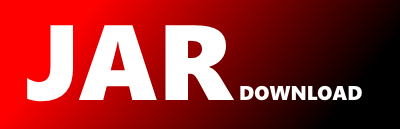
org.eclipse.rdf4j.http.server.ProtocolExceptionResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rdf4j-http-server-spring Show documentation
Show all versions of rdf4j-http-server-spring Show documentation
HTTP server implementing a REST-style protocol
The newest version!
/*******************************************************************************
* Copyright (c) 2015 Eclipse RDF4J contributors, Aduna, and others.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Distribution License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*
* SPDX-License-Identifier: BSD-3-Clause
*******************************************************************************/
package org.eclipse.rdf4j.http.server;
import java.io.StringWriter;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.eclipse.rdf4j.common.exception.ValidationException;
import org.eclipse.rdf4j.common.webapp.views.SimpleResponseView;
import org.eclipse.rdf4j.model.Model;
import org.eclipse.rdf4j.rio.RDFFormat;
import org.eclipse.rdf4j.rio.Rio;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.servlet.HandlerExceptionResolver;
import org.springframework.web.servlet.ModelAndView;
/**
* Simple resolver for Exceptions: returns the correct response code and message to the client.
*
* @author Herko ter Horst
*/
public class ProtocolExceptionResolver implements HandlerExceptionResolver {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
@Override
public ModelAndView resolveException(HttpServletRequest request, HttpServletResponse response, Object handler,
Exception exception) {
logger.debug("ProtocolExceptionResolver.resolveException() called");
Map model = new HashMap<>();
int statusCode = HttpServletResponse.SC_INTERNAL_SERVER_ERROR;
String errMsg = exception.getMessage();
if (exception instanceof HTTPException) {
HTTPException httpExc = (HTTPException) exception;
statusCode = httpExc.getStatusCode();
if (exception instanceof ClientHTTPException) {
logger.info("Client sent bad request ( " + statusCode + ")", exception);
} else {
logger.error("Error while handling request (" + statusCode + ")", exception);
}
} else {
logger.error("Error while handling request", exception);
}
int depth = 10;
Throwable temp = exception;
while (!(temp instanceof ValidationException)) {
if (depth-- == 0) {
break;
}
if (temp == null) {
break;
}
temp = temp.getCause();
}
if (temp instanceof ValidationException) {
// This is currently just a simple fix that causes the validation report to be printed.
// This should not be the final solution.
Model validationReportModel = ((ValidationException) temp).validationReportAsModel();
StringWriter stringWriter = new StringWriter();
// We choose RDFJSON because this format doesn't rename blank nodes.
Rio.write(validationReportModel, stringWriter, RDFFormat.RDFJSON);
statusCode = HttpServletResponse.SC_CONFLICT;
errMsg = stringWriter.toString();
Map headers = new HashMap<>();
headers.put("Content-Type", "application/shacl-validation-report+rdf+json");
model.put(SimpleResponseView.CUSTOM_HEADERS_KEY, headers);
}
model.put(SimpleResponseView.SC_KEY, statusCode);
model.put(SimpleResponseView.CONTENT_KEY, errMsg);
return new ModelAndView(SimpleResponseView.getInstance(), model);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy