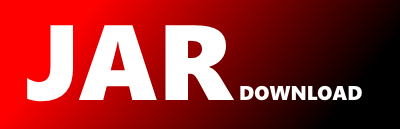
org.eclipse.swt.internal.BidiUtil Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2000, 2022 IBM Corporation and others.
*
* This program and the accompanying materials
* are made available under the terms of the Eclipse Public License 2.0
* which accompanies this distribution, and is available at
* https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.swt.internal;
import java.util.*;
import org.eclipse.swt.*;
import org.eclipse.swt.graphics.*;
import org.eclipse.swt.internal.win32.*;
import org.eclipse.swt.widgets.*;
/*
* Wraps Win32 API used to bidi enable widgets. Up to 3.104 was used by
* StyledText widget exclusively. 3.105 release introduced the method
* #resolveTextDirection, which is used by other widgets as well.
*/
public class BidiUtil {
// Keyboard language ids
public static final int KEYBOARD_NON_BIDI = 0;
public static final int KEYBOARD_BIDI = 1;
// bidi flag
static int isBidiPlatform = -1;
// getRenderInfo flag values
public static final int CLASSIN = 1;
public static final int LINKBEFORE = 2;
public static final int LINKAFTER = 4;
// variables used for providing a listener mechanism for keyboard language
// switching
static Map languageMap = new HashMap<> ();
static Map oldProcMap = new HashMap<> ();
static Callback callback = new Callback (BidiUtil.class, "windowProc", 4); //$NON-NLS-1$
// GetCharacterPlacement constants
static final int GCP_REORDER = 0x0002;
static final int GCP_GLYPHSHAPE = 0x0010;
static final int GCP_LIGATE = 0x0020;
static final int GCP_CLASSIN = 0x00080000;
static final byte GCPCLASS_ARABIC = 2;
static final byte GCPCLASS_HEBREW = 2;
static final byte GCPCLASS_LOCALNUMBER = 4;
static final byte GCPCLASS_LATINNUMBER = 5;
static final int GCPGLYPH_LINKBEFORE = 0x8000;
static final int GCPGLYPH_LINKAFTER = 0x4000;
// ExtTextOut constants
static final int ETO_CLIPPED = 0x4;
static final int ETO_GLYPH_INDEX = 0x0010;
// Windows primary language identifiers
static final int LANG_ARABIC = 0x01;
static final int LANG_HEBREW = 0x0d;
static final int LANG_FARSI = 0x29;
// ActivateKeyboard constants
static final int HKL_NEXT = 1;
static final int HKL_PREV = 0;
/*
* Public character class constants are the same as Windows
* platform constants.
* Saves conversion of class array in getRenderInfo to arbitrary
* constants for now.
*/
public static final int CLASS_HEBREW = GCPCLASS_ARABIC;
public static final int CLASS_ARABIC = GCPCLASS_HEBREW;
public static final int CLASS_LOCALNUMBER = GCPCLASS_LOCALNUMBER;
public static final int CLASS_LATINNUMBER = GCPCLASS_LATINNUMBER;
public static final int REORDER = GCP_REORDER;
public static final int LIGATE = GCP_LIGATE;
public static final int GLYPHSHAPE = GCP_GLYPHSHAPE;
/**
* Adds a language listener. The listener will get notified when the language of
* the keyboard changes (via Alt-Shift on Win platforms). Do this by creating a
* window proc for the Control so that the window messages for the Control can be
* monitored.
*
* @param hwnd the handle of the Control that is listening for keyboard language
* changes
* @param runnable the code that should be executed when a keyboard language change
* occurs
*/
public static void addLanguageListener (long hwnd, Runnable runnable) {
languageMap.put(new LONG(hwnd), runnable);
subclass(hwnd);
}
public static void addLanguageListener (Control control, Runnable runnable) {
addLanguageListener(control.handle, runnable);
}
/**
* Proc used for OS.EnumSystemLanguageGroups call during isBidiPlatform test.
*/
static long EnumSystemLanguageGroupsProc(long lpLangGrpId, long lpLangGrpIdString, long lpLangGrpName, long options, long lParam) {
if ((int)lpLangGrpId == OS.LGRPID_HEBREW) {
isBidiPlatform = 1;
return 0;
}
if ((int)lpLangGrpId == OS.LGRPID_ARABIC) {
isBidiPlatform = 1;
return 0;
}
return 1;
}
/**
* Wraps the ExtTextOut function.
*
* @param gc the gc to use for rendering
* @param renderBuffer the glyphs to render as an array of characters
* @param renderDx the width of each glyph in renderBuffer
* @param x x position to start rendering
* @param y y position to start rendering
*/
public static void drawGlyphs(GC gc, char[] renderBuffer, int[] renderDx, int x, int y) {
int length = renderDx.length;
if (OS.GetLayout (gc.handle) != 0) {
reverse(renderDx);
renderDx[length-1]--; //fixes bug 40006
reverse(renderBuffer);
}
// render transparently to avoid overlapping segments. fixes bug 40006
int oldBkMode = OS.SetBkMode(gc.handle, OS.TRANSPARENT);
OS.ExtTextOut(gc.handle, x, y, ETO_GLYPH_INDEX , null, renderBuffer, renderBuffer.length, renderDx);
OS.SetBkMode(gc.handle, oldBkMode);
}
/**
* Return ordering and rendering information for the given text. Wraps the GetFontLanguageInfo
* and GetCharacterPlacement functions.
*
* @param gc the GC to use for measuring of this line, input parameter
* @param text text that bidi data should be calculated for, input parameter
* @param order an array of integers representing the visual position of each character in
* the text array, output parameter
* @param classBuffer an array of integers representing the type (e.g., ARABIC, HEBREW,
* LOCALNUMBER) of each character in the text array, input/output parameter
* @param dx an array of integers representing the pixel width of each glyph in the returned
* glyph buffer, output parameter
* @param flags an integer representing rendering flag information, input parameter
* @param offsets text segments that should be measured and reordered separately, input
* parameter. See org.eclipse.swt.custom.BidiSegmentEvent for details.
* @return buffer with the glyphs that should be rendered for the given text
*/
public static char[] getRenderInfo(GC gc, String text, int[] order, byte[] classBuffer, int[] dx, int flags, int [] offsets) {
int fontLanguageInfo = OS.GetFontLanguageInfo(gc.handle);
long hHeap = OS.GetProcessHeap();
boolean isRightOriented = OS.GetLayout(gc.handle) != 0;
char [] textBuffer = text.toCharArray();
int byteCount = textBuffer.length;
boolean linkBefore = (flags & LINKBEFORE) == LINKBEFORE;
boolean linkAfter = (flags & LINKAFTER) == LINKAFTER;
GCP_RESULTS result = new GCP_RESULTS();
result.lStructSize = GCP_RESULTS.sizeof;
result.nGlyphs = byteCount;
long lpOrder = result.lpOrder = OS.HeapAlloc(hHeap, OS.HEAP_ZERO_MEMORY, byteCount * 4);
long lpDx = result.lpDx = OS.HeapAlloc(hHeap, OS.HEAP_ZERO_MEMORY, byteCount * 4);
long lpClass = result.lpClass = OS.HeapAlloc(hHeap, OS.HEAP_ZERO_MEMORY, byteCount);
long lpGlyphs = result.lpGlyphs = OS.HeapAlloc(hHeap, OS.HEAP_ZERO_MEMORY, byteCount * 2);
// set required dwFlags
int dwFlags = 0;
int glyphFlags = 0;
// Always reorder. We assume that if we are calling this function we're
// on a platform that supports bidi. Fixes 20690.
dwFlags |= GCP_REORDER;
if ((fontLanguageInfo & GCP_LIGATE) == GCP_LIGATE) {
dwFlags |= GCP_LIGATE;
glyphFlags |= 0;
}
if ((fontLanguageInfo & GCP_GLYPHSHAPE) == GCP_GLYPHSHAPE) {
dwFlags |= GCP_GLYPHSHAPE;
if (linkBefore) {
glyphFlags |= GCPGLYPH_LINKBEFORE;
}
if (linkAfter) {
glyphFlags |= GCPGLYPH_LINKAFTER;
}
}
byte[] lpGlyphs2;
if (linkBefore || linkAfter) {
lpGlyphs2 = new byte[2];
lpGlyphs2[0]=(byte)glyphFlags;
lpGlyphs2[1]=(byte)(glyphFlags >> 8);
}
else {
lpGlyphs2 = new byte[] {(byte) glyphFlags};
}
OS.MoveMemory(result.lpGlyphs, lpGlyphs2, lpGlyphs2.length);
if ((flags & CLASSIN) == CLASSIN) {
// set classification values for the substring
dwFlags |= GCP_CLASSIN;
OS.MoveMemory(result.lpClass, classBuffer, classBuffer.length);
}
char[] glyphBuffer = new char[result.nGlyphs];
int glyphCount = 0;
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy