCellListener
.
*
*
* @see AccessibleTableAdapter
* @see AccessibleTableEvent
* @see AccessibleTableCellListener
* @see AccessibleTableCellEvent
*
* @since 3.6
*/
public class AccessibleTableAdapter implements AccessibleTableListener {
/**
* Deselects one column, leaving other selected columns selected (if any).
*
* @param e an event object containing the following fields:
* - [in] column - 0 based index of the column to be unselected.
* - [out] result - set to {@link ACC#OK} if the column was deselected.
*
*/
@Override
public void deselectColumn(AccessibleTableEvent e) {}
/**
* Deselects one row, leaving other selected rows selected (if any).
*
* @param e an event object containing the following fields:
* - [in] row - 0 based index of the row to be unselected
* - [out] result - set to {@link ACC#OK} if the row was deselected.
*
*/
@Override
public void deselectRow(AccessibleTableEvent e) {}
/**
* Returns the caption for the table.
*
* @param e an event object containing the following fields:
* - [out] accessible - the caption for the table, or null if the table does not have a caption
*
*
* @deprecated IAccessibleTable2::caption is deprecated, instead use the
* IA2_RELATION_LABELED_BY relation to create a relation between the table and its caption.
*/
@Deprecated
@Override
public void getCaption(AccessibleTableEvent e) {}
/**
* Returns the accessible object at the specified row and column in the table.
*
* @param e an event object containing the following fields:
* - [in] row - the 0 based row index for which to retrieve the accessible cell
* - [in] column - the 0 based column index for which to retrieve the accessible cell
* - [out] accessible - the table cell at the specified row and column index,
* or null if the row or column index are not valid
*
*/
@Override
public void getCell(AccessibleTableEvent e) {}
/**
* Returns the accessible object for the specified column in the table.
*
* @param e an event object containing the following fields:
* - [in] column - the 0 based column index for which to retrieve the accessible column
* - [out] accessible - the table column at the specified column index,
* or null if the column index is not valid
*
*/
@Override
public void getColumn(AccessibleTableEvent e) {}
/**
* Returns the total number of columns in the table.
*
* @param e an event object containing the following fields:
* - [out] count - the number of columns in the table
*
*/
@Override
public void getColumnCount(AccessibleTableEvent e) {}
/**
* Returns the description text of the specified column in the table.
*
* @param e an event object containing the following fields:
* - [in] column - the 0 based index of the column for which to retrieve the description
* - [out] result - the description text of the specified column in the table,
* or null if the column does not have a description
*
*/
@Override
public void getColumnDescription(AccessibleTableEvent e) {}
/**
* Returns the accessible object for the column header.
*
* @param e an event object containing the following fields:
* - [out] accessible - an accessible object representing the column header,
* or null if there is no column header
*
*/
@Override
public void getColumnHeader(AccessibleTableEvent e) {}
/**
* Returns the column header cells as an array of accessible objects.
*
* @param e an event object containing the following fields:
* - [out] accessibles - an array of accessible objects representing column header cells,
* or null if there are no column header cells
*
*/
@Override
public void getColumnHeaderCells(AccessibleTableEvent e) {}
/**
* Returns the columns as an array of accessible objects.
*
* @param e an event object containing the following fields:
* - [out] accessibles - an array of accessible objects representing columns,
* or null if there are no columns
*
*/
@Override
public void getColumns(AccessibleTableEvent e) {}
/**
* Returns the accessible object for the specified row in the table.
*
* @param e an event object containing the following fields:
* - [in] row - the 0 based row index for which to retrieve the accessible row
* - [out] accessible - the table row at the specified row index,
* or null if the row index is not valid
*
*/
@Override
public void getRow(AccessibleTableEvent e) {}
/**
* Returns the total number of rows in the table.
*
* @param e an event object containing the following fields:
* - [out] count - the number of rows in the table
*
*/
@Override
public void getRowCount(AccessibleTableEvent e) {}
/**
* Returns the description text of the specified row in the table.
*
* @param e an event object containing the following fields:
* - [in] row - the 0 based index of the row for which to retrieve the description
* - [out] result - the description text of the specified row in the table,
* or null if the row does not have a description
*
*/
@Override
public void getRowDescription(AccessibleTableEvent e) {}
/**
* Returns the accessible object for the row header.
*
* @param e an event object containing the following fields:
* - [out] accessible - an accessible object representing the row header,
* or null if there is no row header
*
*/
@Override
public void getRowHeader(AccessibleTableEvent e) {}
/**
* Returns the row header cells as an array of accessible objects.
*
* @param e an event object containing the following fields:
* - [out] accessibles - an array of accessible objects representing row header cells,
* or null if there are no row header cells
*
*/
@Override
public void getRowHeaderCells(AccessibleTableEvent e) {}
/**
* Returns the rows as an array of accessible objects.
*
* @param e an event object containing the following fields:
* - [out] accessibles - an array of accessible objects representing rows,
* or null if there are no rows
*
*/
@Override
public void getRows(AccessibleTableEvent e) {}
/**
* Returns the number of selected cells.
*
* @param e an event object containing the following fields:
* - [out] count - the number of cells currently selected
*
*/
@Override
public void getSelectedCellCount(AccessibleTableEvent e) {}
/**
* Returns the currently selected cells.
*
* @param e an event object containing the following fields:
* - [out] accessibles - array containing the selected accessible cells
*
*/
@Override
public void getSelectedCells(AccessibleTableEvent e) {}
/**
* Returns the number of selected columns.
*
* @param e an event object containing the following fields:
* - [out] count - the number of columns currently selected
*
*/
@Override
public void getSelectedColumnCount(AccessibleTableEvent e) {}
/**
* Returns the column indexes that are currently selected.
*
* @param e an event object containing the following fields:
* - [out] selected - an array of 0 based column indexes of selected columns
*
*/
@Override
public void getSelectedColumns(AccessibleTableEvent e) {}
/**
* Returns the number of selected rows.
*
* @param e an event object containing the following fields:
* - [out] count - the number of rows currently selected
*
*/
@Override
public void getSelectedRowCount(AccessibleTableEvent e) {}
/**
* Returns the row indexes that are currently selected.
*
* @param e an event object containing the following fields:
* - [out] selected - an array of 0 based row indexes of selected rows
*
*/
@Override
public void getSelectedRows(AccessibleTableEvent e) {}
/**
* Returns the summary description of the table.
*
* @param e an event object containing the following fields:
* - [out] accessible - the summary for the table,
* or null if the table does not have a summary
*
*
* @deprecated IAccessibleTable2::summary is deprecated, instead use the
* IA2_RELATION_DESCRIBED_BY relation to create a relation between the table and its summary.
*/
@Deprecated
@Override
public void getSummary(AccessibleTableEvent e) {}
/**
* Returns the visible columns as an array of accessible objects.
*
* @param e an event object containing the following fields:
* - [out] accessibles - an array of accessible objects representing visible columns,
* or null if there are no visible columns
*
*/
@Override
public void getVisibleColumns(AccessibleTableEvent e) {}
/**
* Returns the visible rows as an array of accessible objects.
*
* @param e an event object containing the following fields:
* - [out] accessibles - an array of accessible objects representing visible rows,
* or null if there are no visible rows
*
*/
@Override
public void getVisibleRows(AccessibleTableEvent e) {}
/**
* Returns a boolean value indicating whether the specified column is
* completely selected.
*
* @param e an event object containing the following fields:
* - [in] column - 0 based index of the column for which to determine whether it is selected
* - [out] isSelected - true if the specified column is selected completely, and false otherwise
*
*/
@Override
public void isColumnSelected(AccessibleTableEvent e) {}
/**
* Returns a boolean value indicating whether the specified row is
* completely selected.
*
* @param e an event object containing the following fields:
* - [in] row - 0 based index of the row for which to determine whether it is selected
* - [out] isSelected - true if the specified row is selected completely, and false otherwise
*
*/
@Override
public void isRowSelected(AccessibleTableEvent e) {}
/**
* Selects a column.
*
* @param e an event object containing the following fields:
* - [in] column - 0 based index of the column to be selected
* - [out] result - set to {@link ACC#OK} if the column was selected.
*
*/
@Override
public void selectColumn(AccessibleTableEvent e) {}
/**
* Selects a row.
*
* @param e an event object containing the following fields:
* - [in] row - 0 based index of the row to be selected
* - [out] result - set to {@link ACC#OK} if the row was selected.
*
*/
@Override
public void selectRow(AccessibleTableEvent e) {}
/**
* Selects a column and deselects all previously selected columns.
*
* @param e an event object containing the following fields:
* - [in] column - 0 based index of the column to be selected
* - [out] result - set to {@link ACC#OK} if the column was selected.
*
*/
@Override
public void setSelectedColumn(AccessibleTableEvent e) {}
/**
* Selects a row and deselects all previously selected rows.
*
* @param e an event object containing the following fields:
* - [in] row - 0 based index of the row to be selected
* - [out] result - set to {@link ACC#OK} if the row was selected.
*
*/
@Override
public void setSelectedRow(AccessibleTableEvent e) {}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy