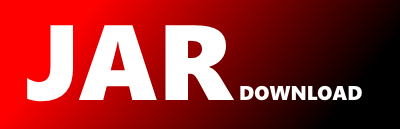
org.eclipse.swt.accessibility.AccessibleTableCellAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.eclipse.swt.gtk.linux.ppc64le Show documentation
Show all versions of org.eclipse.swt.gtk.linux.ppc64le Show documentation
Standard Widget Toolkit for GTK on ppc64le
The newest version!
/*******************************************************************************
* Copyright (c) 2009, 2016 IBM Corporation and others.
*
* This program and the accompanying materials
* are made available under the terms of the Eclipse Public License 2.0
* which accompanies this distribution, and is available at
* https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.swt.accessibility;
/**
* This adapter class provides default implementations for the
* methods in the AccessibleTableCellListener
interface.
*
* Classes that wish to deal with AccessibleTableCell
events can
* extend this class and override only the methods that they are
* interested in.
*
*
* @see AccessibleTableCellListener
* @see AccessibleTableCellEvent
*
* @since 3.6
*/
public class AccessibleTableCellAdapter implements AccessibleTableCellListener {
/**
* Returns the number of columns occupied by this cell accessible.
*
* This is 1 if the specified cell is only in one column, or
* more than 1 if the specified cell spans multiple columns.
*
*
* @param e an event object containing the following fields:
* - [out] count - the 1 based number of columns spanned by the specified cell
*
*/
@Override
public void getColumnSpan(AccessibleTableCellEvent e) {}
/**
* Returns the column headers as an array of cell accessibles.
* TODO: doc that this is a more efficient way to get headers of a cell than TableListener.getRow/ColHeaders
*
* @param e an event object containing the following fields:
* - [out] accessibles - an array of cell accessibles, or null if there are no column headers
*
*/
@Override
public void getColumnHeaders(AccessibleTableCellEvent e) {}
/**
* Translates this cell accessible into the corresponding column index.
*
* @param e an event object containing the following fields:
* - [out] index - the 0 based column index of the specified cell,
* or the index of the first column if the cell spans multiple columns
*
*/
@Override
public void getColumnIndex(AccessibleTableCellEvent e) {}
/**
* Returns the number of rows occupied by this cell accessible.
*
* This is 1 if the specified cell is only in one row, or
* more than 1 if the specified cell spans multiple rows.
*
*
* @param e an event object containing the following fields:
* - [out] count - the 1 based number of rows spanned by the specified cell
*
*/
@Override
public void getRowSpan(AccessibleTableCellEvent e) {}
/**
* Returns the row headers as an array of cell accessibles.
* TODO: doc that this is a more efficient way to get headers of a cell than TableListener.getRow/ColHeaders
*
* @param e an event object containing the following fields:
* - [out] accessibles - an array of cell accessibles, or null if there are no row headers
*
*/
@Override
public void getRowHeaders(AccessibleTableCellEvent e) {}
/**
* Translates this cell accessible into the corresponding row index.
*
* @param e an event object containing the following fields:
* - [out] index - the 0 based row index of the specified cell,
* or the index of the first row if the cell spans multiple rows
*
*/
@Override
public void getRowIndex(AccessibleTableCellEvent e) {}
/**
* Returns the accessible for the table containing this cell.
*
* @param e an event object containing the following fields:
* - [out] accessible - the accessible for the containing table
*
*/
@Override
public void getTable(AccessibleTableCellEvent e) {}
/**
* Returns a boolean value indicating whether this cell is selected.
*
* @param e an event object containing the following fields:
* - [out] isSelected - true if the specified cell is selected and false otherwise
*
*/
@Override
public void isSelected(AccessibleTableCellEvent e) {}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy